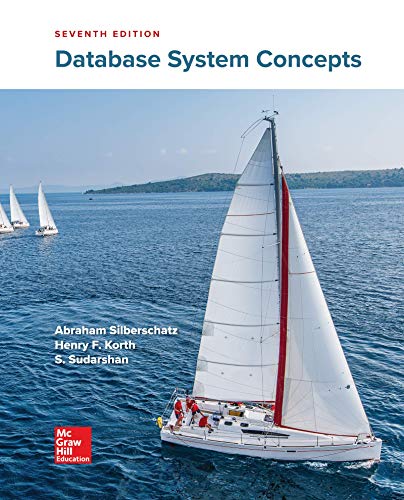
Ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN.
I am having trouble with a specific line of my code
here is my code
import java.util.Comparator;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Scanner;
class Car {
String manufacturer;
String model;
int year;
String vin;
public Car(String manufacturer, String model, int year, String vin) {
super();
this.manufacturer = manufacturer;
this.model = model;
this.year = year;
this.vin = vin;
}
public String getManufacturer() {
return manufacturer;
}
public void setManufacturer(String manufacturer) {
this.manufacturer = manufacturer;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public String getVin() {
return vin;
}
public void setVin(String vin) {
this.vin = vin;
}
}
class CarComparator implements Comparator<Car> {
@Override
public int compare(Car car1, Car car2) {
int yearCompare = Integer.compare(car1.getYear(), car2.getYear());
if (yearCompare != 0) {
return yearCompare;
}
return car1.getVin().compareTo(car2.getVin());
}
}
public class OldestCarsByMake {
public static void main(String[] args) throws FileNotFoundException{
ArrayList<Car> cars = new ArrayList<Car>();
Scanner sc1 = new Scanner(System.in);
System.out.println("Enter filename");
String carFile= sc1.next();
File file = new File(carFile);
Scanner sc2 = new Scanner(file);
String[] carDetails;
sc2.nextLine();
while(sc2.hasNext()){
String carLine = sc2.nextLine();
carDetails=carLine.split("\\s+");
String manufacturer=carDetails[0];
String model = carDetails[1];
int year = Integer.parseInt(carDetails[2]);
String vin = carDetails[3];
boolean flag=true;
for(Car c : cars){
if(c.manufacturer.equals(manufacturer)){
flag=false;
if(c.year>year){
c.setModel(model);
c.setYear(year);
c.setVin(vin);
}
else if(c.year==year){
if((c.vin).compareTo(vin)>0){
c.setModel(model);
c.setYear(year);
c.setVin(vin);
}
}
}
}
if(flag)
cars.add(new Car(manufacturer,model,year,vin));
}
Collections.sort(cars, new CarComparator());
int spaces=25;
for(Car c: cars){
for(int i=0;i<20-(c.manufacturer.length());i++)
System.out.print(" ");
System.out.print(c.manufacturer);
for(int i=0;i<20-(c.model.length());i++)
System.out.print(" ");
System.out.println(c.model+" "+c.year+" "+c.vin);
}
System.out.println(cars.size()+" result(s)");
}
}
however this line of my code produces an error
String model = carDetails[1];
i.e.
Enter filename\n
car-list-3.txtENTER
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 1 out of bounds for length 1\n
\tat OldestCarsByMake.main(OldestCarsByMake.java:68)\n
Can an expert help fix this error to produce the correct outcome

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 3 images

- Written in Python with docstring please if applicable Thank youarrow_forwardYou have to use comment function to describe what each line does import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class PreferenceData { private final List<Student> students; private final List<Project> projects; private int[][] preferences; private static enum ReadState { STUDENT_MODE, PROJECT_MODE, PREFERENCE_MODE, UNKNOWN; }; public PreferenceData() { super(); this.students = new ArrayList<Student>(); this.projects = new ArrayList<Project>(); } public void addStudent(Student s) { this.students.add(s); } public void addStudent(String s) { this.addStudent(Student.createStudent(s)); } public void addProject(Project p) { this.projects.add(p); } public void addProject(String p) { this.addProject(Project.createProject(p)); } public void createPreferenceMatrix() { this.preferences = new…arrow_forwardCreate an instance of the MovieDetails class called new_movie. Then, read three values from input into new_movie's attributes in the following order: year (int) duration (int) title (str)arrow_forward
- JAVA PROGRAM (Create large dataset) Create a data file with 500 lines. Each line in the file consists of a faculty member’s first name, last name, rank, and salary. The faculty member’s first name and last name for the ith line are FirstNamei and LastNamei. The rank is randomly generated as assistant, associate, and full. The salary is randomly generated as a number with two digits after the decimal point. The salary for an assistant professor should be in the range from 50,000 to 80,000, for associate professor from 60,000 to 110,000, and for full professor from 75,000 to 130,000. Save the file in Salary.txt. Here are some sample data: FirstName1 LastName1 assistant 60055.95 FirstName2 LastName2 associate 81112.45 . . . FirstName500 LastName500 full 92255.21arrow_forwardCreate a new C++ project in Visual Studio (or an IDE of your choice). Add the files listed below to your project: BinaryTree.h Download BinaryTree.h BinarySearchTree.h Download BinarySearchTree.h main.cpp Download main.cpp Build and run your project. The program should compile without any errors. Note that function main() creates a new BST of int and inserts the nodes 12, 38, 25, 5, 15, 8, 55 (in that order) and displays them using pre-order traversal. Verify that the insertions are done correctly. You may verify it manually or use the visualization tool at https://www.cs.usfca.edu/~galles/visualization/BST.html. (Links to an external site.) Do the following: 1. add code to test the deleteNode and search member functions. Save screenshot of your test program run. 2. add code to implement the nodeCount member function of class BinaryTree. Test it and save screenshot of your test program run. 3. add code to implement the leafCount member function of class BinaryTree. Test it and…arrow_forwardHow do I implement a file with sets of numbers, such as 0 4 2 6 0 9 1 5 to an adjacency matrix JAVA?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
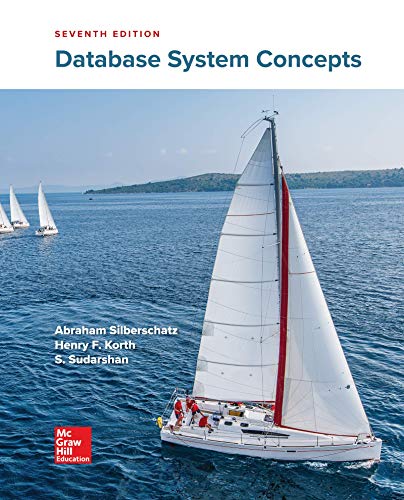
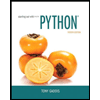
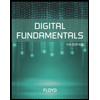
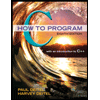
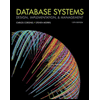
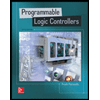