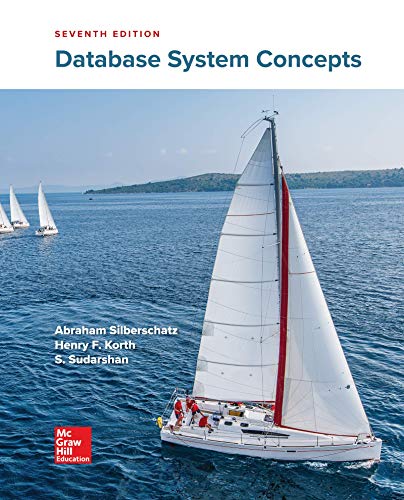
Answer the given question with a proper explanation and step-by-step solution.
Below is your function add(t) from 6.4 which adds all items in the tuple t and returns the result. This function is unprotected and will fail whenever the tuple t contains an item that is not a number. Run the
Expected output:
Tuple of values: (1, 'two', 3)
unsupported operand type(s) for +=: 'int' and 'str'
Sum of the values: None
def add(t):
"""Adds all values in the tuple t"""
result = 0
for x in t:
result += x
return result
# Main program (do not change):
values = (1, 'two', 3)
print("Tuple of values:", values)
print("Sum of the values:", add(values))

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Implement function prt_triangle that takes an integer n from the user and prints a n-row triangle using asterisk. It first prompts the message "Enter the size of the triangle:", takes a number from the user as input, and then prints the triangle. You should use the function printf to display the message, allocate memory for one null-terminated strings of length up to 2 characters using char rows[3], and then use the function fgets to read the input into the strings, e.g. fgets(rows, sizeof(rows), stdin). You also need to declare one integer variable nrows using int nrows, and use the function atoi to convert the string rows into the integer nrows. You can use the command man atoi to find more information on how to use the atoi function. A sample execution of the function is as below:Enter the size of the triangle: 5 * ** ** * make sure it is in C not C++arrow_forwardpython help So, we will try this one from scratch. Implement the function postalLabel() that takes 6 inputs: a first name (as a string) a last name (as a string) an address (as a string) a city (as a string) a state (as a string) a zip code (as an integer) The user will be prompted to enter the six inputs. As noted, the inputs should be entered as strings except for the zip code. Use the string format method to print the label (no other will be accepted). You must provide the appropriate docstring and the comments for every statement. If this information is missing, you will lose points. Your result should look like the example below except it should be your name with other bogus information (do not put in your address, city and state – make them up). This program should consist of input prompts and a string formatted print stamen.>>> postalLabel() >>> Please enter your first name: Parker >>> Please enter your last name: Jones >>> Please enter…arrow_forwardMust show it in Python: Please show step by step with comments. Please show it in simplest form. Input and Output must match with the Question Please go through the Question very carefully.arrow_forward
- JS In this challenge, you must verify the equality of two different values given the parameters a and b. Both the value and type of the parameters need to be equal. The possible types of the given parameters are: What have you learned so far that will permit you to do two different checks (value and type) with a single statement? Implement a function that returns true if the parameters are equal, and false if they are not. Examples checkEquality(1, true) → false // A number and a boolean: the value and type are different. checkEquality(0, "0") → false // A number and a string: the type is different.arrow_forwardWrite a function verifsort that accepts a list as a parameter, check if the list elements are sorted and returns: 1 - if the list is sorted in ascending order, 2 – if the list is sorted in descending order, and 0 – if the list is not sorted. Write a function listsort which accepts a list as a parameter, and sorts it in ascending order. You can use any standard sorting algorithm, but don’t use built-in sort methods and functions. The function must sort and return the list. Write a script that asks user to enter 10 integer numbers, creates a list from those numbers, then calls verifsort function to detect if this list is sorted, prints message (list is sorted or list is not sorted) and if it’s not sorted