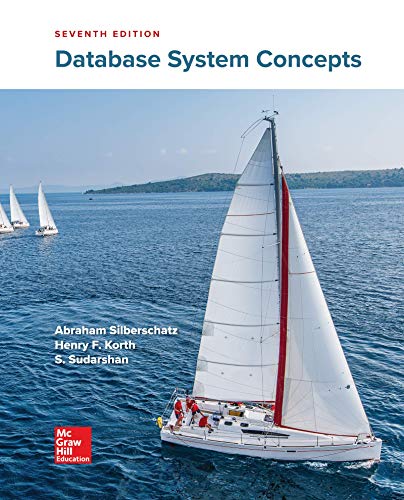
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Design a Client class that interfaces with your SQLDatabase Server (from the class Server). The Client sends a SQLQuery to the SQLDatabase and receives a Dataframe from the SQLDatabase. Your class should contain the default __dunders__ and potentially introduce some custom __dunders__.
classServer.py (Just change if this file has something doesn't run or doesn't fit with new code)
import sqlite3
import pandas as pd
class Server:
def __init__(self, db_name):
self.db = sqlite3.connect(db_name)
self.cursor = self.db.cursor()
def __enter__(self):
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.db.close()
def create_table(self, table_schema):
self.cursor.execute(table_schema)
self.db.commit()
def insert_data(self, table_name, data):
for i in range(len(data)):
keys = ", ".join(data.columns)
values = ", ".join([f"'{value}'" if pd.isna(value) else str(value) for value in data.iloc[i]])
self.cursor.execute(f"INSERT INTO {table_name} ({keys}) VALUES ({values})")
self.db.commit()
def search_data(self, table_name, year):
self.cursor.execute(f"SELECT * FROM {table_name} WHERE year={year}")
results = self.cursor.fetchall()
if results:
# Convert the fetched data into a DataFrame
df = pd.DataFrame(results, columns=[desc[0] for desc in self.cursor.description])
return df
else:
return None
def delete_data(self, table_name, year):
self.cursor.execute(f"DELETE FROM {table_name} WHERE year={year}")
self.db.commit()
return self.cursor.rowcount > 0
import pandas as pd
class Server:
def __init__(self, db_name):
self.db = sqlite3.connect(db_name)
self.cursor = self.db.cursor()
def __enter__(self):
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.db.close()
def create_table(self, table_schema):
self.cursor.execute(table_schema)
self.db.commit()
def insert_data(self, table_name, data):
for i in range(len(data)):
keys = ", ".join(data.columns)
values = ", ".join([f"'{value}'" if pd.isna(value) else str(value) for value in data.iloc[i]])
self.cursor.execute(f"INSERT INTO {table_name} ({keys}) VALUES ({values})")
self.db.commit()
def search_data(self, table_name, year):
self.cursor.execute(f"SELECT * FROM {table_name} WHERE year={year}")
results = self.cursor.fetchall()
if results:
# Convert the fetched data into a DataFrame
df = pd.DataFrame(results, columns=[desc[0] for desc in self.cursor.description])
return df
else:
return None
def delete_data(self, table_name, year):
self.cursor.execute(f"DELETE FROM {table_name} WHERE year={year}")
self.db.commit()
return self.cursor.rowcount > 0
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- in python. For this project, you will import the json module. Write a class named NobelData that reads a JSON file containing data on Nobel Prizes and allows the user to search that data. Your code does not need to access the internet. Your class should have: an init method that reads the file and stores it in whatever data member(s) you prefer. Any data members of the NobelData class must be private. a method named search_nobel that takes as parameters a year and a category, and returns a sorted list (in normal English dictionary order) of the surnames for the winner(s) in that category for that year (up to three people can share the prize). The year will be a string (e.g. "1975"), not a number. The categories are: "chemistry", "economics", "literature", "peace", "physics", and "medicine". For example, your class could be used like this: nd = NobelData() nd.search_nobel("2001", "economics")arrow_forwardjava program For this question, the server contains the id, name and cgpa of some Students in a file. The client will request the server for specific data, which the server will provide to the client. Server: The server contains the data of some Students. For each student, the server contains id, name and cgpa data in a file. Here's an example of the file: data.txt101 Saif 3.52201 Hasan 3.81.... Create a similar file in you server side. The file should have at least 8 students. The client will request the server to provide the details (id, name, cgpa) of the Nth highest cgpa student in the file. If N's value is 1, the server will return the details of the student with the highest cgpa. if N's value is 3, the server will return the details of the student with the third highest cgpa. If N's value is incorrect, the server returns to the client: "Invalid request". Client: The client sends the server the value of N, which is an integer number. The client takes input the value of N…arrow_forwardCreate an anonymous block that a teacher can run to insert the student's grade on a particular assignment. Accept a NUMERIC_GRADE, CLASS_ASSESSMENT_ID, CLASS_ID and STU_ID. Use “today‟s” date for the DATE_TURNED_IN.arrow_forward
