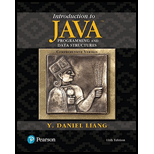
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 7.5, Problem 7.5.2CP
Program Plan Intro
Array:
In Java, the “array” is a data structure, which contains similar data type elements in continuous memory location.
- Each element in an array can be identified by its index value.
- The first element of an array will be stored at the index place “0”.
- The entire memory locations occupied by an array will contain the same data type elements.
Creating an array:
An array can be created after the declaration; the array creation can be performed by using the “new” operator and its variable reference.
The syntax to create an array is as follows:
typeOfElement[] arrayReferenceVariable = new typeOfElement[arraysize]
- The “typeOfElement” refers the data type of the variable.
- The “arrayReferenceVariable” refers the name of the variable to refer the array location.
- The “new” operator refers the new memory location allocation.
- The “arraysize” refers the size of the array.
The size of the array is fixed once the array has been created.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Below is code which defines and creates an Array, myList: int [] myList = new int [5]; //define and createThe loop below stores the values 1 to 5 in myList:for (int i = 0; i < 5; i ++) {myList [ i ] = i + 1; //store values using a loop}The loop below prints the values from first to last in myList:for (int i = 0; i < myList.length; i++) { System.out.print(myList [ i ] + " ");}The code below is used to get user from the user and calculate the sum:import java.util.*;public class WhileWithInput {public static void main(String[] args) {Scanner reader = new Scanner (System.in);int count = 1; double total = 0; while (count <= 5) {System.out.println("Enter a decimal number"); double number = reader.nextDouble();total = total + number;count = count + 1;System.out.println("The total is " + total); }System.out.println("The total is " + total); }}1CPS 2231 Chapter 7 – lab 2 Fall 2019Use the examples on page 1 to complete the lab below. Please submit a screen print of the Eclipse editor…
Arrays: create an array of a given type and populate its values. Use of for loop to traverse through an array to do the following : to print the elements one by one, to search the array for a given value.
ArrayList: create an ArrayList containing elements of a given type . Use some of the common ArrayList methods to manipulate contents of the ArrayList. Write methods that will take an ArrayList as its parameter/argument ; and/or return an ArrayList reference variable as its return type.
Searching for an object in an Array: Loop through the ArrayList to extract each object and to check if this object’s attribute has a given value.
Explain how and why interfaces are used in Java Collection Framework.
Explain the major differences between a Stack and a Queue. Be able to use stack and queue methods.
What is meant by O(N) notation? Express the complexity of a given code using the O(N) notation.
1. An array is a container that holds a group of values of a
2. Each item in an array is called an
3. Each element is accessed by a numerical
4. The index to the first element of an array is 0 and the
index to the last element of the array is the length of the
5. Given the following array declaration, determine which of
the three statements below it are true.
int [] autoMobile = new int [13];
i. autoMobile[0] is the reference to the first element in
the array.
ii. autoMobile[13] is the reference to the last element in
the array.
ii. There are 13 integers in the autoMobile array.
6. In java new is a
which is used to allocate
memory
7. Declare a one-dimensional array named score of type int
that can hold9 values.
8. Declare and initialize a one-dimensional byte array
named values of size 10 so that all entries contain 1.
9. Declare and initialize a one-dimensional array to store
name of any 3 students.
10. Declare a two-dimensional array named mark of type
double that can hold 5x3…
Chapter 7 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 7.2 - Prob. 7.2.1CPCh. 7.2 - Prob. 7.2.2CPCh. 7.2 - What is the output of the following code? int x =...Ch. 7.2 - Indicate true or false for the following...Ch. 7.2 - Which of the following statements are valid? a....Ch. 7.2 - Prob. 7.2.6CPCh. 7.2 - What is the array index type? What is the lowest...Ch. 7.2 - Write statements to do the following: a. Create an...Ch. 7.2 - What happens when your program attempts to access...Ch. 7.2 - Identify and fix the errors in the following code:...
Ch. 7.2 - What is the output of the following code? 1....Ch. 7.4 - Will the program pick four random cards if you...Ch. 7.5 - Use the arraycopy method to copy the following...Ch. 7.5 - Prob. 7.5.2CPCh. 7.7 - Suppose the following code is written to reverse...Ch. 7.8 - Prob. 7.8.1CPCh. 7.8 - Prob. 7.8.2CPCh. 7.9 - Prob. 7.9.1CPCh. 7.9 - Prob. 7.9.2CPCh. 7.10 - If high is a very large integer such as the...Ch. 7.10 - Prob. 7.10.2CPCh. 7.10 - Prob. 7.10.3CPCh. 7.11 - Prob. 7.11.1CPCh. 7.11 - How do you modify the selectionSort method in...Ch. 7.12 - What types of array can be sorted using the...Ch. 7.12 - To apply java.util.Arrays.binarySearch (array,...Ch. 7.12 - Show the output of the following code: int[] list1...Ch. 7.13 - This book declares the main method as public...Ch. 7.13 - Show the output of the following program when...Ch. 7 - (Assign grades) Write a program that reads student...Ch. 7 - (Reverse the numbers entered) Write a program that...Ch. 7 - (Count occurrence of numbers) Write a program that...Ch. 7 - (Analyze scores) Write a program that reads an...Ch. 7 - (Print distinct numbers) Write a program that...Ch. 7 - (Revise Listing 5.1 5, PrimeNumber.java) Listing...Ch. 7 - (Count single digits) Write a program that...Ch. 7 - (Average an array) Write two overloaded methods...Ch. 7 - (Find the smallest element) Write a method that...Ch. 7 - Prob. 7.10PECh. 7 - (Statistics: compute deviation) Programming...Ch. 7 - (Reverse an array) The reverse method in Section...Ch. 7 - Prob. 7.13PECh. 7 - Prob. 7.14PECh. 7 - 7 .15 (Eliminate duplicates) Write a method that...Ch. 7 - (Execution time) Write a program that randomly...Ch. 7 - Prob. 7.17PECh. 7 - (Bubble sort) Write a sort method that uses the...Ch. 7 - (Sorted?) Write the following method that returns...Ch. 7 - (Revise selection sort) In Listing 7 .8, you used...Ch. 7 - (Sum integers) Write a program that passes an...Ch. 7 - (Find the number of uppercase letters in a string)...Ch. 7 - (Game: locker puzzle) A school bas 100 lockers and...Ch. 7 - (Simulation: coupon collectors problem) Coupon...Ch. 7 - (Algebra: solve quadratic equations) Write a...Ch. 7 - (Strictly identical arrays) The arrays 1ist1 and...Ch. 7 - (Identical arrays) The arrays 1ist1 and 1ist2 are...Ch. 7 - (Math: combinations) Write a program that prompts...Ch. 7 - (Game: pick four cards) Write a program that picks...Ch. 7 - (Pattern recognition: consecutive four equal...Ch. 7 - (Merge two sorted Lists) Write the following...Ch. 7 - (Partition of a list) Write the following method...Ch. 7 - Prob. 7.33PECh. 7 - (Sort characters in a string) Write a method that...Ch. 7 - (Game: hangman) Write a hangman game that randomly...Ch. 7 - (Game: Eight Queens) The classic Eight Queens...Ch. 7 - Prob. 7.37PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- An array's index type may be any form of data. Do you think this is true or not?arrow_forward2DArray method:It will allocate a block of memory which represents a two-dimension arraybased on the input values supplied by the user. Ask the user to enter the number of rows andcolumn for 2D array that the user want to manipulate. The number of rows and columns that theuser enters may or may not define a square matrix (when the number of rows equals the numberof columns). The array will have exactly rows time columns (m * n) elements. It will notcontain any extra or empty cells. Initialize the “matrix” by rows with random number between 1to 100. Pass two arguments by out reference so that you can assign the number of row andcolumns of data to the first and second arguments. This is return 2D array method afterallocating new memory for 2D array and initialize it with random value. Generate randomnumber code in C# as following: Random randnum = new Random( );int number = randnum.Next (1, 101);SwapRow method: it will rotate the data in the matrix in an up/down method such that the…arrow_forwardJAVA PROGRAM Chapter 7. PC #16. 2D Array Operations Write a program that creates a two-dimensional array initialized with test data. Use any primitive data type that you wish. The program should have the following methods: • getTotal. This method should accept a two-dimensional array as its argument and return the total of all the values in the array. • getAverage. This method should accept a two-dimensional array as its argument and return the average of all the values in the array. • getRowTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the total of the values in the specified row. • getColumnTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The method should return the…arrow_forward
