characters. Otherwise, it should return False. Implement has_duplicates by creating a histogram using the histogram function above. Do not use any of the implementations of
Could you solve this Question please?
Write a function called has_duplicates that takes a string parameter and returns True if the string has any repeated characters. Otherwise, it should return False.
Implement has_duplicates by creating a histogram using the histogram function above. Do not use any of the implementations of has_duplicates that are given in your textbook. Instead, your implementation should use the counts in the histogram to decide if there are any duplicates.
Write a loop over the strings in the provided test_dups list. Print each string in the list and whether or not it has any duplicates based on the return value of has_duplicates for that string. For example, the output for "aaa" and "abc" would be the following.
aaa has duplicates
abc has no duplicates
Print a line like one of the above for each of the strings in test_dups.
Obs:Copy the code below into your program
def histogram(s):
d = dict()
for c in s:
if c not in d:
d[c] = 1
else:
d[c] += 1
return d

Step by step
Solved in 4 steps with 2 images

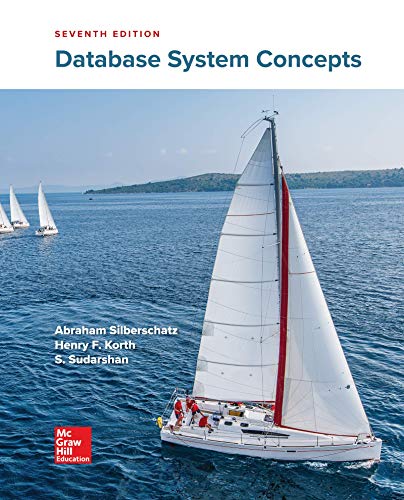
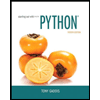
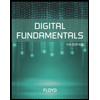
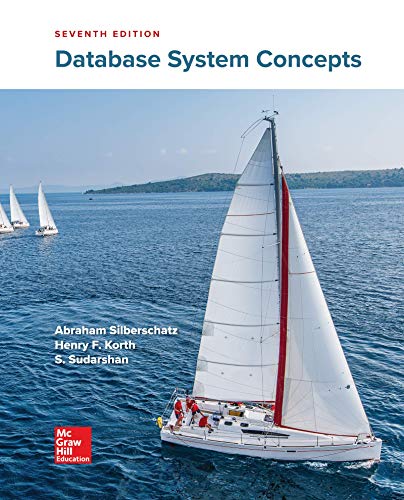
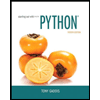
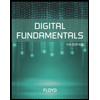
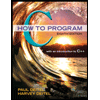
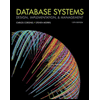
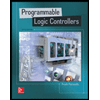