- I'm not sure how to fix my error import csv #This is the classclass ConvertCSVToJSON: def __init__(self, headings, ID, linesFromFile, inputFileName, outputFileName="Project7Output.txt"): self.headings=headings # Headings (row 1 of the file) self.ID=ID self.linesFromFile=linesFromFile # rows2-end of file (EOF) self.outputFileName=outputFileName self.inputFileName=inputFileName def createKeyValuePair(self, key, value): # Think about parameters and how the code will work result="\""+key+"\""+":"+value return result #create a line function/method in class to have access to headings and file. def createLine(self, ID, line): #splits the line into different components lineComponets=line.split(",") dictionary={}#creats a dictionary for the componets for i in range(len(self.heading)): dictionary[self.headings[i]]=lineComponents[i] #adds ID as a keyvalue pair dictionary["ID"]=str(ID) #should convert to json…arrow_forwardInfoFrame Data Storage Process Data File A0 7 B0 0 C0 18 D0 11 E0 16 F0 10 G0 11 H0 8 I0 13 J0 11 A10 19 B10 9 C10 3 D10 4 E10 7 ... F10 3 G10 19 H10 18 I10 15 J10 13 Testing Create a main method in your script. Utilize the data file provided to create the InfoFrame object. Write the code shown below in the main method EXACTLY as shown. Call the main method Substitute your filepath herearrow_forwardjava programming please type the code For this problem set, you will submit a single java file named Homework.java. You are supposed to add the functions described below to your Homework class. The functional signatures of the functions you create must exactly match the signatures in the problem titles. You are not allowed to use any 3rd party libraries in the homework assignment nor are you allowed to use any dependencies from the java.util package besides the Pattern Class. When you hava completed your homework, upload the Homework.java file to Grader Than. All tests will timeout and fail if your code takes longer than 10 seconds to complete. PrivateFunction() This is a private non-static function that takes no arguments and returns an integer. You don't need to put any code in this function, you may leave the function's code empty. Don't overthink this problem. This is to test your knowledge on how to create a non-static private function. Arguments: returns int - You may…arrow_forward
- Ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN. I am having trouble with a specific line of my code here is my code import java.util.Comparator; import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Collections; import java.util.Scanner; class Car { String manufacturer; String model; int year; String vin; public Car(String manufacturer, String model, int year, String vin) { super(); this.manufacturer = manufacturer; this.model = model; this.year = year; this.vin = vin; } public String getManufacturer() { return manufacturer; } public void setManufacturer(String manufacturer) { this.manufacturer = manufacturer; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } public String getVin() { return vin;…arrow_forwardAs the first step in writing an application for the local book store, we will design and implement a Book class that will define the data structure of the application. Each book has a name, author, type (paperback, hardback, magazine), integer ID and pageCount. Provide some data validation in the appropriate method to ensure that the ID and pageCount are not negative. Design and implement a Book class with separation, i.e., separate all functions and methods into both the prototype and an implementation below the main. Provide constructor methods for Book(void), Book(name, author), and one for all components Book(name, author, type, ID, pageCount) Provide accessor (get and