Task - Using pointers to process arrays (C Languaage) In a TV show, each minute can be either interesting or boring. Assume that if 7 consecutive minutes are boring, then an average viewer will stop watching the show. Write a C program that calculates how many minutes that an average viewer will watch a TV show, given the interesting minutes. Assume the TV shows are 45 minutes long. Requirements Name your program project4_minutes.c. Follow the format of the examples below. The program will read in the number of interesting minutes, then read in the interesting minutes. The program should include the following function. Do not modify the function prototype. int find_minute(int *minutes, int n); minutes represents the input array for interesting minutes, n is the length of the array (the number of interesting minutes). The function returns the how many minutes an average viewer will watch the show. This function should use pointer arithmetic– not subscripting – to visit array elements. In other words, eliminate the loop index variables and all use of the [] operator in the function. In the main function, call the find_minute function and display the result. Pointer arithmetic is NOT required in the main function. Examples (your program must follow this format precisely) Example #1 Enter the number of interesting minutes: 3 Enter the interesting minutes: 7 13 28 Output: 20 Note: In the first example, minutes 14, 15, 16, 17, 18, 19, 20 are all boring and thus an average viewer will stop watching after the 20th minute. So, the average viewer will watch for 20 minutes. Example #2 Enter the number of interesting minutes: 3 Enter the interesting minutes: 7 13 18 Output: 25 Note: In the second example, minutes 19, 20, 21, 22, 23, 24, 25 are all boring and thus an average viewer will stop watching after the 25th minute. So, the average viewer will watch for 25 minutes. Example #3 Enter the number of interesting minutes: 6 Enter the interesting minutes: 9 13 28 32 38 42 Output: 7 Note: In the third example, minutes 1, 2, 3, 4, 5, 6, 7 are all boring and thus an average viewer will stop watching after the 7th minute. So, the average viewer will watch for 7 minutes. Example #4 Enter the number of interesting minutes: 6 Enter the interesting minutes: 5 12 18 24 31 38 Output: 45 Note: In the fourth example, there are no consecutive 7 boring minutes So, the average viewer will watch for the whole 45 minutes.
Task - Using pointers to process arrays (C Languaage)
In a TV show, each minute can be either interesting or boring. Assume that if 7 consecutive minutes are boring, then an average viewer will stop watching the show. Write a C program that calculates how many minutes that an average viewer will watch a TV show, given the interesting minutes. Assume the TV shows are 45 minutes long.
Requirements
- Name your program project4_minutes.c.
- Follow the format of the examples below.
- The program will read in the number of interesting minutes, then read in the interesting minutes.
- The program should include the following function. Do not modify the function prototype.
int find_minute(int *minutes, int n);
-
- minutes represents the input array for interesting minutes, n is the length of the array (the number of interesting minutes). The function returns the how many minutes an average viewer will watch the show.
- This function should use pointer arithmetic– not subscripting – to visit array elements. In other words, eliminate the loop index variables and all use of the [] operator in the function.
- In the main function, call the find_minute function and display the result.
- Pointer arithmetic is NOT required in the main function.
Examples (your program must follow this format precisely)
Example #1
Enter the number of interesting minutes: 3
Enter the interesting minutes: 7 13 28
Output: 20
Note: In the first example, minutes 14, 15, 16, 17, 18, 19, 20 are all boring and thus an average viewer will stop watching after the 20th minute. So, the average viewer will watch for 20 minutes.
Example #2
Enter the number of interesting minutes: 3
Enter the interesting minutes: 7 13 18
Output: 25
Note: In the second example, minutes 19, 20, 21, 22, 23, 24, 25 are all boring and thus an average viewer will stop watching after the 25th minute. So, the average viewer will watch for 25 minutes.
Example #3
Enter the number of interesting minutes: 6
Enter the interesting minutes: 9 13 28 32 38 42
Output: 7
Note: In the third example, minutes 1, 2, 3, 4, 5, 6, 7 are all boring and thus an average viewer will stop watching after the 7th minute. So, the average viewer will watch for 7 minutes.
Example #4
Enter the number of interesting minutes: 6
Enter the interesting minutes: 5 12 18 24 31 38
Output: 45
Note: In the fourth example, there are no consecutive 7 boring minutes So, the average viewer will watch for the whole 45 minutes.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

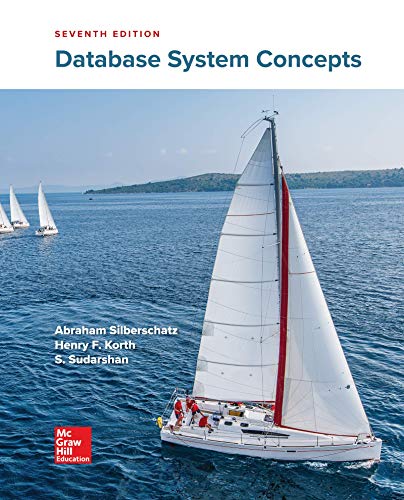
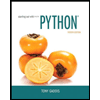
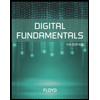
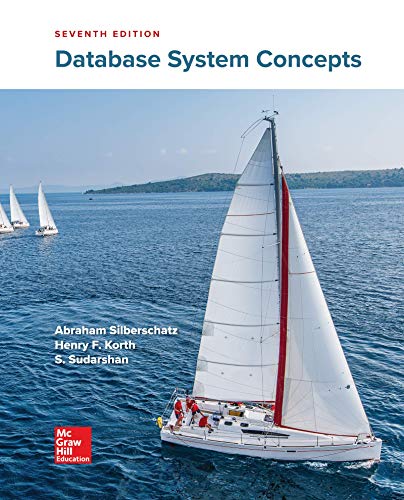
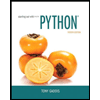
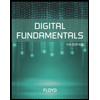
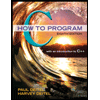
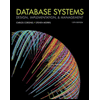
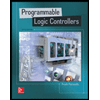