Create class Test in a file named Test.java. This class contains a main program that performs the following actions: Instantiate a doubly linked list. Insert strings “a”, “b”, and “c” at the head of the list using three Insert() operations. The state of the list is now [“c”, “b”, “a”]. Set the current element to the second-to-last element with a call to Tail() followed by a call to Previous()Then insert string “d”. The state of the list is now [“c”, “d”, “b”, “a”]. Set the current element to past-the-end with a call to Tail() followed by a call to Next(). Then insert string “e”. The state of the list is now [“c”, “d”, “b”, “a”, “e”] . Print the list with a call to Print() and verify that the state of the list is correct.
Create class Test in a file named Test.java. This class contains a main
performs the following actions:
-
Instantiate a doubly linked list.
-
Insert strings “a”, “b”, and “c” at the head of the list using three Insert() operations. The state of the list is now [“c”, “b”, “a”].
-
Set the current element to the second-to-last element with a call to Tail() followed by a call to Previous()Then insert string “d”. The state of the list is now [“c”, “d”, “b”, “a”].
-
Set the current element to past-the-end with a call to Tail() followed by a call to Next(). Then insert string “e”. The state of the list is now [“c”, “d”, “b”, “a”, “e”] .
-
Print the list with a call to Print() and verify that the state of the list is correct.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

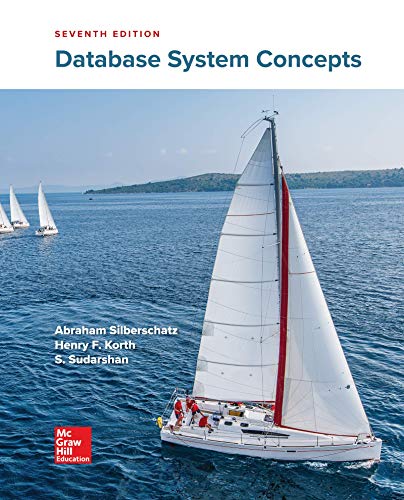
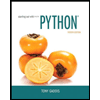
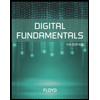
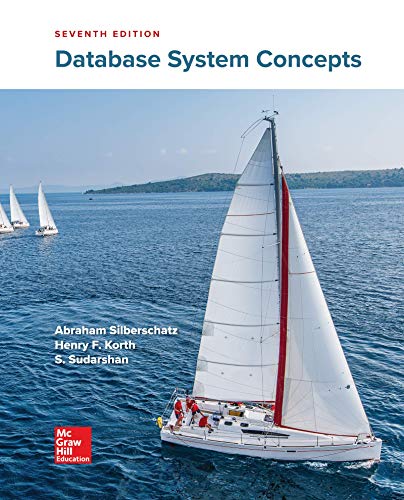
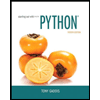
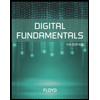
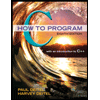
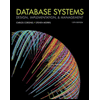
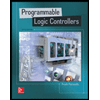