-check this code then, In python 3 make a code that shows the performance curve of the algorithm using time measurements 1. Approach: Non recursive: //include necessary header files #include using namespace std; //main function int main() { int days,buy_on_this_day ,sell_on_this_day; //get number of days as input from user cout<<"Enter number of days: "; cin>>days; int stock_price[days]; for(int i=0;i>stock_price[i]; } int i=0; for(int i=0;i= stock_price[i-1]) i++; sell_on_this_day =i-1; cout< using namespace std; //recurrsive function to find max profit int maxPro(int stock_price[], int starting_day, int last_day) { if (last_day <= starting_day) return 0; int maximum_profit = 0; for (int i = starting_day; i < last_day; i++) { for (int j = i + 1; j <= last_day; j++) { if (stock_price[j] > stock_price[i]) { //update profit int pro = (stock_price[j] - stock_price[i]) + maxPro(stock_price, starting_day, i - 1) + maxPro(stock_price, j + 1, last_day); //update maximum profit maximum_profit = max(maximum_profit, pro); } } } return maximum_profit; } //main function int main() { //declare variables int days,buy_on_this_day ,sell_on_this_day ; //get days and stock price as input cout<<"Enter number of days: "; cin>>days; int stock_stock_price[days]; for(int i=0;i>stock_stock_price[i]; } int ans =maxPro(stock_stock_price,0,days-1); cout<<"max profit: "<
-check this code then, In python 3 make a code that shows the performance curve of the
1. Approach: Non recursive:
//include necessary header files
#include <iostream>
using namespace std;
//main function
int main()
{
int days,buy_on_this_day ,sell_on_this_day;
//get number of days as input from user
cout<<"Enter number of days: ";
cin>>days;
int stock_price[days];
for(int i=0;i<days;i++)
{ cout<<"Enter stock_price";
cin>>stock_price[i];
}
int i=0;
for(int i=0;i<days-1;i++)
{
//comparing current price with next day price and finding the minima
while(i<days-1 && stock_price[i+1]<=stock_price[i])
i++;
if(i==days-1)
break;
buy_on_this_day =i++;
while(i<days && stock_price[i]>= stock_price[i-1])
i++;
sell_on_this_day =i-1;
cout<<buy_on_this_day <<" : index of the change before we buy"<<endl;
cout<<sell_on_this_day<<" :index of the change before we sell"<<endl;
}
int profit;
int maxim =0;
int minSofar = stock_price[0];
for(int i=0;i<days;i++)
{ //calculating the min, profit and max profit
minSofar = min(minSofar, stock_price[i]);// update min s
profit= stock_price[i]-minSofar; // update profit
maxim = max(maxim,profit);// update maximum profit
}
cout<<"Maximum Profit: "<<maxim;
}
//2.APproach:Recurrsive:
//include necessary header files
#include <iostream>
using namespace std;
//recurrsive function to find max profit
int maxPro(int stock_price[], int starting_day, int last_day)
{
if (last_day <= starting_day)
return 0;
int maximum_profit = 0;
for (int i = starting_day; i < last_day; i++)
{
for (int j = i + 1; j <= last_day; j++)
{
if (stock_price[j] > stock_price[i])
{
//update profit
int pro = (stock_price[j] - stock_price[i]) + maxPro(stock_price, starting_day, i - 1) + maxPro(stock_price, j + 1, last_day);
//update maximum profit
maximum_profit = max(maximum_profit, pro);
}
}
}
return maximum_profit;
}
//main function
int main()
{ //declare variables
int days,buy_on_this_day ,sell_on_this_day ;
//get days and stock price as input
cout<<"Enter number of days: ";
cin>>days;
int stock_stock_price[days];
for(int i=0;i<days;i++)
{ cout<<"Enter stock price";
cin>>stock_stock_price[i];
}
int ans =maxPro(stock_stock_price,0,days-1);
cout<<"max profit: "<<ans

Step by step
Solved in 2 steps

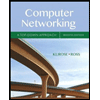
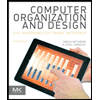
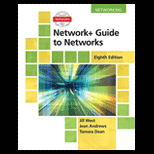
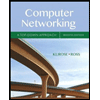
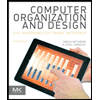
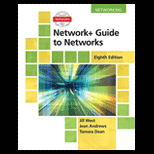
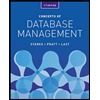
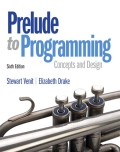
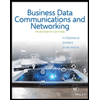