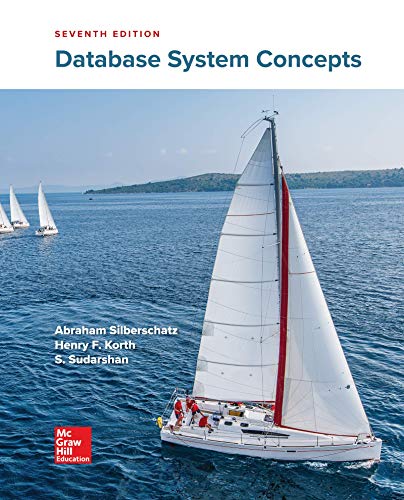
Ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN.
i am having trouble getting my code to work
I have this code:
import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner; class Car { String manufacturer; String model; int year; String vin; public Car(String manufacturer, String model, int year, String vin) { this.manufacturer = manufacturer; this.model = model; this.year = year; this.vin = vin; } public String getManufacturer() { return manufacturer; } public void setManufacturer(String manufacturer) { this.manufacturer = manufacturer; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } public String getVin() { return vin; } public void setVin(String vin) { this.vin = vin; } } public class Demo { public static void main(String[] args) throws FileNotFoundException { Scanner keyboard = new Scanner(System.in); System.out.println("Enter filename"); String input = keyboard.nextLine(); File file = new File(input); Scanner scanner = new Scanner(file); scanner.nextLine(); ArrayList<Car> list = new ArrayList<>(); while (scanner.hasNextLine()) { String[] arr = scanner.nextLine().split(" "); if (arr.length >= 4) { Car car = new Car(arr[0], arr[1], Integer.parseInt(arr[2]), arr[3]); list.add(car); } } for (int end = list.size() - 1; end >= 1; end--) { for (int current = 0; current <= end - 1; current++) { Car car1 = list.get(current); Car car2 = list.get(current + 1); int n = car1.manufacturer.toLowerCase().compareTo(car2.manufacturer.toLowerCase()); if (n == 0) { n = car1.year - car2.year; } if (n == 0) { n = car1.vin.compareTo(car2.vin); } if (n > 0) { Car temp = list.get(current); list.set(current, list.get(current + 1)); list.set(current + 1, temp); } } } // Find the oldest car for each manufacturer and print the results System.out.println("Oldest cars by make"); Car oldest = null; int count = 0; for (int i = 0; i < list.size() - 1; i++) { if (oldest == null) { oldest = list.get(i); } if (!list.get(i).manufacturer.equals(list.get(i + 1).manufacturer) || i == list.size() - 2) { if (i == list.size() - 2 && list.get(i).manufacturer.equals(list.get(i + 1).manufacturer)) { oldest = (oldest.year < list.get(i + 1).year) ? oldest : list.get(i + 1); } count++; System.out.println(String.format("%15s%25s%5s %s", oldest.manufacturer, oldest.model, oldest.year, oldest.vin)); oldest = null; } } System.out.println(count + " result(s)"); } }
it is supposed to display
Enter filename\n
car-list-3.txtENTER
Oldest cars by make\n
Acura Legend 1988 YV1672MK8A2784103\n
Aptera Typ-1 2009 19UUA56952A698282\n
Aston Martin DB9 2006 JTDJTUD36ED662608\n
Audi 5000S 1985 JN1CV6EK1CM209730\n
Austin Mini Cooper 1964 1G6KD57Y86U095255\n
Bentley Continental GT 2006 19UUA655X4A245718\n
BMW 7 Series 1993 1FM5K7B80FG198521\n
Buick LeSabre 1986 5FRYD4H82EB970786\n
Cadillac Fleetwood 1992 WAUSVAFA0AN244068\n
Chevrolet Corvette 1957 WBA3V7C57F5095471\n
Chrysler Town & Country 1994 WBANU53558B610150\n
Daewoo Lanos 1999 2HNYD18412H169358\n
Daihatsu Charade 1992 JH4CL95958C112754\n...
56 result(s)\n
but it is diaplying errors
Enter filename\n
car-list-3.txtENTER
Exception in thread "main" java.lang.NumberFormatException: For input string: "Rover"\n
\tat java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:67)\n
\tat java.base/java.lang.Integer.parseInt(Integer.java:665)\n
\tat java.base/java.lang.Integer.parseInt(Integer.java:781)\n
\tat Demo.main(Demo.java:53)\n
i need my code to be fixed so that is displays the information properly to display the oldest car for every manufacturer from that file and If two cars have the same year, compare based on the VIN.
