This project assumes that you have completed Project 1. Place several Student objects into a list and shuffle it. Then run the sort method with this list and display all of the students’ information. Print to the console the unsorted list first of all students followed by the sorted list of all students Note: The sorted list should output in the following format: Sorted list of students: Name: Name1 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name2 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name3 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name4 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name5 Scores: 0 0 0 0 0 0 0 0 0 0 code: # import random to use random shuffle function. import random # import random to use random shuffle function. import random class Student(object): """Represents a student.""" def __init__(self, name, number): """All scores are initially 0.""" self.name = name self.scores = [] for count in range(number): self.scores.append(0) def getName(self): """Returns the student's name.""" return self.name def setScore(self, i, score): """Resets the ith score, counting from 1.""" self.scores[i - 1] = score def getScore(self, i): """Returns the ith score, counting from 1.""" return self.scores[i - 1] def getAverage(self): """Returns the average score.""" return sum(self.scores) / len(self._scores) def getHighScore(self): """Returns the highest score.""" return max(self.scores) def __str__(self): """Returns the string representation of the student.""" return "Name: " + self.name + "\nScores: " + \ " ".join(map(str, self.scores)) def __lt__(self, other): """Returns self < other, with respect to names.""" return self.name < other.name def __ge__(self, other): """Returns self >= other, with respect to names.""" return self.name >= other.name def __eq__(self, other): """Tests for equality.""" if self is other: return True elif type(self) != type(other): return False else: return self.name == other.name def main(): """Tests sorting.""" # Create the list and put 5 students into it lyst = [] for count in reversed(range(5)): s = Student("Name" + str(count + 1), 10) lyst.append(s) # Complete the definition of the main function print("Sorted list of students:") # Shuffle the list using the import statement random random.shuffle(lyst) # Sort the list using the sort() method. lyst.sort() # Disply the student list data. for obj in lyst: print(obj.__str__()) if __name__ == "__main__": main() Make sure to display the unsorted student information first and follow the template shown in the instructions. If the output format does not match the template provided, the test may return incorrect results for your program.
This project assumes that you have completed Project 1. Place several Student objects into a list and shuffle it. Then run the sort method with this list and display all of the students’ information. Print to the console the unsorted list first of all students followed by the sorted list of all students Note: The sorted list should output in the following format: Sorted list of students: Name: Name1 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name2 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name3 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name4 Scores: 0 0 0 0 0 0 0 0 0 0 Name: Name5 Scores: 0 0 0 0 0 0 0 0 0 0 code: # import random to use random shuffle function. import random # import random to use random shuffle function. import random class Student(object): """Represents a student.""" def __init__(self, name, number): """All scores are initially 0.""" self.name = name self.scores = [] for count in range(number): self.scores.append(0) def getName(self): """Returns the student's name.""" return self.name def setScore(self, i, score): """Resets the ith score, counting from 1.""" self.scores[i - 1] = score def getScore(self, i): """Returns the ith score, counting from 1.""" return self.scores[i - 1] def getAverage(self): """Returns the average score.""" return sum(self.scores) / len(self._scores) def getHighScore(self): """Returns the highest score.""" return max(self.scores) def __str__(self): """Returns the string representation of the student.""" return "Name: " + self.name + "\nScores: " + \ " ".join(map(str, self.scores)) def __lt__(self, other): """Returns self < other, with respect to names.""" return self.name < other.name def __ge__(self, other): """Returns self >= other, with respect to names.""" return self.name >= other.name def __eq__(self, other): """Tests for equality.""" if self is other: return True elif type(self) != type(other): return False else: return self.name == other.name def main(): """Tests sorting.""" # Create the list and put 5 students into it lyst = [] for count in reversed(range(5)): s = Student("Name" + str(count + 1), 10) lyst.append(s) # Complete the definition of the main function print("Sorted list of students:") # Shuffle the list using the import statement random random.shuffle(lyst) # Sort the list using the sort() method. lyst.sort() # Disply the student list data. for obj in lyst: print(obj.__str__()) if __name__ == "__main__": main() Make sure to display the unsorted student information first and follow the template shown in the instructions. If the output format does not match the template provided, the test may return incorrect results for your program.
Chapter8: Arrays
Section: Chapter Questions
Problem 9PE
Related questions
Question
This project assumes that you have completed Project 1. Place several Student objects into a list and shuffle it. Then run the sort method with this list and display all of the students’ information. Print to the console the unsorted list first of all students followed by the sorted list of all students
Note: The sorted list should output in the following format:
Sorted list of students:
Name: Name1
Scores: 0 0 0 0 0 0 0 0 0 0
Name: Name2
Scores: 0 0 0 0 0 0 0 0 0 0
Name: Name3
Scores: 0 0 0 0 0 0 0 0 0 0
Name: Name4
Scores: 0 0 0 0 0 0 0 0 0 0
Name: Name5
Scores: 0 0 0 0 0 0 0 0 0 0
code:
# import random to use random shuffle function.
import random
# import random to use random shuffle function.
import random
class Student(object):
"""Represents a student."""
def __init__(self, name, number):
"""All scores are initially 0."""
self.name = name
self.scores = []
for count in range(number):
self.scores.append(0)
def getName(self):
"""Returns the student's name."""
return self.name
def setScore(self, i, score):
"""Resets the ith score, counting from 1."""
self.scores[i - 1] = score
def getScore(self, i):
"""Returns the ith score, counting from 1."""
return self.scores[i - 1]
def getAverage(self):
"""Returns the average score."""
return sum(self.scores) / len(self._scores)
def getHighScore(self):
"""Returns the highest score."""
return max(self.scores)
def __str__(self):
"""Returns the string representation of the student."""
return "Name: " + self.name + "\nScores: " + \
" ".join(map(str, self.scores))
def __lt__(self, other):
"""Returns self < other, with respect
to names."""
return self.name < other.name
def __ge__(self, other):
"""Returns self >= other, with respect
to names."""
return self.name >= other.name
def __eq__(self, other):
"""Tests for equality."""
if self is other:
return True
elif type(self) != type(other):
return False
else:
return self.name == other.name
def main():
"""Tests sorting."""
# Create the list and put 5 students into it
lyst = []
for count in reversed(range(5)):
s = Student("Name" + str(count + 1), 10)
lyst.append(s)
# Complete the definition of the main function
print("Sorted list of students:")
# Shuffle the list using the import statement random
random.shuffle(lyst)
# Sort the list using the sort() method.
lyst.sort()
# Disply the student list data.
for obj in lyst:
print(obj.__str__())
if __name__ == "__main__":
main()
Make sure to display the unsorted student information first and follow the template shown in the instructions. If the output format does not match the template provided, the test may return incorrect results for your program.Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
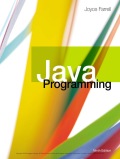
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
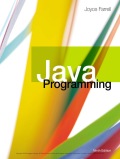
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT