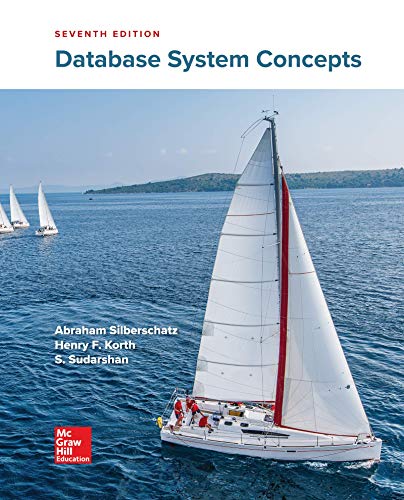
DEBUG Exercise 1.
// This file defines the Patient class used by
// a doctor's office. The Patient class has two
// overloaded constructors -- a default constructor,
// and one that requires an ID number and full name.
// A demonstration program declares two Patient objects.
class Patient
Declarations
private string idNum
private string lastName
private string firstName
private Patient()
idNum = "0000"
lastName = "XXXX"
firstName = "XXXX"
return
public Patient(num id, string last, string first)
this.id = id
lastName = last
firstName = first
return
public string display()
output "Patient #", idNum, lastName, firstName
return
endClass
start
Declarations
Patient patient1
Patient patient2("234", "Lee", "Lydia")
patient1.display()
patient2.display()
stop
DEBUG Exercise 2

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Summary In this lab, you create a derived class from a base class, and then use the derived class in a Python program. The program should create two Motorcycle objects, and then set the Motorcycle’s speed, accelerate the Motorcycle object, and check its sidecar status. Instructions Open the file named Motorcycle.py. Create the Motorcycle class by deriving it from the Vehicle class. Call the parent class __init()__ method inside the Motorcycle class's __init()__ method. In theMotorcycle class, create an attribute named sidecar. Write a public set method to set the value for sidecar. Write a public get method to retrieve the value of sidecar. Write a public accelerate method. This method overrides the accelerate method inherited from the Vehicle class. Change the message in the accelerate method so the following is displayed when the Motorcycle tries to accelerate beyond its maximum speed: "This motorcycle cannot go that fast". Open the file named MyMotorcycleClassProgram.py. In the…arrow_forwardWhen a class uses dynamically allocated objects for its members and it does not have a copy assignment operator what of the following could happen (mark all that apply) There could be runtime errors There could be a double frees (double delete errors) There could be memory leaks Nothing happens the compiler provides the correct constructor There could be compile time errorsarrow_forwardpublic class VisitorPackage{// Declare data fields: an int named travelerID, // a String named destination, and // a double named totalCost.// Your code here... // Implement the default contructor.// Set the value of destination to N/A, and choose an // appropriate value for the other data fields,// Your code here... // Implement the overloaded constructor that passes new// values to all data fields.// Your code here... // Implement method getTotalCost to return the totalCost.// Your code here... // Implement method applyDiscount.//// This method applies a discount off of the total cost// of the tour. The way the discount is applied depends// on the kind of visitor.//// Child discount: the unit discount is subtracted ONCE// from the total cost of the tour.// Senior discount: the unit discount is subtracted TWICE// from the total cost of the tour.// Parameters:// - a char to indicate the kind of visitor// ('c' for child, 's' for senior)// - a double that indicates the amount of the…arrow_forward
- Written in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardpublic class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forwardProgram: File GamePurse.h: class GamePurse { // data int purseAmount; public: // public functions GamePurse(int); void Win(int); void Loose(int); int GetAmount(); }; File GamePurse.cpp: #include "GamePurse.h" // constructor initilaizes the purseAmount variable GamePurse::GamePurse(int amount){ purseAmount = amount; } // function definations // add a winning amount to the purseAmount void GamePurse:: Win(int amount){ purseAmount+= amount; } // deduct an amount from the purseAmount. void GamePurse:: Loose(int amount){ purseAmount-= amount; } // return the value of purseAmount. int GamePurse::GetAmount(){ return purseAmount; } File main.cpp: // include necessary header files #include <stdlib.h> #include "GamePurse.h" #include<iostream> #include<time.h> using namespace std; int main(){ // create the object of GamePurse class GamePurse dice(100); int amt=1; // seed the random generator srand(time(0)); // to play the…arrow_forward
- A field has access that is somewhere between public and private. static final Opackage Oprotectedarrow_forward1. RetailItem Class Write a class named Retailltem that holds data about an item in a retail store. The class should have the following fields: • description. It is a String object that holds a brief description of the item. • unitsOnHand. It is an int variable that holds the number of units currently in inventory. • price. It is a double that holds the item's retail price. Write appropriate mutator methods that store values in these fields and accessor methods that return the values in these fields. Once you have written the class, write a separate program that creates three RetailItem objects and stores the following data in them. Units On Hold Description Designer Jeans Jacket Price 40 34.95 12 59.95 Shirt 20 24.95arrow_forward11:02 all 10% + Create 1 1000.01 Create 2 2000.02 Create 3 3000.03 Deposit 111.11 Deposit 2 22.22 Withdraw 4 5000.00 Create 4 4000.04 Withdraw 1 0.10 Balance 2 Withdraw 2 0.20 Deposit 3 33.33 Withdraw 4 0.40 Bad Command 65 Balance 1 Balance 2 Balance 3 Balance 4arrow_forward
- class Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.Major = m def set_id(self, newid): #This is known as setter self.id = newid def get_id(self): #This is known as a getter return self.id def set_fn(self, newfirstName): self.fn = newfirstName def get_fn(self): return self.fn def set_ln(self, newlastName): self.ln = newlastName def get_ln(self): return self.ln def set_dob(self, newdob): self.dob = newdob def get_dob(self): return self.dob def set_m(self, newMajor): self.m = newMajor def get_m(self): return self.m def print_student_info(self): print(f'{self.id} {self.firstName} {self.lastName} {self.dateOfBirth} {self.Major}')all_students = []id=100user_input = int(input("How many students: "))for x in range(user_input): firstName = input('Enter…arrow_forwardclass IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */ ? int count; public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */ ? /* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */ ? virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };arrow_forwardthis is my retail item code: //Import statements public class RetailItem { private String description; private int units; private double price; public RetailItem() { } public RetailItem(String x, int y, double z) { description = x; units = y; price = z; } public RetailItem(RetailItem i) { } public void setDescription(String x) { description = x; } public void setPrice(double z) { price = z; } void setUnits(int y) { units = y; } public int getUnits() { return units; } public String getDescription() { return description; } public double getPrice() { return price; } // Main class public static void main(String[] args) { String str = "Shirt"; RetailItem r1 = new RetailItem("Jacket", 12,…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
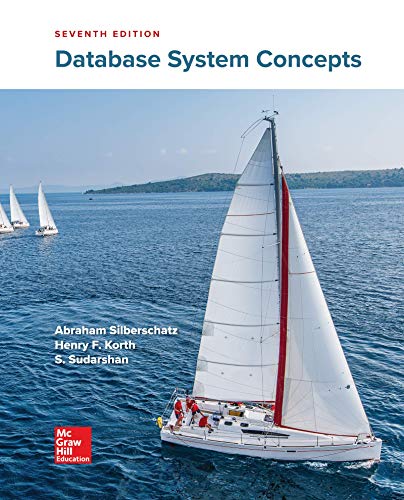
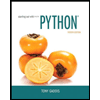
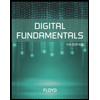
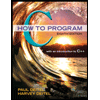
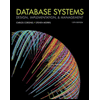
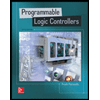