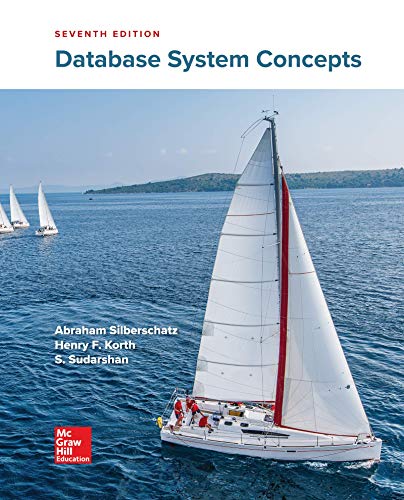
public class VisitorPackage
{
// Declare data fields: an int named travelerID,
// a String named destination, and
// a double named totalCost.
// Your code here...
// Implement the default contructor.
// Set the value of destination to N/A, and choose an
// appropriate value for the other data fields,
// Your code here...
// Implement the overloaded constructor that passes new
// values to all data fields.
// Your code here...
// Implement method getTotalCost to return the totalCost.
// Your code here...
// Implement method applyDiscount.
//
// This method applies a discount off of the total cost
// of the tour. The way the discount is applied depends
// on the kind of visitor.
//
// Child discount: the unit discount is subtracted ONCE
// from the total cost of the tour.
// Senior discount: the unit discount is subtracted TWICE
// from the total cost of the tour.
// Parameters:
// - a char to indicate the kind of visitor
// ('c' for child, 's' for senior)
// - a double that indicates the amount of the discount.
//
// Consider the case when the kind is neither 'c' or 's'
// and output a message, "Discount cannot be applied."
//
// Does not return a value.
public void applyDiscount(char kind, double discount)
{
// Your code here...
// Implement method applyWeekdayRate
// Subtracts the discount, computed from the percentage,
// from the total cost.
//
// Parameter: a double to indicate a percentage (1.0 to 99.0)
//
// Example: If the tour cost so far is $150.00 and the percentage
// is 10, then the tour cost will go down to $135.00.
//
// Does not return a value.
public void applyWeekdayRate(double percentage)
{
// Your code here...
// Implement method printInfo.
// Prints out the visitor ID, the destination, and the
// total cost of the tour.
//
// Output is formatted on one line separated by dashes
// in the format: id - destination - $totalCost
//
// The total cost is formatted with 2 decimal places.
//
// Does not return a value.
public void printInfo()
{
// Your code here...
public class Z_RunVP
{
public static void main( String args[] )
{
// GROUP 1:
// John and Luke are going to Six Flags.
// The price for a ticket is $65.00 per person.
// John's id is 765, and Luke's id is 432.
// They are going on a weekday.
// Luke is a senior.
//
// 1. Declare two objects (john and luke) and initialize
// their id, destination, and cost....
// 2. Use method applyWeekdayRate to apply a 20% discount
// for both John and Luke....
// 3. Use method applyDiscount to apply a discount with
// unit amount $12.50 for Luke.
// Remember that Luke is a senior....
// 4. Print out the information for both John and Luke
// using the printInfo method....
// 5. Calculate and output the total cost of their tour.
// (Output the cost to two decimal places.)
// Hint: Use the get method to get the total cost for
// each and sum them up....
System.out.println();
// GROUP 2:
// Peter, Paul, and Mary are going to Disneyland.
// The price for the ticket is $99.00 per person.
// Peter's id is 413, Paul's id is 189, and Mary's id is 516.
// Paul and Mary are children. The unit discount is $8.95.
//
// 1. Declare and initialize three objects (peter,
// paul, and mary)....
// 2. Use method applyWeekdayRate to apply a 15%
// discount for Peter and Paul.
// 3. Use the method applyDiscount to apply the
// child discount for Paul and Mary....
// 4. Print out the information for all three of them....
// 5. Calculate and output the total cost of
// their tour (to 2 decimal places)....

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Written in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forward28arrow_forwardData structure & Algrothium java program Create the three following classes: 1. class containing two strings attributes first name and last name.2. class containing the name object and an ArrayList of string to store the list gifts. This class would extend the attached NicePersonInterface.java3. class containing one ArrayList of Names to store the naughty names. Another ArrayList of NicePerson to store the nice names and gifts. Add atleast 4 names in each list and display all information.arrow_forward
- class implementation file -- Rectangle.cpp class Rectangle { #include #include "Rectangle.h" using namespace std; private: double width; double length; public: void setWidth (double); void setLength (double) ; double getWidth() const; double getLength() const; double getArea () const; } ; // set the width of the rectangle void Rectangle::setWidth (double w) { width = w; } // set the length of the rectangle void Rectangle::setLength (double l) { length l; //get the width of the rectangle double Rectangle::getWidth() const { return width; // more member functions herearrow_forwardN-SIDED REGULAR POLYGON) In an n-sided regular polygon, all sides have the same length and all angles have the same degree (i.e., the polygon is both equilateral and equiangular). Design a class named RegularPolygon that contains: ▪ A private int data field named n that defines the number of sides in the polygon with default value 3. ▪ A private double data field named side that stores the length of the side with default value 1. A private double data field named x that defines the x-coordinate of the polygon's center with default value 0. ▪ A private double data field named y that defines the y-coordinate of the polygon's center with default value 0. A no-arg constructor that creates a regular polygon with default values. A constructor that creates a regular polygon with the specified number of sides and length of side, centered at (0, 0). A constructor that creates a regular polygon with the specified number of sides, length of side, and x- and y-coordinates. ▪ The accessor and…arrow_forwardclass IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */ ? int count; public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */ ? /* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */ ? virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };arrow_forward
- class Container {private int val1=1;private void printValue(){final int val2=2;class Containee{public void f(){System.out.println(String.format("Values are: %d and %d."),val1);}}}public static void main(String[] args) {(new Container()).printValue();}} a.The above does not compile because the method f() requires an instance of class Container b.The above does not compile because the class definition in done within the body of the function c.The above code complies with no errors, but does not produce any ouput d.The above code does not compile because the methodf() cannot access the variable val1arrow_forwardHere is a statement: class temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const; temporary(string = "", double = 0.0, double = 0.0); private: string description; double first; double second; }; How can I write a definition of the member function manipulate that returns a decimal number as follows: If the value of description is "rectangle", it returns first * second; if the value of description is "circle", it returns the area of the circle with radius first; if the value of description is "sphere", it returns the volume of the sphere with radius first; if the value of description is "cylinder", it returns the volume of the cylinder with radius first and height second; otherwise, it returns the value -1.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
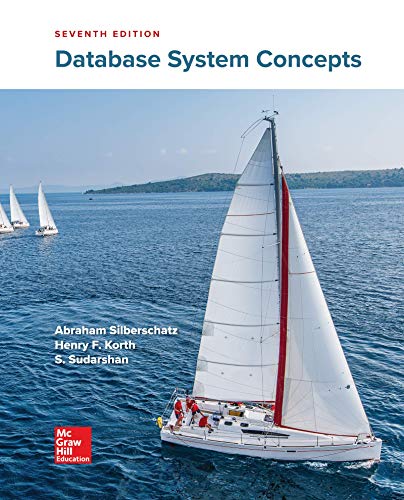
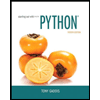
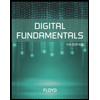
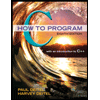
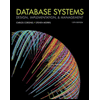
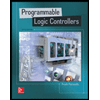