set) methods for each property as well as a display method. Provide data validation for the ID and pageCount in the appropriate methods. Provide a method to calculate the cost for buying books (with a 7.75% sales tax) according to the following chart: Hardcover: $29.95 per book Paperback:…arrow_forwardMake any necessary modifications to the RushJob class so that it can be sorted by job number. Modify the JobDemo3 application so the displayed orders have been sorted. Save the application as JobDemo4. An example of the program is shown below: Enter job number 22 Enter customer name Joe Enter description Powerwashing Enter estimated hours 4 Enter job number 6 Enter customer name Joey Enter description Painting Enter estimated hours 8 Enter job number 12 Enter customer name Joseph Enter description Carpet cleaning Enter estimated hours 5 Enter job number 9 Enter customer name Josefine Enter description Moving Enter estimated hours 12 Enter job number 21 Enter customer name Josefina Enter description Dog walking Enter estimated hours 2 Summary: RushJob 6 Joey Painting 8 hours @$45.00 per hour. Rush job adds 150 premium. Total price is $510.00 RushJob 9 Josefine Moving 12 hours @$45.00 per hour. Rush job adds 150 premium. Total price is $690.00 RushJob 12 Joseph Carpet cleaning 5 hours…arrow_forward
- Part 2: Sorting the WorkOrders via dates Another error that will still be showing is that there is not Comparable/compareTo() method setup on the WorkOrder class file. That is something you need to fix and code. Implement the use of the Comparable interface and add the compareTo() method to the WorkOrder class. The compareTo() method will take a little work here. We are going to compare via the date of the work order. The dates of the WorkOrder are saved in a MM-DD-YYYY format. There is a dash '-' in between each part of the date. You will need to split both the current object's date and the date sent through the compareTo() parameters. You will have three things to compare against. You first need to check the year. If the years are the same value then you need to go another step to check the months, otherwise you compare them with less than or greater than and return the corresponding value. If you have to check the months it would be the same for years. If the months are the same you…arrow_forwardYour company is doing some data cleanup, and notices that the email list of all users has been getting outdated. For one, there are some users with repeat email addresses, and some of the email accounts no longer exist. Your job is to create a series of methods that can purge some of the old data from the existing email list. Create static methods in the DataPurge class that can do the following: removeDuplicates This method takes an email list, and removes the duplicate email values. It also prints to the console which duplicate emails have been removed. removeAOL This method removes all email addresses from a list that are from aol.com. It notifies the user which email addresses are being removed as well. containsOnlyEmails This method returns true if all of the data in the email list is actually an email address. We will define something as an email address if it contains the characters @ and . Test your methods out in the DataPurgeTester file. You don’t have to change anything…arrow_forwardThe contact service shall be able to add contacts with a unique ID. The contact service shall be able to delete contacts per contact ID. The contact service shall be able to update contact fields per contact ID. The following fields are updatable: firstName lastName Number Address need help with this class in Javaarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
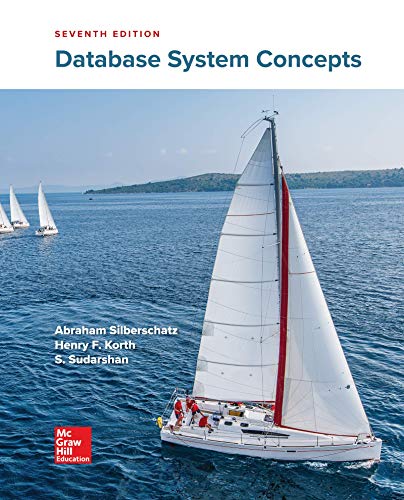
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
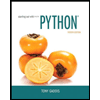
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
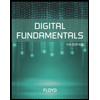
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
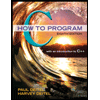
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
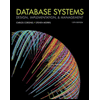
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
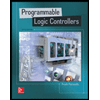
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education