- v Question Completion Status: Attach File Browse My Computer QUESTION 2 Write a Java program that creates an array of input n (get the input from the user) random integer numbers between 10 and 79 inclusive, and pass the array to a method named countAboveAverage that return integer. In this method, count the array elements that are above or equal to average and return the count. Display the returned count in main method. Finally print the n random numbers, Average, Above or equal to Average count and Below Average count. (use formula: n- countAboveAverage) Here is a sample run: Enter the value of n: 10 Random numbers: 11 20 31 10 19 79 71 51 33 41 Average : 36.6 Above or equal to Average : 4 Below Average : 6 Attach File Browse My Computer Activate Win Savo All Answors Click Save and Submit to save and submit. Click Save All Answers to save all answers.arrow_forwardShift Left k Cells Consider an array named source. Write a method/function named shiftLeft( source, k) that shifts all the elements of the source array to the left by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly. Example: source=[10,20,30,40,50,60] shiftLeft(source,3) After calling shiftLeft(source,3), printing the array should give the output as: [ 40, 50, 60, 0, 0, 0 ]arrow_forwardBubble Sorting an Array of ObjectsCreate a Student class and you will need to do the following: Enter the number of students (must be greater than 5). Enter the student’s names and grades Make sure that you enter the names and grades in random order. Iterate through the array of students and using the bubble sort, order the array by grade.arrow_forward
- Input Parameters: sea: an array of integers indices': an array of integers (each of which may or may not be a valid index for sea") Assumptions: sea may be empty. indices may be empty. *indices may contain duplicates. What to return? - For each integer value in 'indices', if it is a valid index for input array ssa', then use it to index into 'sea' and include the result in the output array. For example: Say ssa' is (23, 46, 69} and indices' is (2, -1, 0, 3, 0, 2} (where indices -1 and 3 are invalid, so only indices 2, 0, 0, 2 are used) Then the method should return (69, 23, 23, 69} Note: Order of elements in the output array corresponds to the order in which valid indices in the 'indices' array are arranged. That is, the output array may be empty if the input array sea is empty, or if the input array indices does not contain any valid indices. See the JUnit tests related to this method. */ public static int () task1(int ) seq, int () indices) { int (] result - null; return result;arrow_forwardint Marks [20] = { 70,85, 60, 55,90,100,50}; For the above code, answer the following questions in the space provided below: 1. Find the size of Marks array. 2. How many number of elements are present in Marks array ? 3. Find the index value for last element in array 4. Find the index value for the element 60 5. Find the value of Marks[2] + Marks[0] in Marks array.arrow_forwardHow to return a new array object that is not mutated?arrow_forward
- 2D arrays (matrices/grids) Write a program that takes 20 student objects and stores them in an array. Each Student object should have a first name and a last name. Sort the array by student’s last name. You can use any of the sorts you have. Then put the names into a 2D array that has 5 rows and 4 columns. Create 2 seating charts by printing the 2D array. Student 17 Student 18 Student 19 Student 20 Student 13 Student 14 Student 15 Student 16 Student 9 Student 10 Student 11 Student 12 Student 5 Student 6 Student 7 Student 8 Student 1 Student 2 Student 3 Student 4 Student 5 Student 10 Student 15 Student 20 Student 4 Student 9 Student 14 Student 19 Student 3 Student 8 Student 13 Student 18 Student 2 Student 7 Student 12 Student 17 Student 1 Student 6 Student 11 Student 16arrow_forward1. Shift Left k Cells Use Python Consider an array named source. Write a method/function named shiftLeft( source, k) that shifts all the elements of the source array to the left by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly. Example: source=[10,20,30,40,50,60] shiftLeft(source,3) After calling shiftLeft(source,3), printing the array should give the output as: [ 40, 50, 60, 0, 0, 0 ]arrow_forwardAn array definition reserves space for the array. true or falsearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
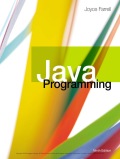
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
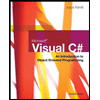
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage