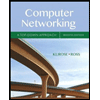
JAVA Task, by using ArrayList
The program reads manatee names from the standard input stream and from time to time prints a sorted portion of the list of names. All the manatee names in the input are given in the order in which the necropsy was performed. You can imagine a unique timestamp associated with each necropsy. The names are not necessarily unique (many of the manatees have the same name). Interspered in the standard input stream are queries that begin with the question mark character. Queries ask for an alphabetical list of manatee names to be printed to the standard output stream. The list depends only on the names upto that point in the input stream and does include any manatee names that appear in the input stream after the query.
The list does not contain all the names of the previous necropsies, but only a selection of them. A query provides the names of two manatees, say A and B. The response to the query drops necropsies performed before the first named A and all the necropsies performed after the last one named B. In the response, the names are to be in alphabetical order and are to be numbered 1, 2, and so on in the standard output stream.
In alphabetical or dictionary order (unlike lexicographic order), case of a letter is considered only after the letter itself: b < B < c; and diacritics are likewise secondardy characteristics: u < ü < v
Read all input from the standard input stream. Write all output to the standard output stream. Manatee names do not contain white space. For each query a numbered list is printed, unless one or the other or both manatee are not found in the previous input. In which case, print the line "Not found".
In the case that both names are found, but the reponse is empty, print the line "Empty list".
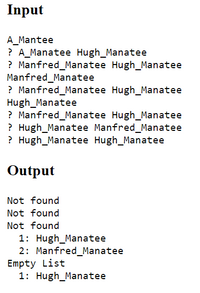

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- /*TASK 5. Implement part 1 of the report. You need to read data from input.txt file, store it into array of items (called items) and write it in a formatted form to the output file. Code, debug and present it in the best possible format. Hint: It might be helpful to format the String coming from toString( ) method using String.format( ) method, which works similar to System.out.prinf. Hint2: You might have to close and delete existing output .doc or .txt file from its folder * public class YourUserName_hw7 { static Item [ ] items = new Item [200];//an array of Items static EdibleItem [ ] edibleItems;//an array of Edible Items static int countEdibleitems = 0;//count edible items static String pageHeader; //save the header //main( ) method runs the program public static void main(String[ ] args) throws FileNotFoundException { double sum = 0, average = 0; //Optional - create a new input file //CreateInput.createInputFile( );…arrow_forwardUsing Python This project assumes that you have completed Project 1. Place several Student objects into a list and shuffle it. Then run the sort method with this list and display all of the students’ information. Print to the console the unsorted list first of all students followed by the sorted list of all students Note: The sorted list should output in the following format: Sorted list of students: Name: Name1 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name2 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name3 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name4 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name5 Scores: 0 0 0 0 0 0 0 0 Hint: Use the print function with a Student object as an argument to print the Student object in the format specified above. Use sort list method to sort list utput unsorted and sorted list to the terminal Make sure to display the unsorted student information first and follow the template shown in the instructions. If the output format does not match the template provided, the test may…arrow_forwardString replacement is read from input. Then, sentence is read from input. If replacement is in sentence, then: Output 'Located at index: ' followed by the index of the last occurrence. Create a string from sentence with the first two occurrences of replacement replaced by '5' and output sentence on a newline.arrow_forward
- In this lab, we will practice the use of arrays. We will write a program that follows the steps below: Input 6 numbers from the user user inputs numbers one at a time Loop continuously until the exit condition below: ask the user to search for a query number if the query number is in the list: reports the first location of the query number in the list, change the number in that position to 0, show the updated list if the query number is not in the list: exit the loop You must write at least one new function: findAndReplace(array1,...,query) – take in an array and query number as input (in addition to any other needed inputs); it will return the first location of the query number in the list (or -1 if it is not in the list) and will change the number at that location to 0. You may receive the number inputs and print all outputs in int main if you wish. You may report location based on 0-indexing or 1-indexing (but you need 0-indexing to access the array elements!).arrow_forwardGrey lines (start - 20 & 22-end of code) can NOT be edited. New JAVA code must be in between theses as show on line 21 in image.arrow_forwardWrite a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements: • Each row contains the title, rating, and all showtimes of a unique movie. • A space is placed before and after each vertical separator (I) in each row. • Column 1 displays the movie titles and is left justified with a minimum of 44 characters. . If the movie title has more than 44 characters, output the first 44 characters only. • Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. • Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the CSV file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Ex: If the input of the program is: movies.csv and the contents of…arrow_forward
- The function interleave_lists in python takes two parameters, L1 and L2, both lists. Notice that the lists may have different lengths. The function accumulates a new list by appending alternating items from L1 and L2 until one list has been exhausted. The remaining items from the other list are then appended to the end of the new list, and the new list is returned. For example, if L1 = ["hop", "skip", "jump", "rest"] and L2 = ["up", "down"], then the function would return the list: ["hop", "up", "skip", "down", "jump", "rest"]. HINT: Python has a built-in function min() which is helpful here. Initialize accumulator variable newlist to be an empty list Set min_length = min(len(L1), len(L2)), the smaller of the two list lengths Use a for loop to iterate k over range(min_length) to do the first part of this function's work. On each iteration, append to newlist the item from index k in L1, and then append the item from index k in L2 (two appends on each iteration). AFTER the loop…arrow_forwardIn c++ and without the use of vectors, please. Thanks very much! A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3…arrow_forward1. The codewords will be scrambled words. You will read the words used for the codes from a file and store it into an array. There are 60 words in the file that range in size from 3 characters to 7 characters. The file is called wordlist.txt and can be found attached to the assignment in IvyLearn.2. Start with input for an integer seed for the random number generator. There is no need to put a prompt before the cin operation.3. Ask the Player if they are ready to play and only proceed if they type a Y or y. If the user types an N or n then the program should finish. a. INPUT VALIDATION: Make sure the user has typed either y, Y, n, or N4. Create a variable to keep track of the number of guesses the user has made.5. Use the random number generator to pick a word from the list of words.6. Once you have chosen a word as the codeword you will need to scramble the letters to make it into a code. You should use the random number generator to help you mix up the letters.7. Display to the user…arrow_forward
- Please explain the stepsarrow_forwardIn c++ and please without the use of vectors. Thanks very much! A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use linear search. Modify the algorithm to output the count of how many comparisons were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Joe 123-5432 Linda 983-4123…arrow_forwardComputer Science Using Java, write a simple Insertion Sort program that can read in integers from a text file (line by line) and sort them into another text file. Use inFile and outFile for the input and output files, respectively. Also make sure that the algorithm keeps track of the comparisons and exchanges performed by the sort so that they may be printed out in the console after the sort is completedarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
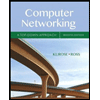
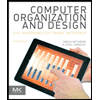
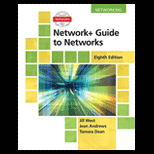
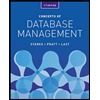
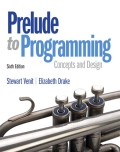
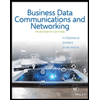