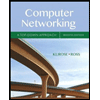
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
Code In Java Using Array or doubly Linklist instead of linklist
COVID-19 has effect whole world. People are interested in knowing current situation of COVID-19. You are required to write JAVA program for storing information in a Array Following JAVA classes are provided.
// Node class
public class COVIDDetail
{
public String country;
public int activecases;
public COVIDDetail next;
}
// linked List class
public class COVIDList
{
COVIDDetail start=null;
}
You are required to add following methods to COVIDList class
- void add(COVIDDetail n) this method receives a node as argument and adds it to given list. Nodes are arranged in descending order with respect to number of cases. Note that you are not required to sort the list in fact while adding first find its appropriate position and then add given node to that position.
- void updateCases(String country_name , int cases) this method receives two argument country name and cases (can have positive or negative value). Update active cases of those countries whose name matches with the one provided as argument, by adding activecases with cases (value provided as argument).
- String maxCases( ) returns name of country with maximum number of cases.
- void displayData( ) display data of least effected country.
- COVIDList covidFreeCountries( ) returns new list of those countries which are COVID free. Country whose active cases are 0 is considered to be COVID free.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- Please help convert the following Java coding to C++ /LinkedList.java============== //Interface public interface LinkedList<T> { public boolean isEmpty(); public void insertAtStart(T data); public void insertAtEnd(T data); public void deleteAtStart(); public void deleteAtEnd(); public T getStartData(); public T getEndData(); public void deleteLinkedList(); } //===end of LinkedList.java===== //==LinkNode.java================ public class LinkNode<T> { private T data; private LinkNode<T> next; public LinkNode(T data,LinkNode<T> next) { this.setData(data); this.setNext(next); } public T getData() { return data; } public void setData(T data) { this.data = data; } public LinkNode<T> getNext() { return next; } public void setNext(LinkNode<T> next) { this.next = next; } } //==end of LinkNode.java========= //===singlyLinkedList.java============= public…arrow_forwardGiven main() in the Inventory class, define an insertAtFront() method in the InventoryNode class that inserts items at the front of a linked list (after the dummy head node). Ex. If the input is: 4 plates 100 spoons 200 cups 150 forks 200 the output is: 200 forks 150 cups 200 spoons 100 plates public class InventoryNode { private String item; private int numberOfItems; private InventoryNode nextNodeRef; // Reference to the next node public InventoryNode() { item = ""; numberOfItems = 0; nextNodeRef = null; } // Constructor public InventoryNode(String itemInit, int numberOfItemsInit) { this.item = itemInit; this.numberOfItems = numberOfItemsInit; this.nextNodeRef = null; } // Constructor public InventoryNode(String itemInit, int…arrow_forwardpackage hw5; public class LinkedIntSet {private static class Node {private int data;private Node next; public Node(int data, Node next) {this.data = data;this.next = next;}} private Node first; // Always points to the first node of the list.// THE LIST IS ALWAYS IN SORTED ORDER!private int size; // Always equal to the number of elements in the set. /*** Construts an empty set.*/public LinkedIntSet() {throw new RuntimeException("Not implemented");} /*** Returns the number of elements in the set.* * @return the number of elements in the set.*/public int size() {throw new RuntimeException("Not implemented");} /*** Tests if the set contains a number* * @param i the number to check* @return <code>true</code> if the number is in the set and <code>false</code>* otherwise.*/public boolean contains(int i) {throw new RuntimeException("Not implemented");} /*** Adds <code>element</code> to this set if it is not already present and* returns…arrow_forward
- Given main(), complete the program to add people to a queue. The program should read in a list of people's names including "You" (ending with -1), adding each person to the peoplelnQueue queue. Then, remove each person from the queue until "You" is at the head of the queue. Include print statements as shown in the example below. Ex. If the input is Zadie Smith Tom Sawyer You Louisa Alcott the output is Welcome to the ticketing service... You are number 3 in the queue. Zadie Smith has purchased a ticket. You are now number 2 Tom Sawyer has purchased a ticket. You are now number 1 You can now purchase your ticket!arrow_forwardCould you help me the following question? The Node structure is defined as follows (we will use integers for data elements): public class Node { public int data; public Node next; public Node previous; } The Double Ended Doubly Linked List (DEDLL) class definition is as follows: public class DEDLL { public int currentSize; public Node head; public Node tail; } This design is a modification of the standard linked list. In the standard that we discussed in class, there is only a head node that represents the first node in the list and each node only contains a reference to the next node in the list until the chain of nodes reach null. In this DEDLL, each node also has a reference to the previous node. The DEDLL also contains a reference to the tail of the list that represents the last node in the list. For example, a DEDLL with 4 elements may have nodes with values 5, 2, 6, and 8 from head to tail. This…arrow_forwardModify the Java class for the abstract stack type shown belowto use a linked list representation and test it with the same code thatappears in this chapter. class StackClass {private int [] stackRef;private int maxLen,topIndex;public StackClass() { // A constructorstackRef = new int [100];maxLen = 99;topIndex = -1;}public void push(int number) {if (topIndex == maxLen)System.out.println("Error in push–stack is full");else stackRef[++topIndex] = number;}public void pop() {if (empty())System.out.println("Error in pop–stack is empty"); else --topIndex;}public int top() {if (empty()) {System.out.println("Error in top–stack is empty");return 9999;}elsereturn (stackRef[topIndex]);}public boolean empty() {return (topIndex == -1);}}An example class that uses StackClass follows:public class TstStack {public static void main(String[] args) {StackClass myStack = new StackClass();myStack.push(42);myStack.push(29);System.out.println("29 is: " + myStack.top());myStack.pop();System.out.println("42 is:…arrow_forward
- Implement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 2.) Define SLinkedList Classa. Instance Variables: # Node head # Node tail # int sizeb. Constructorc. Methods # int getSize() //Return the number of nodes of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # int getFirst() //Return the value of the first node of the list. # int getLast()/ /Return the value of the Last node of the list. # Node getHead()/ /Return the head # setHead(Node h)//Set the head # Node getTail()/ /Return the tail # setTail(Node t)//Set the tail # addFirst(E e) //add a new element to the front of the list # addLast(E e) // add new element to the end of the list # E removeFirst()//Return the value of the first node of the list # display()/ /print out values of all the nodes of the list # Node search(E key)//check if a given…arrow_forwardComputer science helparrow_forwardIn Java. The following is a class definition of a linked list Node:class Node{int info;Node next;}Show the instructions required to create a linked list that is referenced by head and stores in order, the int values 13, 6 and 2. Assume that Node's constructor receives no parameters.arrow_forward
- Redesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forwardclass Queue { private static int front, rear, capacity; private static int queue[]; Queue(int c) { front = rear = 0; capacity = c; queue = new int[capacity]; } static void queueEnqueue(int data) { if (capacity == rear) { System.out.printf("\nQueue is full\n"); return; } else { queue[rear] = data; rear++; } return; } static void queueDequeue() { if (front == rear) { System.out.printf("\nQueue is empty\n"); return; } else { for (int i = 0; i < rear - 1; i++) { queue[i] = queue[i + 1]; } if (rear < capacity) queue[rear] = 0; rear--; } return; } static void queueDisplay() { int i; if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } for (i = front; i < rear; i++) { System.out.printf(" %d <-- ", queue[i]); } return; } static void queueFront() { if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } System.out.printf("\nFront Element is: %d", queue[front]);…arrow_forwardPlease help with this Java program, and include explanations and commentsarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
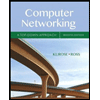
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
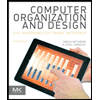
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
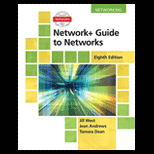
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
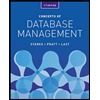
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
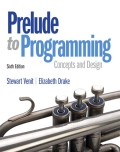
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
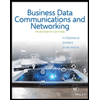
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY