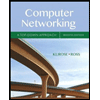
An 8-puzzle game consists of 8 sliding tiles, numbered by digits from 1 to 8 and arranged in a 3x3 array of nine cells. A configuration in the puzzle refers to some specific arrangement of the tiles in the array, where each digit is arranged into a different cell. One of the cell is empty (represented by a "*") and any adjacent tile can be moved into the empty cell. An example below
c | 1 | 2 | 3
b | 4 | * | 5
a | 6 | 7 | 8
------------------
Y/X a b c
We use a 3-tuple (number, X, Y) to represent the position of a digit, where number is the actual digit, and (X,Y) is the coordinate value of the digit in the current configuration (e.g., in the configuration above, we have (5,c,b), meaning the digit 5 is at the position of (c,b)).
Hence the configuration in the example can be represented as:
[(1,a,c), (2,b,c), (3,c,c), (4,a,b), (5,c,b), (6,a,a), (7,b,a), (8,c,a)].
Any move of an adjacent tile into the empty cell moves the current configuration into the one adjacent to it.
a) How many different configurations for this game?
b) List all the adjacent configurations from the example above.

Step by stepSolved in 2 steps

- check_game_over(): as the name suggests, this function should check to see if the game is over (if one side has no stones left in all of its pockets). It takes as an argument the game board and should return True if the game is over and False otherwise.arrow_forwardArray_1 25 70 80 99 30 15 86 77 66 89 55 24 31 20 30 40 15 91 65 63 17 30 29 45 18 49 71 46 89 88 87 86 Fig2 Use array_1 in Fig2 to answer the following question: What is the value in position [2][3]? __________. Group of answer choices 25 70 80 99 30 15 86 77 66 89 55 24 31 20 30 40 15 91 65 63 17 30 29 45 18 49 71 46 89 88 87 86 Not on Gridarrow_forwardID: A Name: ID: A 6. There are 32 students standing in a classroom. Two different algorithms are given for finding the average height of the students. Algorithm A Step 1: All students stand. gniwolldi odT gaihnel vd Step 2: A randomly selected student writes his or her height on a card and is seated. Step 3: A randomly selected standing student adds his or her height to the value on the card, records the new value on the card, and is seated. The previous value on the card is erased. Step 4: Repeat step 3 until no students remain standing. Step 5: The sum on the card is divided by 32. The result is given to the teacher. Algorithm B Step 1: All students stand. Step 2: Each student is given a card. Each student writes his or her height on the card. Step 3: Standing students form random pairs at the same time. Each pair adds the numbers written on their cards and writes the result on one student's card; the other student is seated. The previous value on the card is erased. Step 4: Repeat…arrow_forward
- The Spider Game Introduction: In this assignment you will be implementing a game that simulates a spider hunting for food using python. The game is played on a varying size grid board. The player controls a spider. The spider, being a fast creature, moves in the pattern that emulates a knight from the game of chess. There is also an ant that slowly moves across the board, taking steps of one square in one of the eight directions. The spider's goal is to eat the ant by entering the square it currently occupies, at which point another ant begins moving across the board from a random starting location. Game Definition: The above Figure illustrates the game. The yellow box shows the location of the spider. The green box is the current location of the ant. The blue boxes are the possible moves the spider could make. The red arrow shows the direction that the ant is moving - which, in this case, is the horizontal X-direction. When the ant is eaten, a new ant is randomly placed on one of the…arrow_forwardprogramming used: javaarrow_forwardA number maze is an n × n grid of positive integers. A token starts in the upper left corner; your goal is to move the token to the lower-right corner. On each turn, you are allowed to move the token up, down, left, or right; the distance you may move the token is determined by the number on its current square. For example, if the token is on a square labeled 3, then you may move the token three steps up, three steps down, three steps left, or three steps right. However, you are never allowed to move the token off the edge of the board. 6 3574 5 315 3 283 35 74 6 53 15 1 4 2 8 3 1 4 4 5 7 2 3 4 5 7 2 3 3 1 3 2★ 3 KT3 A 5 × 5 maze that can be solved in eight moves In this problem, you will design and analyze an efficient algorithm that either returns the minimum number of moves required to solve a given number maze, or correctly reports that the maze has no solution. Describe the solution to this problem at a high level, justify why it works, write down the pseudocode for your algorithm…arrow_forward
- Array_1 25 70 80 99 30 15 86 77 66 89 55 24 31 20 30 40 15 91 65 63 17 30 29 45 18 49 71 46 89 88 87 86 Fig2 Use array_1 in Fig2 to answer the following question: What is the value in position [1][5]? __________ Group of answer choices 25 70 80 99 30 15 86 77 66 89 55 24 31 20 30 40 15 91 65 63 17 30 29 45 18 49 71 46 89 88 87 86 Not on Gridarrow_forwardOpengl Help Programming Language: c++ I need help setting coordinate boundries for this program so the shape can't leave the Opengl window. The shape needs to stay visiable.arrow_forwardCodeWorkout Gym Course Q Search kola shreya@ columbusstate.edu Search exercises... X274: Recursion Programming Exercise: Cannonballs X274: Recursion Programming Exercise: Cannonballs Spherical objects, such as cannonballs, can be stacked to form a pyramid with one cannonball at the top, sitting on top of a square composed of four cannonballs, sitting on top of a square composed of nine. cannonballs, and so forth. Given the following recursive function signature, write a recursive function that takes as its argument the height of a pyramid of cannonballs and returns the number of cannonballs it contains. Examples: cannonball(2) -> 5 Your Answwer: 1 public int cannonball(int height) { 3. 4} Check my answer! Reset Next exercise Feedbackarrow_forward
- A number maze is a k ×k grid of positive integers. A token starts in the upper left corner and your goal is to move the token to the lower-right corner. On each turn, you are allowed to move the token up, down, left, or right; the distance you may move the token is determined by the number on its current square. For example, if the token is on a square labeled 3, then you may move the token exactly three steps up, three steps down, three steps left, or three steps right. However, you are never allowed to move the token off the edge of the board. Design and analyze an algorithm that in O(k2) time determines if there is a way to move the token in the given number maze or not.arrow_forwardBlue-Eyed Island: A group of individuals live on an island until a visitor arrives with an unusual order: all blue-eyed people must leave the island as quickly as possible. Every evening at 8:00 p.m., a flight will depart. Everyone can see everyone else's eye colour, but no one knows their own (nor is anyone allowed to tell them). Furthermore, they have no idea how many people have blue eyes, but they do know that at least one person does. How long will it take for the blue-eyed individuals to leave?arrow_forwardPlease written by computer source Please solve with C - NIM Gamearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
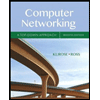
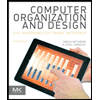
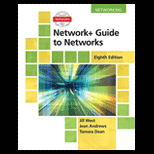
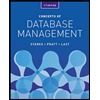
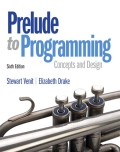
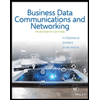