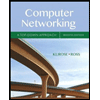
I am having a lot of errors pop up, and I need the pattern to follow the instructions below. Please don't send me the program that is already in Bartleby, it isn't right.
Professor instructions: Write a Java program that prompts the user to enter a security code that matches a specific pattern. Your program must approve the user's entry. The pattern consists of these characters in this sequence:
A lower case character, two upper case characters, two or three digits, two lower case characters, an upper case character, 2 or 3 lower case characters, 2 digits.
This is the code I have, but errors are poping up:
public static void main(String[] args) throws IOException {
Scanner scan = new Scanner(System.in);
System.out.print("Enter the code pattern ");
String s;
s = scan.next();
if (s.length() == 12) {
if (first12(s) && last(s.substring(10)) && middle(s.substring(8,10))) {
System.out.print("Yes, ");
System.out.print(s);
System.out.println("matches the pattern");
}
else{
System.out.print("No, ");
System.out.print(s);
System.out.println(" doen't matches the pattern");
}
}
else if(s.length()==14){
if(first12(s) && last(s.substring(11)) && middle(s.substring(8,11))){
System.out.print("Yes, ");
System.out.print(s);
System.out.println(" matches the pattern");
}
else{
System.out.print("No, ");
System.out.print(s);
System.out.println(" doen't matches the pattern");
}
}
else{
System.out.print("No, ");
System.out.print(s);
System.out.println(" doen't matches the pattern");
}
scan.close();
}
public static Boolean first12(String s){
char c0,c1,c2,c3,c4,c5,c6,c7,c8,c9,c10,c11;
c0 = s.charAt(0);
c1 = s.charAt(1);
c2 = s.charAt(2);
c3 = s.charAt(3);
c4 = s.charAt(4);
c5 = s.charAt(5);
c6 = s.charAt(6);
c7 = s.charAt(7);
c8 = s.charAt(8);
c9 = s.charAt(9);
c10 = s.charAt(10);
c11 = s.charAt(11);
if(Character.isLowerCase(c0) &&
Character.isUpperCase(c1) &&
Character.isUpperCase(c2) &&
Character.isDigit(c3) &&
Character.isDigit(c4) &&
Character.isLowerCase(c5) &&
Character.isLowerCase(c6) &&
Character.isUpperCase(c7) &&
Character.isLowerCase(c8) &&
Character.isLowerCase(c9) &&
Character.isDigit(c10) &&
Character.isDigit(c11)){
return true;
}
else {
return false;
}
}
public static Boolean last(String s) {
char c0, c1, c2;
c0 = s.charAt(0);
c1 = s.charAt(1);
c2 = s.charAt(2);
if (Character.isLowerCase(c0) && Character.isUpperCase(c1) && Character.isUpperCase(c2)) {
return true;
}
else {
return false;
}
}
public static Boolean middle(String s) {
if (s.length() == 2) {
char c0, c1;
c0 = s.charAt(0);
c1 = s.charAt(1);
if ( Character.isLowerCase(c0) && Character.isUpperCase(c1)) {
return true;
}
else {
return false;
}
}
else {
char c0, c1, c2;
c0 = s.charAt(0);
c1 = s.charAt(1);
c2 = s.charAt(2);
if (Character.isLowerCase(c0) && Character.isUpperCase(c1) && Character.isUpperCase(c2)) {
return true;
}
else {
return false;
}
}
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- CODE IN C# NOT JAVA (reject if you can't do C# please): Write an application that runs 1,000,000 games of craps and answers the following questions (explain each step): How many games are won on the first roll, second roll, …, twentieth roll and after the twentieth roll? How many games are lost on the first roll, second roll, …, twentieth roll and after the twentieth roll? What are the chances of winning at craps? [Note: You should discover that craps is one of the fairest casino games. What do you suppose this means?] What is the average length of a game of craps?arrow_forwardCongrats! You work for Zillow now. Your first task is to write an algorithm that recommends apartments based on a user’s preferences. Zillow offers the following three properties for rent: Apartment A – 600 square feet, pets allowed, $1400/month Apartment B – 800 square feet, no pets, $1600/month Apartment C – 1000 square feet, pets allowed, $1800/month Write an algorithm for a program that does the following: Prompt the user for the minimum square footage they will accept, how many pets they have, and the maximum price they’d pay per month, and then calculate and output the apartments that match their preferences. If there is no apartment that matches their preferences, print out "No matches, sorry!". Make sure the inputs are valid--negative square footages, prices, and pet numbers don’t make sense!arrow_forwardcan you please write it in java.util.scanner form Write a program that prompts the user to enter a number within the range of 1 through 10. The program should display the Roman numeral version of that number. If the number is outside the range of 1 through 10, the program should display an error message. but use switch statement for the program.arrow_forward
- Alert ⚠️ You guys use AI tool to answer. Last time I found plagiarism and AI detection in my answer. Now If you will use these things I'll surely give multiple downvotes and will report sure.arrow_forwardJava Programming: Write a program that reads five integer values from the user, then analyzes them as if they were a hand of cards. When your program runs it might look like this (user input is in orange for clarity): Enter five numeric cards, no face cards. Use 2 - 9. Card 1: 8 Card 2: 7 Card 3: 8 Card 4: 2 Card 5: 9 Pair! (This is a pair, since there are two eights). You are only required to find the hands Pair and Straight.arrow_forwardUsing pythonarrow_forward
- Use C language to write your program. Show your code in a picture with commentsarrow_forwardOn Thursday, November 4, 2021, Big Man Games wrote: Computer programs are great at computing mathematical formulas. Once the formula is properly encoded you can use the code as much as you want without reprogramming it and you can share it with non-programmers without any trouble. This lab is an example of such a formula. Once you program it you won’t have to worry about the area of a circle again. Write and test a program that computes the area of a circle. This program should request a number representing a radius as input from the user. Use the formula 3.14 × radius2 to compute the area. Tip: There are a couple of ways to code an exponent. Look in the Operators unit for help (and you can’t use an x for multiplication). Tip: You will need to use the float data type to compute the remainder. The output should explain the results. Don’t just print a number. Tip: For your print statement you will need to use the comma, “,”, or plus, “+” symbols to stitch your output together. (“The…arrow_forwardJava Programming: The program will figure out a number chosen by a user. Ask user to think of a number from 1 to 100. The program will make guesses and the user will tell the program to guess higher (h) or lower (l). Sample run of the program might look like below : Guess a number from 1 to 100. (here, the user is thinking of 81) Is it 50? (h/l/c): h Is it 75? (h/l/c): h Is it 88? (h/l/c): l Is it 81? (h/l/c): c Great! Do you want to play again? (y/n): y Guess a number from 1 to 100. (here the user is thinking 37) Is it 50? (h/l/c): l Is it 25? (h/l/c): h Is it 37? (h/l/c): c Great! Do you want to play again? (y/n): n our program should implement the binary search algorithm. Every time the program makes a guess it should guess the midpoint of the remaining possible values. Consider the first example above, in which the user has chosen the number 81: On the first guess, the possible values are 1 to 100. The midpoint is 50. The user responds by saying “higher” On the second…arrow_forward
- Help me debug this exercise using Javaarrow_forwardDevelop a program that allows the user to enter a start value of 1 to 4, a stop value of 5 to 12 and a multiplier of 2 to 8. The program must display a multiplication table with results using these values. For example, if the user enters a start value of 3, a stop value of 7 and a multiplier value of 3, the table should be displayed as follows: Multiplication Table 3 x 3 = 9 3 x 4 = 12 3 x 5 = 15 3 x 6 = 18 3 x 7 = 21 additionally Each multiplication problem must be displayed with a problem number. The program must prompt the user to enter an answer to each of the multiplication problems. The correct answer should be displayed after the user enters an answer. A message should be displayed informing the user that the answer entered was correct or incorrect followed by an appropriate motivating comment. A running record of the number of correct and incorrect responses must be kept. After all the problems have been presented the user must be informed of his/her performance; number of…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
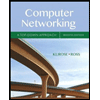
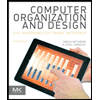
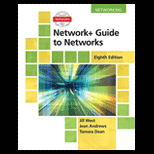
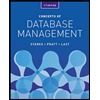
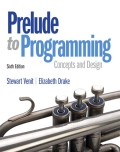
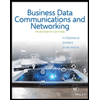