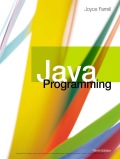
Concept explainers
Explanation of Solution
Program:
File name: “CertOfDeposit.java”
//Import necessary header files
import java.time.LocalDate;
//Define a class named CertOfDeposit
public class CertOfDeposit
{
//Declare the private variables
private int certificateNumber;
private String lastName;
private double balance;
private LocalDate issueDate;
private LocalDate maturityDate;
/*Define a constructor that sets the instance variables
to class CertOfDeposit */
public CertOfDeposit(int certificateNumber,String lastName,double balance, LocalDate issueDate)
{
//Refers to the instance variables
this.certificateNumber=certificateNumber;
this.lastName=lastName;
this.balance=balance;
this.issueDate=issueDate;
this.maturityDate=issueDate.plusYears(1);
}
//Define a method that sets the certificate number
public void setCertificateNumber(int certificateNumber)
{
this.certificateNumber=certificateNumber;
}
//Define a method that sets the last name
public void setLastName(String lastName)
{
this.lastName=lastName;
}
//Define a method that sets the balance amount
public void setBalance(double balance)
{
this.balance=balance;
}
//Define a method that sets the issue date
public void setIssueDate(LocalDate issueDate)
{
this.issueDate=issueDate;
}
//Define a method that sets the maturity date
public void setMaturityDate(LocalDate maturityDate)
{
this.maturityDate=maturityDate;
}
//Define a method that returns the certificate number
public int getCertificateNumber()
{
//Return the value
return certificateNumber;
}
//Define a method that returns the last name
public String getLastName()
{
//Return the value
return lastName;
}
//Define a method that returns the balance amount
public double getBalance()
{
//Return the value
return balance;
}
//Define a method that returns the issue date as LocalDate object
public LocalDate getIssueDate()
{
//Return the value
return issueDate;
}
//Define a method that returns the maturity date as LocalDate object
public LocalDate getMaturityDate()
{
//Return the value
return maturityDate;
}
}
File name: “CertOfDepositArray.java”
//Import necessary header files
import java.time.LocalDate;
import java.util.Scanner;
//Define a class named CertOfDepositArray
public class CertOfDepositArray
{
//Define a main method
public static void main(String[] args)
{
//Declare the variables
int certificateNumber;
String name;
double balance;
int day;
int month;
int year;
final int size=5;
//Create an object for Scanner class
Scanner scanner=new Scanner(System...

Trending nowThis is a popular solution!

- Create a class name Taxpayer. Data fields for Taxpayer include yearly gross income and Social Security number (use an int for the type, and do not use dashes within the Social Security number). Methods include a constructor that requires a value for both data fields and two methods that each return one of the data field values. Write an application named USeTaxpayer that declares an array of 10 Taxpayer objects. Set each Social Security number to 999999999 and each gross income to zero. Display the 10 Taxpayer objects. Save the files as TAxpayer.java and USeTAxpayer.javaarrow_forwardThis is the question - The developers of a free online game named Sugar Smash have asked you to develop a class named SugarSmashPlayer that holds data about a single player. The class contains the following fields: idNumber - the player’s ID number (of type int) name - the player's screen name (of type String) scores - an array of integers that stores the highest score achieved in each of 10 game levels Include get and set methods for each field. The get method for scores should require the game level to retrieve the score for. The set method for scores should require two parameters—one that represents the score achieved and one that represents the game level to be retrieved or assigned. Display an error message if the user attempts to assign or retrieve a score from a level that is out of range for the array of scores. Additionally, no level except the first one should be set unless the user has earned at least 100 points at each previous level. If a user tries to set a score for a…arrow_forwardThis is the question - The developers of a free online game named Sugar Smash have asked you to develop a class named SugarSmashPlayer that holds data about a single player. The class contains the following fields: idNumber - the player’s ID number (of type int) name - the player's screen name (of type String) scores - an array of integers that stores the highest score achieved in each of 10 game levels Include get and set methods for each field. The get method for scores should require the game level to retrieve the score for. The set method for scores should require two parameters—one that represents the score achieved and one that represents the game level to be retrieved or assigned. Display an error message if the user attempts to assign or retrieve a score from a level that is out of range for the array of scores. Additionally, no level except the first one should be set unless the user has earned at least 100 points at each previous level. If a user tries to set a score for a…arrow_forward
