using python language Use the function design recipe to develop a function named unique_list. The function takes a list of strings, which may be empty. The function returns a new list that includes the first occurrence of each value in a list and omits later repeats. The returned list should include the first occurrences of values in a list in their original order. For example, if a list contains the following animals ['cat', 'dog', 'cat', 'bug', 'dog', 'ant', 'dog', 'bug'], the unique_list function returns a unique list: ['cat', 'dog', 'bug', 'ant'].
Automated testing is required
Your solutions can use:
• the [] operator to get and set list elements (e.g., lst[i] and lst[i] = a)
• the in and not in operators (e.g., a in lst)
• the list concatenation operator (e.g., lst1 + lst2)
• the list replication operator (e.g., lst * n or n * lst)
• Python's built-in len, min and max functions, unless otherwise noted.
Your solutions cannot use:
• list slicing (e.g., lst[i : j] or (lst[i : j] = t)
• the del statement (e.g., del lst[0])
• Python's built-in reversed and sorted functions.
• any of the Python methods that provide list operations, e.g., sum, append, clear, copy, count, extend, index, insert, pop, remove, reverse and sort
• list comprehensions
using python language Use the function design recipe to develop a function named unique_list. The function takes a list of strings, which may be empty. The function returns a new list that includes the first occurrence of each value in a list and omits later repeats. The returned list should include the first occurrences of values in a list in their original order. For example, if a list contains the following animals ['cat', 'dog', 'cat', 'bug', 'dog', 'ant', 'dog', 'bug'], the unique_list function returns a unique list: ['cat', 'dog', 'bug', 'ant'].

Step by step
Solved in 2 steps

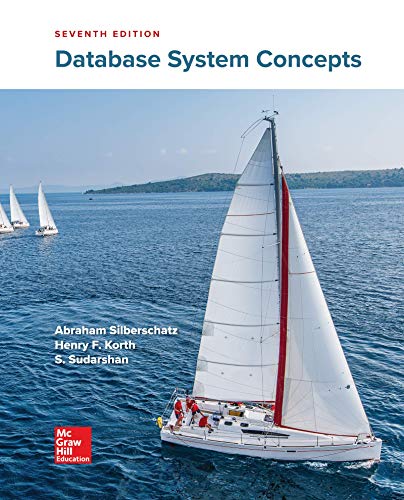
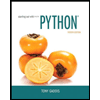
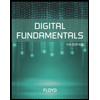
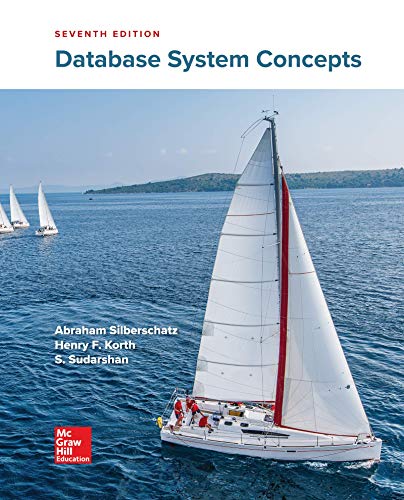
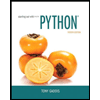
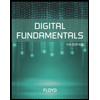
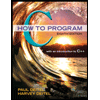
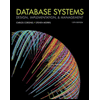
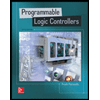