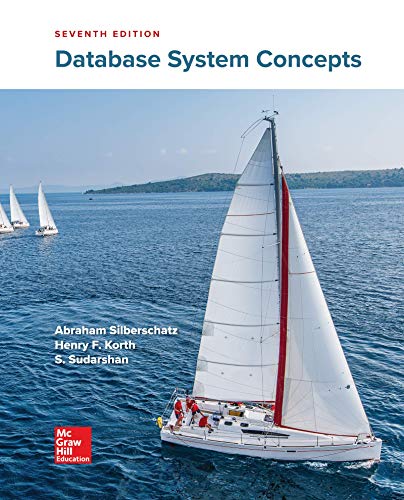
Ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN.
I am having trouble with my code
here is my code
import java.util.Comparator;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Scanner;
class Car {
String manufacturer;
String model;
int year;
String vin;
public Car(String manufacturer, String model, int year, String vin) {
super();
this.manufacturer = manufacturer;
this.model = model;
this.year = year;
this.vin = vin;
}
public String getManufacturer() {
return manufacturer;
}
public void setManufacturer(String manufacturer) {
this.manufacturer = manufacturer;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public String getVin() {
return vin;
}
public void setVin(String vin) {
this.vin = vin;
}
}
class CarComparator implements Comparator<Car> {
@Override
public int compare(Car car1, Car car2) {
int yearCompare = Integer.compare(car1.getYear(), car2.getYear());
if (yearCompare != 0) {
return yearCompare;
}
return car1.getVin().compareTo(car2.getVin());
}
}
public class OldestCarsByMake {
public static void main(String[] args) throws FileNotFoundException{
ArrayList<Car> cars = new ArrayList<Car>();
Scanner sc1 = new Scanner(System.in);
System.out.println("Enter filename");
String carFile= sc1.next();
File file = new File(carFile);
Scanner sc2 = new Scanner(file);
String[] carDetails;
sc2.nextLine();
while(sc2.hasNext()){
String carLine = sc2.nextLine();
carDetails=carLine.split("\\s+");
String manufacturer=carDetails[0];
String model = carDetails[1];
int year = Integer.parseInt(carDetails[2]);
String vin = carDetails[3];
boolean flag=true;
for(Car c : cars){
if(c.manufacturer.equals(manufacturer)){
flag=false;
if(c.year>year){
c.setModel(model);
c.setYear(year);
c.setVin(vin);
}
else if(c.year==year){
if((c.vin).compareTo(vin)>0){
c.setModel(model);
c.setYear(year);
c.setVin(vin);
}
}
}
}
if(flag)
cars.add(new Car(manufacturer,model,year,vin));
}
Collections.sort(cars, new CarComparator());
int spaces=25;
for(Car c: cars){
for(int i=0;i<20-(c.manufacturer.length());i++)
System.out.print(" ");
System.out.print(c.manufacturer);
for(int i=0;i<20-(c.model.length());i++)
System.out.print(" ");
System.out.println(c.model+" "+c.year+" "+c.vin);
}
System.out.println(cars.size()+" result(s)");
}
}
My code is producing an error an wont run in the program
Enter filename\n
car-list-3.txtENTER
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 1 out of bounds for length 1\n
\tat OldestCarsByMake.main(OldestCarsByMake.java:68)\n
i am not sure where i am going wrong with my code, can an expert fix my code and explain the issue
i need it to produce
Enter filename\n
car-list-3.txtENTER
Oldest cars by make\n
Acura Legend 1988 YV1672MK8A2784103\n
Aptera Typ-1 2009 19UUA56952A698282\n
Aston Martin DB9 2006 JTDJTUD36ED662608\n
Audi 5000S 1985 JN1CV6EK1CM209730\n
Austin Mini Cooper 1964 1G6KD57Y86U095255\n
Bentley Continental GT 2006 19UUA655X4A245718\n
BMW 7 Series 1993 1FM5K7B80FG198521\n
Buick LeSabre 1986 5FRYD4H82EB970786\n
Cadillac Fleetwood 1992 WAUSVAFA0AN244068\n
Chevrolet Corvette 1957 WBA3V7C57F5095471\n
Chrysler Town & Country 1994 WBANU53558B610150\n
Daewoo Lanos 1999 2HNYD18412H169358\n
Daihatsu Charade 1992 JH4CL95958C112754\n
Dodge D150 1993 19XFA1F37BE391950\n....