- This is the question - The developers of a free online game named Sugar Smash have asked you to develop a class named SugarSmashPlayer that holds data about a single player. The class contains the following fields: idNumber - the player’s ID number (of type int) name - the player's screen name (of type String) scores - an array of integers that stores the highest score achieved in each of 10 game levels Include get and set methods for each field. The get method for scores should require the game level to retrieve the score for. The set method for scores should require two parameters—one that represents the score achieved and one that represents the game level to be retrieved or assigned. Display an error message if the user attempts to assign or retrieve a score from a level that is out of range for the array of scores. Additionally, no level except the first one should be set unless the user has earned at least 100 points at each previous level. If a user tries to set a score for a…arrow_forwardCreate a class named Salesperson. Data fields for Salesperson include an integer ID number and a double annual sales amount. Methods include a constructor that requires values for both data fields, as well as get and set methods for each of the data fields. Write an application named DemoSalesperson that declares an array of 10 Salesperson objects. Set each ID number to 9999 and each sales value to zero. Display the 10 Salesperson objects.arrow_forwardDesign a class named BaseBallGame that has the fields for two names and a final score for each team. Include methods to set and get the values for each data field. Create the class diagram and write the pseudocode that defines the class. Design an application (submit pseudocode) that declares three BaseBallGame objects and sets and displays their values. Design an application (submit pseudocode) that declares an array of 12 BaseBallGame objects. Prompt the user for data for each object, and display all the values. Then pass each object to a method that displays the name of the winning team or "Tie" if the score is a tie.arrow_forward
- Suppose you are using a jQuery animation to make an element change color when the user clicks on it, and you also want to execute a function as soon as the animation finishes running. How can you do this? a. Pass the function in as the first argument to theanimate()method called on the element. b. Use anif elseblock with thehasClass()method to determine when to call the function. c. Pass the function in as the final argument to theanimate()method called on the element. d. You cannot accomplish this using jQuery commands.arrow_forwardIn JavaScript, the following statement _____.let town = { name: "Helena", county: "Shelby", population: 18500}; Question options: A creates an object containing items that are referenced by index value B instantiates a town object that the program can call Array object methods on C produces an object literal with three methods that can be called on it D creates an associative array containing three key-value pairsarrow_forwardWrite a program called ArrayOfStudents that displays information of three students. You need to use array of objects. Each element in the array need to be initialized using a constructor. Each Student will have (name, id and Specialization). The Program will display the student information by invoking the method showStudentData(). Challenge: Can You modify the program to allow the user to enter the number of students stored in the array of objects, and ask the user to insert the details of each student.arrow_forward
- Design a class named AutomobileLoan that holds a loan number, make and model of automobile, and balance. Include methods to set values for each data field and a method that displays all the loan information. design an application that declares an array of 20 AutomobileLoan object. Prompt the user for data for each object and then display all the valuesarrow_forwardusing JAVA Create a class called Transcript which will take the following parameters for its constructor:- 1. The UoF ID of the student 2. The full name of the student 3. The year in which the student joined 4. The student's GPA score NOTE: The class also has a class field which is a 2D array with 32 elements containing the course codes and grades obtained by the studentarrow_forwardThis is the question I am stuck on - Radio station KJAVA wants a class to keep track of recordings it plays. Create a class named Recording that contains fields to hold methods for setting and getting a Recording’s title, artist, and playing time in seconds. Write an application that instantiates five Recording objects and prompts the user for values for the data fields. Then prompt the user to enter which field the Recordings should be sorted by—(S)ong title, (A)rtist, or playing (T)ime. Perform the requested sort procedure, and display the Recording objects. This is what I have so far - public class Recording { private String song; private String artist; private int playTime; public void setSong(String title) { this.song = title; } public void setArtist(String name) { this.artist = name; } public void setPlayTime(int time) { this.playTime = time; } public String getSong() { return song; } public String…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
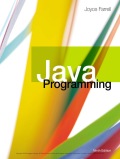
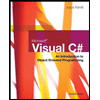
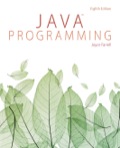