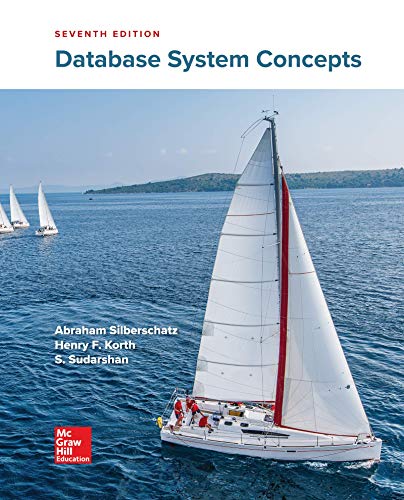
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
using java
Complete the attached assignment.
![### Java Program to Display Airline Seat Layout
This Java program is designed to display the layout of seats in an airplane using a two-dimensional array. Below is the transcribed code and an explanation of its components.
```java
public class AirlineDriver {
public static void main (String[] args) {
char [][] layout = { {'A', 'B', 'C', 'D'},
{'A', 'B', 'C', 'D'},
{'A', 'B', 'C', 'D'},
{'A', 'B', 'C', 'D'},
{'A', 'B', 'C', 'D'}};
displaySeats(layout);
}
public static void displaySeats(char [][] plane) {
for (int row = 0; row < plane.length; row++) {
System.out.print(" " + (row + 1) + " ");
// Add for loop to display one row
// Display row
System.out.println();
}
}
}
```
### Explanation
1. **Class Declaration:**
- `public class AirlineDriver`: This is the main class named `AirlineDriver`.
2. **Main Method:**
- `public static void main (String[] args)`: This method is the entry point of any Java application. It initializes a two-dimensional char array named `layout` which represents the seat arrangement.
3. **Seat Layout Array:**
- The `layout` array is a 5x4 grid, where each inner array represents a row of seats 'A', 'B', 'C', and 'D'.
4. **Display Function:**
- `public static void displaySeats(char [][] plane)`: This method takes a 2D char array as an argument and prints the seat layout.
- A `for` loop iterates over each row, printing the row number. The method suggests adding code inside the loop to display the actual seat characters.
5. **Potential Enhancements:**
- Complete the inner loop to print each character in a row.
- Format the output for better readability.
This code is a starting point for understanding how to handle 2D arrays in Java, particularly for applications such as managing seating arrangements in transportation systems.](https://content.bartleby.com/qna-images/question/ff17d012-70ad-4782-80ac-5077bdfc24e1/0997538a-df08-45b3-abb6-822716b0f9ec/mgjksr8_thumbnail.png)
Transcribed Image Text:### Java Program to Display Airline Seat Layout
This Java program is designed to display the layout of seats in an airplane using a two-dimensional array. Below is the transcribed code and an explanation of its components.
```java
public class AirlineDriver {
public static void main (String[] args) {
char [][] layout = { {'A', 'B', 'C', 'D'},
{'A', 'B', 'C', 'D'},
{'A', 'B', 'C', 'D'},
{'A', 'B', 'C', 'D'},
{'A', 'B', 'C', 'D'}};
displaySeats(layout);
}
public static void displaySeats(char [][] plane) {
for (int row = 0; row < plane.length; row++) {
System.out.print(" " + (row + 1) + " ");
// Add for loop to display one row
// Display row
System.out.println();
}
}
}
```
### Explanation
1. **Class Declaration:**
- `public class AirlineDriver`: This is the main class named `AirlineDriver`.
2. **Main Method:**
- `public static void main (String[] args)`: This method is the entry point of any Java application. It initializes a two-dimensional char array named `layout` which represents the seat arrangement.
3. **Seat Layout Array:**
- The `layout` array is a 5x4 grid, where each inner array represents a row of seats 'A', 'B', 'C', and 'D'.
4. **Display Function:**
- `public static void displaySeats(char [][] plane)`: This method takes a 2D char array as an argument and prints the seat layout.
- A `for` loop iterates over each row, printing the row number. The method suggests adding code inside the loop to display the actual seat characters.
5. **Potential Enhancements:**
- Complete the inner loop to print each character in a row.
- Format the output for better readability.
This code is a starting point for understanding how to handle 2D arrays in Java, particularly for applications such as managing seating arrangements in transportation systems.
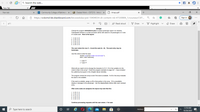
Transcribed Image Text:**Educational Content: Assigning Passenger Seats in an Airplane**
**Arrays in Java Programming**
This exercise involves modifying the `AirlineDriver.java` program to assign passenger seats on a small commuter airline with a seating capacity of 20 passengers. The seating is organized into 5 rows of 4 seats each, as shown below:
```
1 A B C D
2 A B C D
3 A B C D
4 A B C D
5 A B C D
```
### User Input for Seat Selection
- **Instructions:** The user will input the seat selection by specifying the row number (1–5) and the seat letter (A–D). Note: The seat letter input can be in lowercase.
To capture the seat selection from the user, use the following code snippet:
```java
System.out.print("Enter row and seat ");
seat = scan.nextLine();
r = seat.??; // Extract and assign row number
c = seat.??; // Extract and assign seat letter
```
### Converting Seat Input to Array Indices
- **Objective:** Convert the seat letter (A, B, C, or D) into the corresponding column index in the array. Refer to the "LetterCount" program example in Chapter Seven Activities for guidance.
