JAVA PROGRAM Chapter 7. PC #16. 2D Array Operations Write a program that creates a two-dimensional array initialized with test data. Use any primitive data type that you wish. The program should have the following methods: • getTotal. This method should accept a two-dimensional array as its argument and return the total of all the values in the array. • getAverage. This method should accept a two-dimensional array as its argument and return the average of all the values in the array. • getRowTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the total of the values in the specified row. • getColumnTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The method should return the total of the values in the specified column. • getHighestInRow. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the highest value in the specified row of the array. • getLowestInRow. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the lowest value in the specified row of the array. Demonstrate each of the methods in this program. The name of the file is read from command-line arguments: args[0] Inpu11.txt 1 2 3 4 5 6 7 8 9 Input2.txt 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 Input3.txt 555.66 333.44 123.45 555.66 333.44 123.45 778.88 900.00 123.12 778.88 900.00 123.12 234.23 567.98 12.12 234.23 567.98 12.12 Input4.txt 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 Test Case 1 Command Line arguments: input1.txt Row 0 Subtotal: 6.000\n Row 1 Subtotal: 15.000\n Row 2 Subtotal: 24.000\n Column 0 Subtotal: 12.000\n Column 1 Subtotal: 15.000\n Column 2 Subtotal: 18.000\n Array Total: 45.000\n Test Case 2 Command Line arguments: input2.txt Row 0 Subtotal: 1012.550\n Row 1 Subtotal: 1802.000\n Row 2 Subtotal: 814.330\n Column 0 Subtotal: 1568.770\n Column 1 Subtotal: 1801.420\n Column 2 Subtotal: 258.690\n Array Total: 3628.880\n Test Case 3 Command Line arguments: input3.txt Row 0 Subtotal: 2025.100\n Row 1 Subtotal: 3604.000\n Row 2 Subtotal: 1628.660\n Column 0 Subtotal: 1568.770\n Column 1 Subtotal: 1801.420\n Column 2 Subtotal: 258.690\n Column 3 Subtotal: 1568.770\n Column 4 Subtotal: 1801.420\n Column 5 Subtotal: 258.690\n Array Total: 7257.760\n Test Case 4 Command Line arguments: input4.txt Row 0 Subtotal: 1012.550\n Row 1 Subtotal: 1802.000\n Row 2 Subtotal: 814.330\n Row 3 Subtotal: 1012.550\n Row 4 Subtotal: 1802.000\n Row 5 Subtotal: 814.330\n Row 6 Subtotal: 1012.550\n Row 7 Subtotal: 1802.000\n Row 8 Subtotal: 814.330\n Column 0 Subtotal: 4706.310\n Column 1 Subtotal: 5404.260\n Column 2 Subtotal: 776.070\n Array Total: 10886.640\n
JAVA PROGRAM Chapter 7. PC #16. 2D Array Operations Write a program that creates a two-dimensional array initialized with test data. Use any primitive data type that you wish. The program should have the following methods: • getTotal. This method should accept a two-dimensional array as its argument and return the total of all the values in the array. • getAverage. This method should accept a two-dimensional array as its argument and return the average of all the values in the array. • getRowTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the total of the values in the specified row. • getColumnTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The method should return the total of the values in the specified column. • getHighestInRow. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the highest value in the specified row of the array. • getLowestInRow. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the lowest value in the specified row of the array. Demonstrate each of the methods in this program. The name of the file is read from command-line arguments: args[0] Inpu11.txt 1 2 3 4 5 6 7 8 9 Input2.txt 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 Input3.txt 555.66 333.44 123.45 555.66 333.44 123.45 778.88 900.00 123.12 778.88 900.00 123.12 234.23 567.98 12.12 234.23 567.98 12.12 Input4.txt 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 Test Case 1 Command Line arguments: input1.txt Row 0 Subtotal: 6.000\n Row 1 Subtotal: 15.000\n Row 2 Subtotal: 24.000\n Column 0 Subtotal: 12.000\n Column 1 Subtotal: 15.000\n Column 2 Subtotal: 18.000\n Array Total: 45.000\n Test Case 2 Command Line arguments: input2.txt Row 0 Subtotal: 1012.550\n Row 1 Subtotal: 1802.000\n Row 2 Subtotal: 814.330\n Column 0 Subtotal: 1568.770\n Column 1 Subtotal: 1801.420\n Column 2 Subtotal: 258.690\n Array Total: 3628.880\n Test Case 3 Command Line arguments: input3.txt Row 0 Subtotal: 2025.100\n Row 1 Subtotal: 3604.000\n Row 2 Subtotal: 1628.660\n Column 0 Subtotal: 1568.770\n Column 1 Subtotal: 1801.420\n Column 2 Subtotal: 258.690\n Column 3 Subtotal: 1568.770\n Column 4 Subtotal: 1801.420\n Column 5 Subtotal: 258.690\n Array Total: 7257.760\n Test Case 4 Command Line arguments: input4.txt Row 0 Subtotal: 1012.550\n Row 1 Subtotal: 1802.000\n Row 2 Subtotal: 814.330\n Row 3 Subtotal: 1012.550\n Row 4 Subtotal: 1802.000\n Row 5 Subtotal: 814.330\n Row 6 Subtotal: 1012.550\n Row 7 Subtotal: 1802.000\n Row 8 Subtotal: 814.330\n Column 0 Subtotal: 4706.310\n Column 1 Subtotal: 5404.260\n Column 2 Subtotal: 776.070\n Array Total: 10886.640\n
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.4: Arrays As Arguments
Problem 6E: (Electrical eng.) Write a program that declares three one-dimensional arrays named volts, current,...
Related questions
Question
100%
JAVA PROGRAM
Chapter 7. PC #16. 2D Array Operations
Write a program that creates a two-dimensional array initialized with test data. Use any primitive data type that you wish. The program should have the following methods:
• getTotal. This method should accept a two-dimensional array as its argument and return the total of all the values in the array.
• getAverage. This method should accept a two-dimensional array as its argument and return the average of all the values in the array.
• getRowTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the total of the values in the specified row.
• getColumnTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The method should return the total of the values in the specified column.
• getHighestInRow. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the
subscript of a row in the array. The method should return the highest value in the specified row of the array.
• getLowestInRow. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the lowest value in the specified row of the array.
Demonstrate each of the methods in this program. The name of the file is read from command-line arguments: args[0]
Inpu11.txt
1 2 3
4 5 6
7 8 9
4 5 6
7 8 9
Input2.txt
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
778.88 900.00 123.12
234.23 567.98 12.12
Input3.txt
555.66 333.44 123.45 555.66 333.44 123.45
778.88 900.00 123.12 778.88 900.00 123.12
234.23 567.98 12.12 234.23 567.98 12.12
778.88 900.00 123.12 778.88 900.00 123.12
234.23 567.98 12.12 234.23 567.98 12.12
Input4.txt
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
778.88 900.00 123.12
234.23 567.98 12.12
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
Test Case 1
input1.txt
Row 0 Subtotal: 6.000\n
Row 1 Subtotal: 15.000\n
Row 2 Subtotal: 24.000\n
Column 0 Subtotal: 12.000\n
Column 1 Subtotal: 15.000\n
Column 2 Subtotal: 18.000\n
Array Total: 45.000\n
Row 1 Subtotal: 15.000\n
Row 2 Subtotal: 24.000\n
Column 0 Subtotal: 12.000\n
Column 1 Subtotal: 15.000\n
Column 2 Subtotal: 18.000\n
Array Total: 45.000\n
Test Case 2
input2.txt
Row 0 Subtotal: 1012.550\n
Row 1 Subtotal: 1802.000\n
Row 2 Subtotal: 814.330\n
Column 0 Subtotal: 1568.770\n
Column 1 Subtotal: 1801.420\n
Column 2 Subtotal: 258.690\n
Array Total: 3628.880\n
Row 1 Subtotal: 1802.000\n
Row 2 Subtotal: 814.330\n
Column 0 Subtotal: 1568.770\n
Column 1 Subtotal: 1801.420\n
Column 2 Subtotal: 258.690\n
Array Total: 3628.880\n
Test Case 3
input3.txt
Row 0 Subtotal: 2025.100\n
Row 1 Subtotal: 3604.000\n
Row 2 Subtotal: 1628.660\n
Column 0 Subtotal: 1568.770\n
Column 1 Subtotal: 1801.420\n
Column 2 Subtotal: 258.690\n
Column 3 Subtotal: 1568.770\n
Column 4 Subtotal: 1801.420\n
Column 5 Subtotal: 258.690\n
Array Total: 7257.760\n
Row 1 Subtotal: 3604.000\n
Row 2 Subtotal: 1628.660\n
Column 0 Subtotal: 1568.770\n
Column 1 Subtotal: 1801.420\n
Column 2 Subtotal: 258.690\n
Column 3 Subtotal: 1568.770\n
Column 4 Subtotal: 1801.420\n
Column 5 Subtotal: 258.690\n
Array Total: 7257.760\n
Test Case 4
input4.txt
Row 0 Subtotal: 1012.550\n
Row 1 Subtotal: 1802.000\n
Row 2 Subtotal: 814.330\n
Row 3 Subtotal: 1012.550\n
Row 4 Subtotal: 1802.000\n
Row 5 Subtotal: 814.330\n
Row 6 Subtotal: 1012.550\n
Row 7 Subtotal: 1802.000\n
Row 8 Subtotal: 814.330\n
Column 0 Subtotal: 4706.310\n
Column 1 Subtotal: 5404.260\n
Column 2 Subtotal: 776.070\n
Array Total: 10886.640\n
Row 1 Subtotal: 1802.000\n
Row 2 Subtotal: 814.330\n
Row 3 Subtotal: 1012.550\n
Row 4 Subtotal: 1802.000\n
Row 5 Subtotal: 814.330\n
Row 6 Subtotal: 1012.550\n
Row 7 Subtotal: 1802.000\n
Row 8 Subtotal: 814.330\n
Column 0 Subtotal: 4706.310\n
Column 1 Subtotal: 5404.260\n
Column 2 Subtotal: 776.070\n
Array Total: 10886.640\n
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
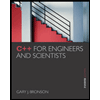
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
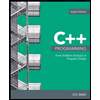
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
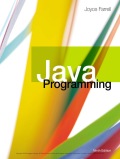
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
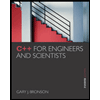
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
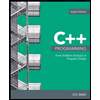
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
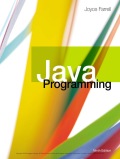
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
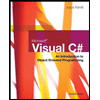
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
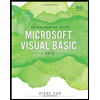
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning