Can you help me with my code? It is only giving me one error saying my "identifier: binary_search is unidentified", and I can't figure out how to fix it. #include #include #include using namespace std; template class myVector : public vector { bool sorted = false; // static member to remember if the list is sorted public: int seqSearch(T searchItem); int binarySearch(T searchItem); void bubbleSort(); void insertionSort(); }; int main() { // Define test vector myVector nameList; // Add values to the vector nameList.push_back("Alice"); nameList.push_back("Bob"); nameList.push_back("Eve"); nameList.push_back("Charlie"); nameList.push_back("Dave"); // Test sort methods nameList.bubbleSort(); nameList.insertionSort(); // Test search methods int index = nameList.seqSearch("Charlie"); cout << "Charlie was found at index: " << index << endl; index = nameList.binarySearch("Charlie"); cout << "Charlie was found at index: " << index << endl; // Print sorted vector using range based for loop cout << "Sorted vector: "; for (const auto& name : nameList) { cout << name << " "; } cout << endl; // Define new test vector myVector intList; // Define an iterator to the vector auto it = intList.begin(); // Add values to the vector intList.insert(it, 1); intList.insert(it, 3); intList.insert(it, 2); intList.insert(it, 5); intList.insert(it, 4);
Can you help me with my code? It is only giving me one error saying my "identifier: binary_search is unidentified", and I can't figure out how to fix it.
#include <iostream>
#include <string>
#include <vector>
using namespace std;
template <class T>
class myVector : public vector<T> {
bool sorted = false; // static member to remember if the list is sorted
public:
int seqSearch(T searchItem);
int binarySearch(T searchItem);
void bubbleSort();
void insertionSort();
};
int main() {
// Define test vector
myVector<string> nameList;
// Add values to the vector
nameList.push_back("Alice");
nameList.push_back("Bob");
nameList.push_back("Eve");
nameList.push_back("Charlie");
nameList.push_back("Dave");
// Test sort methods
nameList.bubbleSort();
nameList.insertionSort();
// Test search methods
int index = nameList.seqSearch("Charlie");
cout << "Charlie was found at index: " << index << endl;
index = nameList.binarySearch("Charlie");
cout << "Charlie was found at index: " << index << endl;
// Print sorted vector using range based for loop
cout << "Sorted vector: ";
for (const auto& name : nameList) {
cout << name << " ";
}
cout << endl;
// Define new test vector
myVector<int> intList;
// Define an iterator to the vector
auto it = intList.begin();
// Add values to the vector
intList.insert(it, 1);
intList.insert(it, 3);
intList.insert(it, 2);
intList.insert(it, 5);
intList.insert(it, 4);
// Test the STL binary_search algorithm
bool found = binary_search(intList.begin(), intList.end(), 3);
if (found) {
cout << "3 was found in the vector." << endl;
}
else {
cout << "3 was not found in the vector." << endl;
}
// Print the resulting vector using an iterator
cout << "Sorted vector: ";
for (it = intList.begin(); it != intList.end(); it++) {
cout << *it << " ";
}
cout << endl;
return 0;
}
template <class T>
int myVector<T>::seqSearch(T searchItem) {
// Sequential search implementation
for (int i = 0; i < this->size(); i++) {
if (this->at(i) == searchItem) {
return i; // return index of found item
}
}
return -1; // return -1 if item not found
}
template <class T>
void myVector<T>::bubbleSort() {
// Bubble sort implementation
bool swapped = true;
while (swapped) {
swapped = false;
for (int i = 0; i < this->size() - 1; i++) {
if (this->at(i) > this->at(i + 1)) {
// Swap values
T temp = this->at(i);
this->at(i) = this->at(i + 1);
this->at(i + 1) = temp;
swapped = true;
}
}
}
sorted = true; // set sorted to true after sorting
}
template <class T>
void myVector<T>::insertionSort() {
// Insertion sort implementation
for (int i = 1; i < this->size(); i++) {
T value = this->at(i);
int j = i - 1;
while (j >= 0 && this->at(j) > value) {
this->at(j + 1) = this->at(j);
j--;
}
this->at(j + 1) = value;
}
sorted = true; // set sorted to true after sorting
}
template <class T>
int myVector<T>::binarySearch(T searchItem) {
// Binary search implementation
if (!sorted) { // check if list is sorted
bubbleSort(); // sort list if not sorted
}
int low = 0;
int high = this->size() - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (this->at(mid) == searchItem) {
return mid; // return index of found item
}
else if (this->at(mid) > searchItem) {
high = mid - 1;
}
else {
low = mid + 1;
}
}
return -1; // return -1 if item not found
}
int main() {
// Define test vector
myVector<string> nameList;
// Add values to the vector
nameList.push_back("Alice");
nameList.push_back("Bob");
nameList.push_back("Eve");
nameList.push_back("Charlie");
nameList.push_back("Dave");
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

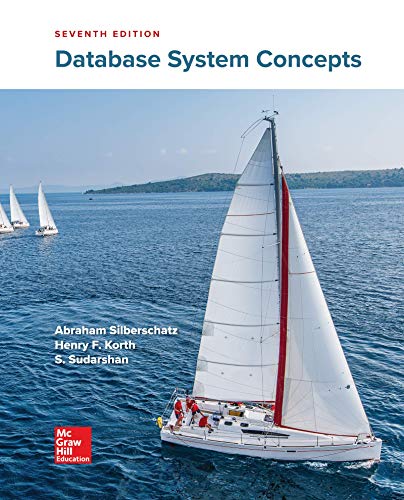
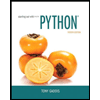
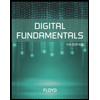
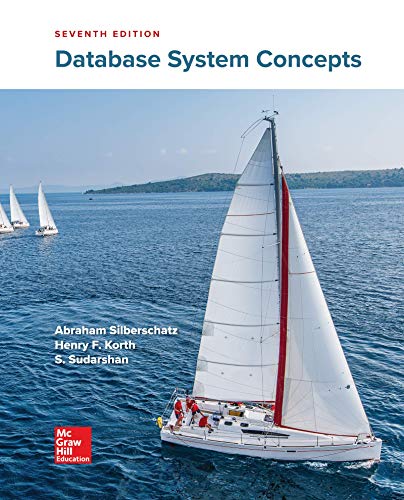
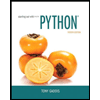
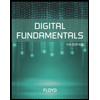
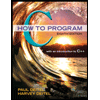
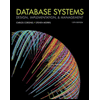
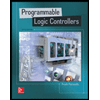