Write a code in C# that finds the smallest ID of a subject taught by the most teachers without using any Linq Lib's elements, such as OrderBy, OrderByDescending, etc: We know about the teachers at a school, what lessons they have and when. We identify the teachers, the subjects, the days of the week and the time slots in a day with ID numbers. Write a program that determines the subject that is taught by the most teachers. Input The first line of the standard input contains the count of lessons (1≤L≤800), the count of teachers (1≤N≤100), the count of subjects (1≤M≤100), the ID of a teacher (1≤T≤N) and the ID of a day (1≤D≤5), separated by spaces. The next L lines contain 4 integers separated by spaces: the ID of the teacher (1≤TID≤N), the ID of subject taught (1≤TS≤M), day (1≤Day≤5), and time slot (0≤Time≤8) of a lesson. For example, 3 7 2 1 means that the third teacher teaches the seventh subject on the second day of the week in the first time slot. Output The first line of the standard output should contain the ID of the subject that is taught by the most teachers. If there is more than one solution, give the smallest ID. Example: Input 8 3 4 1 1 1 1 1 6 1 1 2 2 1 2 1 3 2 1 2 2 2 2 3 1 3 4 1 2 3 2 1 4 3 3 2 1 Output: 2
Write a code in C# that finds the smallest ID of a subject taught by the most teachers without using any Linq Lib's elements, such as OrderBy, OrderByDescending, etc:
We know about the teachers at a school, what lessons they have and when. We identify the teachers, the subjects, the days of the week and the time slots in a day with ID numbers. Write a program that determines the subject that is taught by the most teachers.
Input
The first line of the standard input contains the count of lessons (1≤L≤800), the count of teachers (1≤N≤100), the count of subjects (1≤M≤100), the ID of a teacher (1≤T≤N) and the ID of a day (1≤D≤5), separated by spaces. The next L lines contain 4 integers separated by spaces: the ID of the teacher (1≤TID≤N), the ID of subject taught (1≤TS≤M), day (1≤Day≤5), and time slot (0≤Time≤8) of a lesson. For example, 3 7 2 1 means that the third teacher teaches the seventh subject on the second day of the week in the first time slot.
Output
The first line of the standard output should contain the ID of the subject that is taught by the most teachers. If there is more than one solution, give the smallest ID.
Example:
Input
8 3 4 1 1
1 1 1 6
1 1 2 2
1 2 1 3
2 1 2 2
2 2 3 1
3 4 1 2
3 2 1 4
3 3 2 1
Output:
2

Step by step
Solved in 4 steps with 3 images

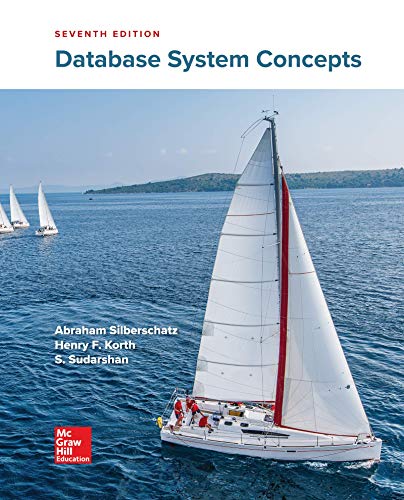
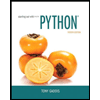
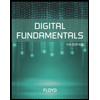
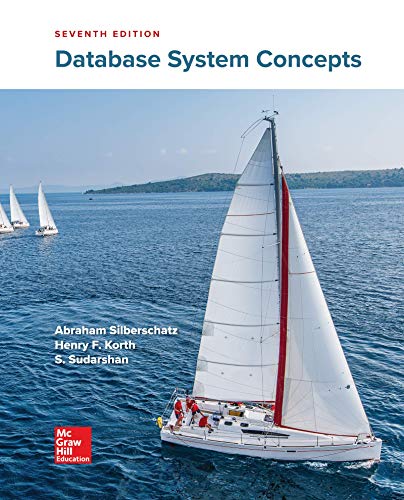
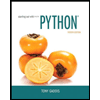
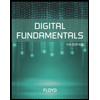
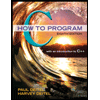
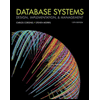
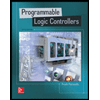