Task - Median elements (C Langugage) Given an array of integer elements, the median is the value that separates the higher half from the lower half of the values. In other words, the median is the central element of a sorted array. Since multiple elements of an input array can be equal to the median, in this task you are asked to compute the number of elements equal to the median in an input array of size N, with N being an odd number. Requirements Name your program project4_median.c. Follow the format of the examples below. The program will read the value of N, then read in the values that compose the array. These values are not necessarily sorted. The program should include the following function. Do not modify the function prototype. int compute_median(int *a, int n); a represents the input array, n is the length of the array. The function returns the median of the values in a. This function should use pointer arithmetic– not subscripting – to visit array elements. In other words, eliminate the loop index variables and all use of the [] operator in the function. In the main function, call the compute_median function, then compute and display the result. Pointer arithmetic is NOT required in the main function. Examples (your program must follow this format precisely) Example #1 Enter array size: 5 Enter array elements: 31 17 32 31 88 Output: 2 Example #2 Enter array size: 5 Enter array elements: 1 1 1 1 1 Output: 5 Example #3 Enter array size: 7 Enter array elements: 44 29 22 23 14 15 20 Output: 1
Task - Median elements (C Langugage)
Given an array of integer elements, the median is the value that separates the higher half from the lower half of the values. In other words, the median is the central element of a sorted array.
Since multiple elements of an input array can be equal to the median, in this task you are asked to compute the number of elements equal to the median in an input array of size N, with N being an odd number.
Requirements
- Name your program project4_median.c.
- Follow the format of the examples below.
- The program will read the value of N, then read in the values that compose the array. These values are not necessarily sorted.
- The program should include the following function. Do not modify the function prototype.
int compute_median(int *a, int n);
-
- a represents the input array, n is the length of the array. The function returns the median of the values in a.
- This function should use pointer arithmetic– not subscripting – to visit array elements. In other words, eliminate the loop index variables and all use of the [] operator in the function.
- In the main function, call the compute_median function, then compute and display the result.
- Pointer arithmetic is NOT required in the main function.
Examples (your program must follow this format precisely)
Example #1
Enter array size: 5
Enter array elements: 31 17 32 31 88
Output: 2
Example #2
Enter array size: 5
Enter array elements: 1 1 1 1 1
Output: 5
Example #3
Enter array size: 7
Enter array elements: 44 29 22 23 14 15 20
Output: 1

The Code is given below with output screenshot
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

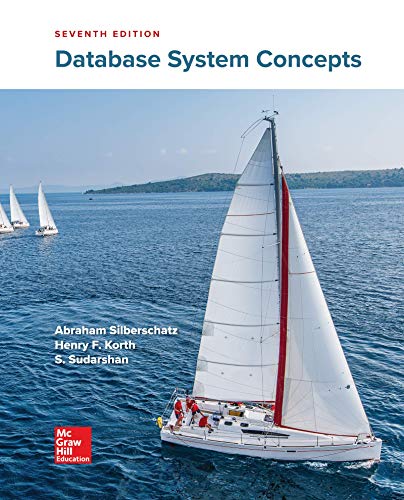
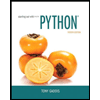
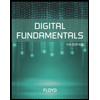
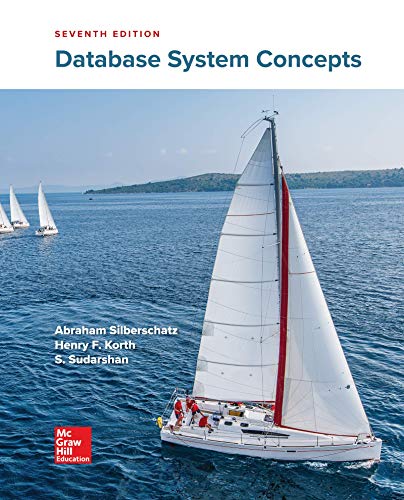
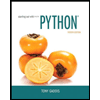
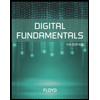
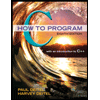
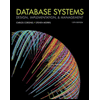
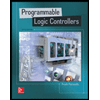