1) make a sierpenski triangle using java and recursion. Make it similar to the code below. And please comment the code
please help me with the following
1) make a sierpenski triangle using java and recursion. Make it similar to the code below. And please comment the code
2) Please make the follow code out put on the console. It a t square fractal
import java.awt.image.*;
import java.awt.Color;
import java.io.*;
import javax.imageio.*;
public class Main
{
static final int DIMENSION = 1000;
static BufferedImage image = new BufferedImage(DIMENSION, DIMENSION, BufferedImage.TYPE_INT_RGB);
static final int WHITE = Color.WHITE.getRGB();
static final int BLACK = Color.BLACK.getRGB();
private static void drawSquare(int x, int y, int side)
{
if (side <= 0)
return;
else
{
int left = x - side/2;
int top = y - side/2;
int right = x + side/2;
int bottom = y + side/2;
for (int i = left; i < right; i++)
for (int j = top; j < bottom; j++)
{
image.setRGB(i, j, BLACK);
}
drawSquare(left, top, side/2);
drawSquare(left, bottom, side/2);
drawSquare(right, top, side/2);
drawSquare(right, bottom, side/2);
}
}
public static void main (String[] args) throws IOException
{
for (int i = 0; i < DIMENSION; i++)
for (int j = 0; j < DIMENSION; j++)
{
image.setRGB(i, j, WHITE);
}
drawSquare(DIMENSION/2, DIMENSION/2, DIMENSION/2);
File imagefile = new File("tfractal.jpg");
ImageIO.write(image, "jpg", imagefile);
}
}

Step by step
Solved in 3 steps with 2 images

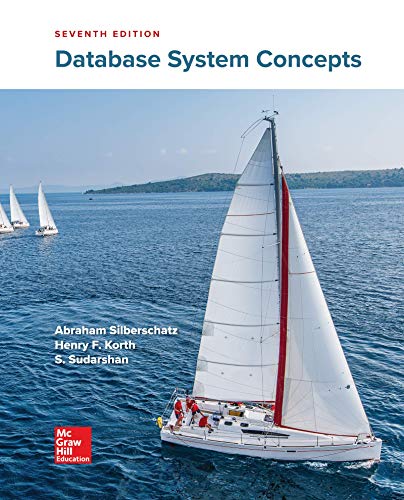
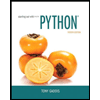
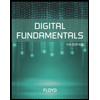
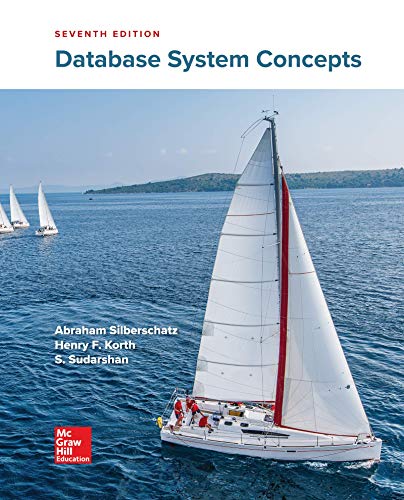
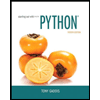
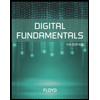
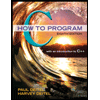
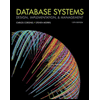
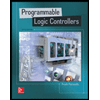