### Seat Assignment Logic
The program checks the availability of a seat by scanning the array. An 'X' in the array indicates the seat is not available.
- **Logic for Seat Availability:**
- If the seat is available, assign 'X' at that position in the array.
- If unavailable, notify the passenger and request a different selection using the `displaySeats` method after each entry.
### Example Layout After Seat Assignments
Once some seats have been assigned, the seating arrangement might resemble:
```
1 A B C X
2 A B C D
3 A B C X
4 A B C D
5 A B C D
```
**Note:** Continue processing seat requests until the user enters "1" for the seat.
This programming exercise helps reinforce understanding of arrays, user input handling, and control structures in Java.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Assume you are writing code to maintain a linked list. You are to usea variable named head to always point to the first node in the list and the variable named tail to always point to the last node in the list. Write the Java statements required to accomplish each of the following actions given the Node definition above. For the following questions, provide both the lines of code to accomplish the required task (given the restrictions above), and a diagram showing the state of the linked list after all your lines of code (in each question) have been executed. (a) Create a linked list with just a single node that has the value 5 in it. (b) Create and append a node with the value 10 to the end of the list in 1aabove.(c) Using a loop construct of your choice, provide the lines of code requiredto add nodes with the values 15, 20, 25, 30, 35 and 40 in that order to the end of the list in 1b above. (d) Add a node with the value 12 to the list in 1c in its proper positionassuming the list…arrow_forwardFor this assignment, you must implement the ElGamal Public-Key Encryption scheme with any programming language that you are comfortable with. The recommendation is to use C/C++ or Java. You can accomplish this assignment individually or by forming a team of 2 students. Alternatively, you can work with your team members of the previous assignment. Your program should get the "key size" from the user in terms of number of bits (for instance, 64, 128, etc). Based on the size, use Fermat or Miller-Rabin primality test algorithm to define your large prime number. You can use the existing programming packages to find a generator of your finite field. Your program should then initialize a pair of key, i.e., private and public keys. For the computations, utilize the Square-and Multiply algorithm. In fact, your program should get a plaintext from the user to perform encryption (generating the ciphertext from the plaintext) and decryption (generating the original plaintext from the ciphertext).…arrow_forwardDesign ADT for a cave and a cave system. An archeologist should be able to add a newly discovered cave to a cave system and to connect two caves together by a tunnel. Duplicate caves—based on GPS coordinates—are not permitted. Archeologists should also be able to list the caves in a given cave system. Specify each ADT operation by stating its purpose, describing its parameters, and writing a pseudocode version of its header. Then Java interface for a cave's methods and one for the methods of a cave system. Include javadoc-style comments in your code. Java programarrow_forward
- In C, what does the malloc() method do? Group of answer choices Dynamically allocates a block of memory with the specified size. Releases a block of memory with the specified size. Reconfigures a block of memory with the specified size. Determines the amount of memory used by a given structure variablearrow_forwardDevelop an algorithm and write a C++ program that counts the letter occurrence in the string; make sure you use call by reference to the array of a class with letter and count as private member attributes when return from parse function. For the class member methods, provide the public accessor and modifier as well as print functions for the private member attributes. Write a test program that reads a C-string and displays the number of letters [a-z] and number of numbers [0-9] in the string. In addition to that, you need to output the histogram of the letter and count array. Here is a sample run of the program: <Output> Enter a string: 2023 is coming The number of letters in 2023 is coming is 8 The number of numbers in 2023 is coming is 4 ---- Histogram ---- Char Count 0 1 2 2 3 1 c 1 g 1 i 2 m 1 n 1 /* not a completed output */ <End Output>arrow_forwarda java assignment about 4 used defined exception using array loop based on real life scenario. (use only java)arrow_forward
- Assignment-3 With the help of a C program show how to typecast a void * to an int * . Attach the code and output and upload the file on classroom.arrow_forwardC++ Attached is the questionarrow_forwardPlease answer in C++ and give explanation LAB 17.1-B Linked List Read the internal documentation for class ItemList carefully. Fill in the missing code in the implementation file, being careful to adhere to the preconditions and postconditions. Compile your program. ------------------------------------------------------------------------------------------------------------------------------- class ItemList { private: struct ListNode { int value; ListNode * next; }; ListNode * head; public: ItemList(); // Post: List is the empty list. bool IsThere(int item) const; // Post: If item is in the list IsThere is // True; False, otherwise. void Insert(int item); // Pre: item is not already in the list. // Post: item is in the list. void Delete(int item); // Pre: item is in the list. // Post: item is no…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
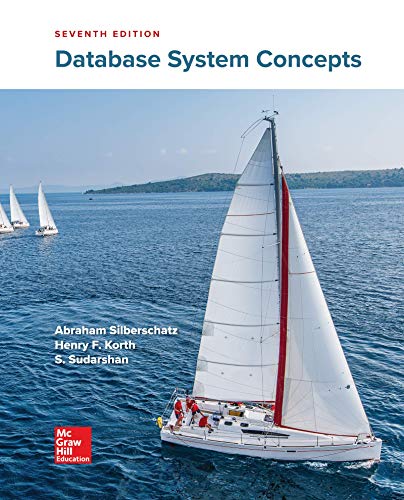
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
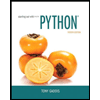
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
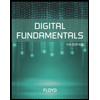
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
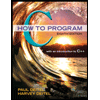
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
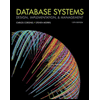
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
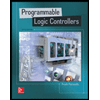
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education