Step by stepSolved in 6 steps with 1 images

- Ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN. I am having trouble with my code here is my code import java.util.Comparator;import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Collections;import java.util.Scanner;class Car {String manufacturer;String model;int year;String vin;public Car(String manufacturer, String model, int year, String vin) {super();this.manufacturer = manufacturer;this.model = model;this.year = year;this.vin = vin;}public String getManufacturer() {return manufacturer;}public void setManufacturer(String manufacturer) {this.manufacturer = manufacturer;}public String getModel() {return model;}public void setModel(String model) {this.model = model;}public int getYear() {return year;}public void setYear(int year) {this.year = year;}public String getVin() {return vin;}public void setVin(String vin) {this.vin = vin;}}class…arrow_forwardBuild an application for a car rental company. It should offer the following features:• Maintain a customer database in a file:o Register / add new customers. Customer information should include▪ Customer ID number▪ Name▪ Phone numbero Search customer database by:▪ Customer ID number▪ Name▪ Phone numberAnd print out the matching records NOTE ; i need the answer to be by switcharrow_forwardFocus on string operations and methods You work for a small company that keeps the following information about its clients: • first name • last name • a 5-digit user code assigned by your company. The information is stored in a file clients.txt with the information for each client on one line (last name first), with commas between the parts. For example Jones, Sally,00345 Lin ,Nenya,00548 Fule,A,00000 Smythe , Mary Ann , 00012 Your job is to create a program assign usernames for a login system. First: write a function named get_parts(string) that will that will receive as its arguments a string with the client data for one client, for example “Lin ,Nenya,00548”, and return the separate first name, last name, and client code. You should remove any extra whitespace from the beginning and newlines from the end of the parts. You’ll need to use some of the string methods that we covered in this lesson You can test your function by with a main() that is just the function call with the…arrow_forward
- Ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN. I am having trouble with a specific line of my code here is my code import java.util.Comparator; import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Collections; import java.util.Scanner; class Car { String manufacturer; String model; int year; String vin; public Car(String manufacturer, String model, int year, String vin) { super(); this.manufacturer = manufacturer; this.model = model; this.year = year; this.vin = vin; } public String getManufacturer() { return manufacturer; } public void setManufacturer(String manufacturer) { this.manufacturer = manufacturer; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } public String getVin() { return vin;…arrow_forwardI am trying to read a CSV file and then store it into an ArrayList. For each row, I'm trying to create a new Country class instance and add it to the list. However, it is giving me the following as the output: edu.uga.cs1302.quiz.Country@7c9bb6b8 edu.uga.cs1302.quiz.Country@441847 edu.uga.cs1302.quiz.Country@2621fba7 edu.uga.cs1302.quiz.Country@4fa6e7a1 edu.uga.cs1302.quiz.Country@6e29b69b edu.uga.cs1302.quiz.Country@4e0f0d39 edu.uga.cs1302.quiz.Country@67da3b9c edu.uga.cs1302.quiz.Country@1f394329 edu.uga.cs1302.quiz.Country@3c07b33b edu.uga.cs1302.quiz.Country@a570747 edu.uga.cs1302.quiz.Country@3ce7cb4a edu.uga.cs1302.quiz.Country@6912e7f4 edu.uga.cs1302.quiz.Country@680d0f86 edu.uga.cs1302.quiz.Country@5a5250ff edu.uga.cs1302.quiz.Country@58ed7d64 edu.uga.cs1302.quiz.Country@26be1cca edu.uga.cs1302.quiz.Country@26cf56a4 edu.uga.cs1302.quiz.Country@6ed22f2a edu.uga.cs1302.quiz.Country@5de769c9 edu.uga.cs1302.quiz.Country@b6966f3 edu.uga.cs1302.quiz.Country@574f6b4c…arrow_forwardcreate a class Person to represent a person and another one to represent the database. A dictionary should be a data member of the database class, with Person objects as search keys for this dictionary. Write a program that tests and demonstrates your database.arrow_forward
- If we want to filter out the results and shows only the values of certain properties, such as Name, Status of Get-Service cmdlet, we can use this cmdlet: Group of answer choices Remove-Item Select-String Select-Object Sort-Objectarrow_forwardCreate a datafile that contains the first name, last name, gender, age, height, smoking preference, eye color and phone number. Add a variety of records to the file. A sample file looks like:  Write a program that opens the file and reads the records one by one. The program will skip any records where the gender preference is not a match. Of those records that match the gender preference, check to see if the age and height are between the maximum and minum preferences. Then check to see if the smoking preference and eye color are also a match. If at least 3 of the remaining fields match, consider the record a partial match, and print it in the report. If all 4 of the remaining fields match, the record is a perfect match and print it in the report with an asterisk next to it. At the end of the program, close the file and report how many total records there were of the specified gender, how many were a partial match, and how many were a perfect match.arrow_forwardAsk the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN. i am having trouble getting my code to work this is my code: import java.util.*;import java.io.*;class Car {String manufacturer;String model;int year;String vin;public Car(String manufacturer, String model, int year, String vin) {super();this.manufacturer = manufacturer;this.model = model;this.year = year;this.vin = vin;}public String getManufacturer() {return manufacturer;}public void setManufacturer(String manufacturer) {this.manufacturer = manufacturer;}public String getModel() {return model;}public void setModel(String model) {this.model = model;}public int getYear() {return year;}public void setYear(int year) {this.year = year;}public String getVin() {return vin;}public void setVin(String vin) {this.vin = vin;}}class CarComparator implements Comparator < Car > {@Overridepublic int compare(Car car1, Car car2) {int yearCompare =…arrow_forward
- Using Go, return a Movie with the given title and genre. The Views, Likes, and Dislikes should all be left in their zero-vale states. type Movie struct { Title string Genre string Likes int Dislikes int Views int } func NewMovie(title, genre string) *Movie { return &Movie { } }arrow_forwardThe following sample file called grades.txt contains grades for several students in an imaginary class. Each line of this file stores a student's name followed by their exam scores. The number of scores might be different for each student. abdul 10 15 20 30 40erica 23 16 19 22haroon 8 22 17 14 32 17 24 21 2 9 11 17omar 12 28 21 45 26 10chengjie 14 32 25 16 89alex 15 22 11 35 36 7 9bryan 34 56 11 29 6laxman 24 23 9 45 27 Now, consider the following code. f = open("grades.txt", "r") for aline in f: items = aline.split() # choose your option for this part f.close() Which option prints out only the names of each student in stored in grades.txt? Question 11 options: print(items[0:]) print(items[0]) print(items[0][0])arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
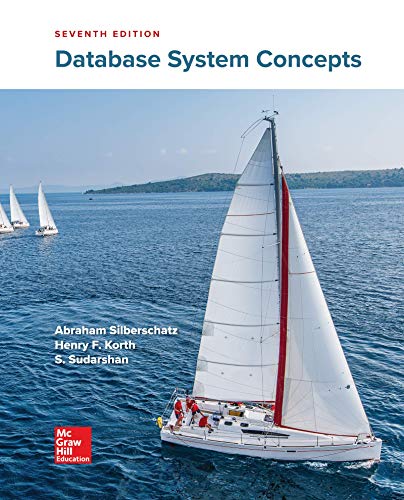
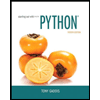
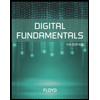
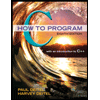
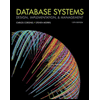
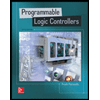