then calls listsort function and prints the sorted list after listsortarrow_forwardint p =5 , q =6; void foo ( int b , int c ) { b = 2 * c ; p = p + c ; c = 1 + p ; q = q * 2; print ( b + c ); } main () { foo (p , q ); print p , q ; } Explain and print the output of the above code when the parameters to the foo function are passed by value. Explain and print the output of the above code when the parameters to the foo function are passed by reference. Explain and print the output of the above code when the parameters to the foo function are passed by value result. Explain and print the output of the above code when the parameters to the foo function are passed by name.arrow_forward
- write a recursive version. The function takes two string parameters, s1 and s2 and returns the starting index of s2 inside the first string s1, or -1 if s2 is not found in s1. You must not use any loops; you also cannot use the string member functions find or rfind. You may use the member functions size, at and substr. Your function must be recursive. CANNOT MODIFY GRAY AREAarrow_forwardCould you solve this Question please? Write a function called has_duplicates that takes a string parameter and returns True if the string has any repeated characters. Otherwise, it should return False. Implement has_duplicates by creating a histogram using the histogram function above. Do not use any of the implementations of has_duplicates that are given in your textbook. Instead, your implementation should use the counts in the histogram to decide if there are any duplicates. Write a loop over the strings in the provided test_dups list. Print each string in the list and whether or not it has any duplicates based on the return value of has_duplicates for that string. For example, the output for "aaa" and "abc" would be the following. aaa has duplicatesabc has no duplicates Print a line like one of the above for each of the strings in test_dups. Obs:Copy the code below into your program def histogram(s): d = dict() for c in s: if c not in d: d[c] =…arrow_forwardPlease use Python when answering this question: Also could you please run a doctest for each solution in the output to make sure the code works and returns everything in the output. Write a function numPairs that accepts two arguments, a target number and a list of numbers. The function then returns the count of pairs of numbers from the list that sum to the target number. In the first example the answer is 2 because the pairs (0,3) and (1,2) both sum to 3. The pair can be two of the same number, e.g. (2,2) but only if the two 2’s are separate twos in the list. In the last example below, there are three 2’s, so there are three different pairs (2,2) so there are 5 pairs total that sum to 4. output: >>> numPairs( 3, [0,1,2,3] ) 2 >>> numPairs( 4, [0,1,2,3] ) 1 >>> numPairs( 6, [0,1,2,3] ) 0 >>>numPairs( 4, [0,1,2,3,4,2] ) 3 >>> numPairs( 4, [0,1,2,3,4,2,2] ) 5 >>> numPairs( 4, [0,1,2,3,4,2,2] )==5 TRUEarrow_forward
- Write a program called p3.py that contains a function called reveal_recursive() that takes a word (string) and the length of the word (int) as input and has the following functionality: 1. prints the word where all characters are replaced by underscores 2. continue to print the word revealing one character at a time. i.e., the second line printed should print the first character followed by “_”’s representing the rest of the word. (see example below) 3. the function should end after printing the entire word once. 4. This function should be recursive Example: #the word is kangaroo ________ k_______ ka______ kan_____ kang____ kanga___ kangar__ kangaro_ kangarooarrow_forwardImplement the following function: Code should be written in python.arrow_forwardCan you answer the following questions in python using matplot.pyplot as pltarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
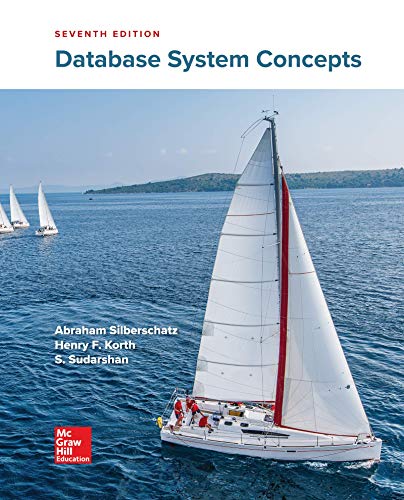
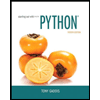
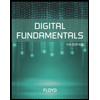
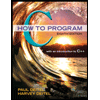
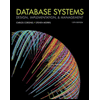
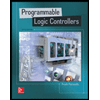