in C++ // praphrase or change this code , while the purpose and output is same
in C++ // praphrase or change this code , while the purpose and output is same .
#include <iostream>
#include <stdint.h>
using namespace std;
class arr{
private:
int size_arr; //To store the size of the array
char *begin; //Pointer for dynamic allocation of memory
int capacity; //To store the capacity of the elements in the array
public:
arr(int n); //constructor (default) for creating an array
int insert_arr(char x); //To insert an element x in the array
int remov_arr(int i); //To remove an element from position i
void display();
void displayAtPos(int i);//Function to display a character at position i
};
arr::arr(int n){
size_arr = n;
begin = new char[size_arr];
capacity = 0;
}
int arr::insert_arr(const char x){
if(capacity >= size_arr){
cout << "Array Full!" << endl;
return -1;
}
begin[capacity++] = x; //One element is added, so increment the capacity by one.
return 0;
}
int arr::remov_arr(int i){
if(capacity == 0){
cout << "Nothing to delete " << endl;
return -1;
}
if(capacity < i || i < 0){
cout << "Out of bound" << endl;
return -1;
}
if(capacity == 1){
begin[capacity] = '\0';
capacity--;
return 0;
}
for(int j = i;j < capacity;j++){
begin[j] = begin[j+1]; //Shift each element towards one place before
}
begin[capacity] = '\0'; //change the duplicate character in the end to a null character.
capacity--; //One element is removed, so decrement the capacity by one.
return 0;
}
void arr::display(void){
for(int i = 0;i < capacity;i++){
cout << begin[i];
}
cout << endl;
}
void arr::displayAtPos(int i){
if(begin[i] == '\0')
cout << "Null character\n";
else{
cout << begin[i] << endl;
}
}
int main()
{
int n;
arr obj1(26);
obj1.insert_arr('A');
obj1.insert_arr('B');
obj1.insert_arr('C');
obj1.insert_arr('D');
obj1.insert_arr('E');
obj1.insert_arr('F');
obj1.insert_arr('G');
obj1.insert_arr('H');
obj1.insert_arr('I');
obj1.insert_arr('J');
obj1.insert_arr('K');
obj1.insert_arr('L');
obj1.insert_arr('M');
obj1.insert_arr('N');
obj1.insert_arr('O');
obj1.insert_arr('P');
obj1.insert_arr('Q');
obj1.insert_arr('R');
obj1.insert_arr('S');
obj1.insert_arr('T');
obj1.insert_arr('U');
obj1.insert_arr('V');
obj1.insert_arr('W');
obj1.insert_arr('X');
obj1.insert_arr('Y');
obj1.insert_arr('Z');
obj1.display();
cout << "Enter element number to be erased(0 to 25) :";
cin >> n;
obj1.remov_arr(n);
cout << "After Removing item at position " << n << " :" << endl;
obj1.display();
cout << "Data at last element is :";
obj1.displayAtPos(25);
return 0;
}

Step by step
Solved in 2 steps with 1 images

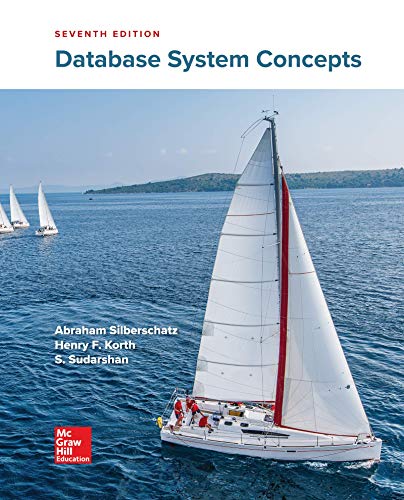
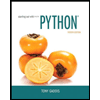
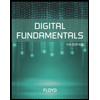
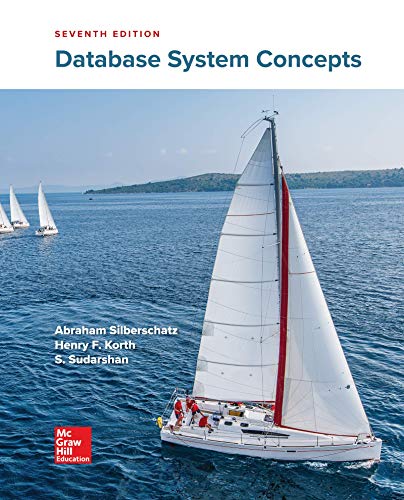
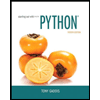
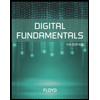
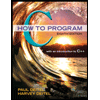
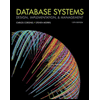
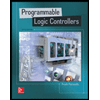