i am not sure where i am going wrong with my code, the code is based on sorting and searching

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 3 images

- GDB and Getopt Class Activity activity [-r] -b bval value Required Modify the activity program from last week with the usage shown. The value for bval is required to be an integer as is the value at the end. In addition, there should only be one value. Since everything on the command line is read in as a string, these now need to be converted to numbers. You can use the function atoi() to do that conversion. You can do the conversion in the switch or you can do it at the end of the program. The number coming in as bval and the value should be added together to get a total. That should be the only value printed out at the end. Total = x should be the only output from the program upon success. Remove all the other print statements after testing is complete. Take a screenshot of the output to paste into a Word document and submit. Practice Compile the program with the -g option to load the symbol table. Run the program using gdb and use watch on the result so the program stops when the…arrow_forwardA for construct is a loop that goes over a list of objects. Consequently, it runs indefinitely if there are items to process. What do you think about this?arrow_forwardFor items 1–3, use the IN300_Dataset1.csv file. Write a Python program that reads the CSV file into a Panda dataframe. Using that dataframe, print the row, source IP, and destination IP as a table. Write a Java program that reads the CSV file into an ArrayList. Convert the ArrayList to a string array and print the row, source IP, and destination IP on the same line using a loop. Write an R program that reads the CVS file using the read.csv data type. Print the row, source IP and destination IP of each line.arrow_forward
- A for construct is used to build a loop that processes a list of elements in programming. To do this, it continues to operate forever as long as there are objects to process. Is this assertion truthful or false? Explain your response.arrow_forwardThe code given below represents a saveTransaction() method which is used to save data to a database from the Java program. Given the classes in the image as well as an image of the screen which will call the function, modify the given code so that it loops through the items again, this time as it loops through you are to insert the data into the salesdetails table, note that the SalesNumber from the AUTO-INCREMENT field from above is to be inserted here with each record being placed into the salesdetails table. Finally, as you loop through the items the product table must be update because as products are sold the onhand field in the products table must be updated. When multiple tables are to be updated with related data, you should wrap it into a DMBS transaction. The schema for the database is also depicted. public class PosDAO { private Connection connection; public PosDAO(Connection connection) { this.connection = connection; } public void…arrow_forwardjava program For this question, the server contains the id, name and cgpa of some Students in a file. The client will request the server for specific data, which the server will provide to the client. Server: The server contains the data of some Students. For each student, the server contains id, name and cgpa data in a file. Here's an example of the file: data.txt101 Saif 3.52201 Hasan 3.81.... Create a similar file in you server side. The file should have at least 8 students. The client will request the server to provide the details (id, name, cgpa) of the Nth highest cgpa student in the file. If N's value is 1, the server will return the details of the student with the highest cgpa. if N's value is 3, the server will return the details of the student with the third highest cgpa. If N's value is incorrect, the server returns to the client: "Invalid request". Client: The client sends the server the value of N, which is an integer number. The client takes input the value of N…arrow_forward
- Python S3 Get File In the Python file, write a program to get all the files from a public S3 bucket named coderbytechallengesandbox. In there there might be multiple files, but your program should find the file with the prefix and cb - then output the full name of the file. You should use the boto3 module to solve this challenge. You do not need any access keys to access the bucket because it is public. This post might help you with how to access the bucket. Example Output ob name.txt Browse Resources Search for any help or documentation you might need for this problem. For exampler array indexing, Ruby hash tables, etc.arrow_forwardwrite in c++Write a program that would allow the user to interact with a part of the IMDB movie database. Each movie has a unique ID, name, release date, and user rating. You're given a file containing this information (see movies.txt in "Additional files" section). The first 4 rows of this file correspond to the first movie, then after an empty line 4 rows contain information about the second movie and so forth. Format of these fields: ID is an integer Name is a string that can contain spaces Release date is a string in yyyy/mm/dd format Rating is a fractional number The number of movies is not provided and does not need to be computed. But the file can't contain more than 100 movies. Then, it should offer the user a menu with the following options: Display movies sorted by id Display movies sorted by release date, then rating Lookup a release date given a name Lookup a movie by id Quit the Program The program should perform the selected operation and then re-display the menu. For…arrow_forwardLoad the airline JSON file into a python dictionary, save it to a text file and print the airlines that operated in Boston and SanFrancisco airports during the month of June.arrow_forward
- Written in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardvoid is a datatype in C. a. True оъ. Falsearrow_forward1) Take the supplied cars_db.sql and create your Cars database (you will have to create the Cars database first, then execute the .sql file from within Netbeans). 2) Implement a program that has the same general user interface as the SimpleSchoolData presented in class. 3) Implement two classes, CarsDisplay which displays the above application, and implement a class named CarQueries that contains the prepared statements to execute the queries. (Java) This is the cars_db.sql DROP TABLE Cars; CREATE TABLE Cars ( CarID INT NOT NULL GENERATED ALWAYS AS IDENTITY, CarMake VARCHAR (10) NOT NULL, CarModel VARCHAR (15) NOT NULL, CarYear VARCHAR (4) NOT NULL, CarMileage DECIMAL (6) NOT NULL ); INSERT INTO Cars (CarMake,CarModel,CarYear,CarMileage) VALUES ('Honda','Civic','1998',135647), ('Honda','Accord','2009',46877), ('Nissan','Pickup','1997',235444);arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
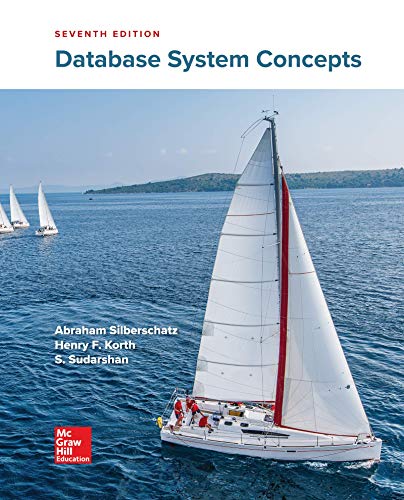
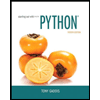
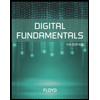
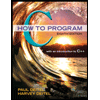
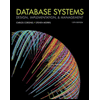
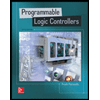