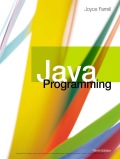
a.
Explanation of Solution
Program:
File name: “CollegeCourse.java”
//Define a class named CollegeCourse
public class CollegeCourse
{
//Declare the private variables
private String courseID;
private int credits;
private char grade;
//Define a get method that returns the course ID
public String getID()
{
//Return the value
return courseID;
}
//Define a get method that returns the credit hours
public int getCredits()
{
//Return the value
return credits;
}
//Define a get method that returns the letter grade
public char getGrade()
{
//Return the value
return grade;
}
//Define a set method that takes the course ID
public void setID(String idNum)
{
//Assign the value
courseID = idNum;
}
//Define a set method that takes the credit hours
public void setCredits(int cr)
{
//Assign the value
credits = cr;
}
//Define a set method that takes the letter grade
public void setGrade(char gr)
{
//Assign the value
grade = gr;
}
}
File name: “Student...
b.
Explanation of Solution
Program:
File name: “InputGrades.java”
//Import necessary header files
import college.CollegeCourse;
import college.Student;
import javax.swing.*;
//Define a class named InputGrades
public class InputGrades
{
//Define a main method
public static void main(String[] args)
{
//Declare an array to store data of 10 students
Student[] students = new Student[10];
//Declare the variables and initialize the values
int x, y, z;
String courseEntry, entry = "", message;
int idEntry, credits;
char gradeEntry = ' ';
boolean isGoodGrade = false;
final int NUM_COURSES = 5;
//Declare an array to store five grades
char[] grades = {'A', 'B', 'C', 'D', 'F'};
//For loop to be executed until x exceeds 10
for(x = 0; x < students.length; ++x)
{
//Create a student object
Student stu = new Student();
//Prompt the user to enter the student ID
entry = JOptionPane.showInputDialog(null, "For student #" +
(x + 1) + ", enter the student ID");
idEntry = Integer.parseInt(entry);
//Function call
stu.setID(idEntry);
//For loop to be executed until y exceeds 5
for(y = 0; y < NUM_COURSES; ++y)
{
//Prompt the user to enter the course
courseEntry = JOptionPane.showInputDialog(null,
"For student #" + (x + 1) + ", enter course #" +
(y + 1));
//Prompt the user to enter the credits for each //course
entry = JOptionPane.showInputDialog(null,
"For student #" + (x + 1) +
", enter credits for course #" + (y + 1));
credits = Integer.parseInt(entry);
//Assign the value false to isGoodGrade
isGoodGrade = false;
//While the user does not enter isGoodGrade
while(!isGoodGrade)
{
//Prompt the user to enter the grade for //course
entry = JOptionPane.showInputDialog(null,
"For student #" + (x + 1) +
", enter grade for course #" + (y + 1));
gradeEntry = entry...

Trending nowThis is a popular solution!

- Design a class named BaseBallGame that has the fields for two names and a final score for each team. Include methods to set and get the values for each data field. Create the class diagram and write the pseudocode that defines the class. Design an application (submit pseudocode) that declares three BaseBallGame objects and sets and displays their values. Design an application (submit pseudocode) that declares an array of 12 BaseBallGame objects. Prompt the user for data for each object, and display all the values. Then pass each object to a method that displays the name of the winning team or "Tie" if the score is a tie.arrow_forwardComplete the following tasks: Design a class named StockTransaction that holds a stock symbol (typically one to four characters), stock name, and price per share. Include methods to set and get the values for each data field. Create the class diagram and write the pseudocode that defines the class. Design an application that declares two StockTransaction objects and sets and displays their values. Design an application that declares an array of 10 StockTransactionobjects. Prompt the user for data for each object, and then display all the values. Design an application that declares an array of 10 StockTransactionobjects. Prompt the user for data for each object, and then pass the array to a method that determines and displays the two stocks with the highest and lowest price per share.arrow_forwardTask 2: Create a class that includes a data member that holds a “serial number” for each object created from the class. That is, the first object created will be numbered 1, the second 2, and so on. To do this, you’ll need another data member that records a count of how many objects have been created so far. (This member should apply to the class as a whole; not to individual objects. What keyword specifies this?) Then, as each object is created, its constructor can examine this count member variable to determine the appropriate serial number for the new object. Add a member function that permits an object to report its own serial number. Then write a main() program that creates three objects and queries each one about its serial number. They should respond I am object number 2, and so on. Paste only class definition here Paste function definitions of member functions one by one in different rows Member function 1() here Member function 2() here…arrow_forward
- Appointment Class Requirements The appointment object shall have a required unique appointment ID string that cannot be longer than 10 characters. The appointment ID shall not be null and shall not be updatable. The appointment object shall have a required appointment Date field. The appointment Date field cannot be in the past. The appointment Date field shall not be null.Note: Use java.util.Date for the appointmentDate field and use before(new Date()) to check if the date is in the past. The appointment object shall have a required description String field that cannot be longer than 50 characters. The description field shall not be null. Appointment Service Requirements The appointment service shall be able to add appointments with a unique appointment ID. The appointment service shall be able to delete appointments per appointment ID.arrow_forwardComplete the following tasks:a. Design a class named AutomobileLoan that holds a loan number, make and model of automobile, and balance. Include methods to set values for each data field and a method that displays all the loan information. Create the class diagram and write the pseudocode that defines the class.b. Design an application that declares two AutomobileLoan objects and sets and displays their values.c. Design an application that declares an array of 20 AutomobileLoan objects. Prompt the user for data for each object, and then display all the values.d. Design an application that declares an array of 20 AutomobileLoan objects. Prompt the user for data for each object, and then pass the array to a method that determines the sum of the balances.arrow_forwardCreate a Student class that have two data members id (assign to your ID) and name (assign to your name). Create the object of the Student class by new keyword and printing the objects value. You may name your object as Student1. The output should be like this: 20170500 Asma Zubaidaarrow_forward
- Create an Employee data type, and then create three employee objects. Each employee object has his/her name, employee ID, and balance. Set the first employee object’s name as “Lacey”, ID as 1234, and balance as 100; Set the second employee object’s name as “Dylan”, ID as 5678, and balance as 0; Set the third employee object’s name as “James”, ID as 9012, and balance as 50; Write a method for withdrawing money; Write a method for depositing money; Create one constructor for the objects Lacey and James, and one constructor for the object Dylan; Set the fields as private. Withdraw 50 on all three employees, and after that display each of their names, IDs, and balances.arrow_forwarddesign a Club class and use it to create an application that simulates asoccer league tournament. You are not limited to the features included in these instructionsDesign the Club class, to be used in your application, containing: A private data member code of type string that holds the three letters club’s abbreviation(ex, FCB, LIV, RAM ...) A private data member name of type string that holds the club’s name (ex, FC Barcelona,Liverpool, Real Madrid …) A private data member round of type integer that holds the number of rounds the club hasplayed so far in the current league. A private data member wins of type integer that holds the number of times the club haswon in the current league. A private data member draws of type integer that holds the number of times the club hasbeen drawn in the current league. A private data member losses of type integer that holds the number of times the club haslost in the current league. A private data member goals of type integer that holds the…arrow_forwardIntroduction In this project, you will complete a C++ class by writing a copy constructor, destructor, and assignment operator. Furthermore, you will write a tester class and a test program and use Valgrind to check that your program is free of memory leaks. Finally, you will submit your project files on GL. If you have submitted programs on GL using shared directories (instead of the submit command), then the submission steps should be familiar. In this project we develop an application which holds the information about a digital art piece. There are two classes in this project. • The Random class generates data. The implementation of this class is provided. It generates random int numbers for colors. To create an object of this class we need to specify min and max values for the constructor. The colors fall between 30 and 37 inclusively. The Art class generates random colors and stores the color values. You implement the Art class. An object of the Art class is a digital masterpiece.…arrow_forward
- a - Create a FitnessTracker class that includes data fields for a fitness activity, the number of minutes spent participating, and the date. The class includes methods to get each field. In addition, create a default constructor that automatically sets the activity to running, the minutes to 0, and the date to January 1 of the current year. Save the file as FitnessTracker.java. Create an application that demonstrates each method works correctly, and save it as TestFitnessTracker.java. b - Create an additional overloaded constructor for the FitnessTracker class you created in Exercise 3a. This constructor receives parameters for each of the data fields and assigns them appropriately. Add any needed statements to the TestFitnessTracker application to ensure that the overloaded constructor works correctly, save it, and then test it. c - Modify the FitnessTracker class so that the default constructor calls the three-parameter constructor. Save the class as FitnessTracker2.java. Create an…arrow_forwardUsing Java & Apache NetBeans Provide the screenshots for NetBeans Create a class named Customer that will determine the monthly repayment amount due by a customer for a product bought on credit. The class has five fields: customer name, contact number, product price, number of months and the monthly repayment amount. Write get and set methods for each field, except for the monthly repayment amount field. The set methods must prompt the user to enter the values for the following fields: customer name, contact number, product price and number of months. This class also needs a method to calculate the monthly repayment amount (product price divided by the number of months). Add a subclass named Finance_Period that will determine if a customer will pay interest or not. If the number of months to pay for the product is greater than three, the customer will pay 25% interest, else no interest applies. The maximum number of months to pay for the product is 12. Override the…arrow_forwardStatic & Not Final Field: Accessed by every object, Changing Non-Static & Final Field: Accessed by object itself, Non-Changing Static & Final: Accessed by every object, Non-Changing Non-Static & Not Final Field: Accessed by object itself, ChangingRead the following situation and decide how the variables should be defined. You have a class named HeartsPlayerA round of Hearts starts with every player having 13 cardsPlayers then choose 3 cards to “trade” with a player (1st you pass left, 2nd you pass right, 3rd you pass across, 4th you keep)Players then strategically play cards in order to have the lowest scoreAt the end of the round, points are cumulatively totaled for each player.If one player’s total is greater than 100, the game ends and the player with the lowest score wins. 1. How should the following data fields be defined (with respect to final and static)?(a) playerPosition (These have values of North, South, East, or West)(b) directionOfPassing(c) totalScore…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
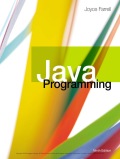