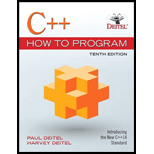
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7, Problem 7.31RE
Program Plan Intro
Program Plan:
- Int a[] used as a input array
- Int n is a size of an array
- Minvaluefunction used to find the minimun value
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(Random sentences and story writer) Write an app that uses random-number generation to create sentences. Use four arrays of strings, called article, noun, verb andprepostion. Create a sentence by selecting a word at random from each array in the following order: article, noun, verb, preposition, article, noun.As each word is picked, concatenate it to the previous words in the sentence. The words should be separated by spaces. When the sentence is output, it should start witha capital letter and end with a period. The program should generate 10 sentences and output them to a text box. The arrays should be filled as follows:The article array should contain the articles "the", "a", "one", "some" and "any";The noun array should contain the nouns "boy", "girl", "dog", "town", "car";The verb array should contain the past tense verbs "drove", "jumped", "ran", "walked" and "skipped";The preposition array should contain the preposotions "to", "from", "over", "under" and "on";
(Recursive Greatest Common Divisor) The greatest common divisor of integers x and y isthe largest integer that evenly divides both x and y. Write a recursive function gcd that returns thegreatest common divisor of x and y. The gcd of x and y is defined recursively as follows: If y is equalto 0, then gcd(x, y) is x; otherwise gcd(x, y) is gcd(y, x % y), where % is the remainder operator.
(Recursion and Backtracking)
Write the pseudo code for a recursive method called addB2D that takes two binary numbers as strings, adds the numbers and returns the result as a decimal integer. For example, addB2D(‘‘101’’, ‘‘11’’) should return 8 (because the strings are binary representation of 5 and 3, respectively).
Your solution should not just simply convert the binary numbers to decimal numbers and add the re- sults. An acceptable solution tries to find the sum by just directly processing the binary representations without at first converting them to decimal values.
Chapter 7 Solutions
C++ How to Program (10th Edition)
Ch. 7 - Exercises 7.6(Fill in the Blanks) Fill in the...Ch. 7 - (True or False) Determine whether each of the...Ch. 7 - (Write C++ Statements) Write C++ statements to...Ch. 7 - (Two-Dimensional array Questions) Consider a...Ch. 7 - (Salesperson Salary Ranges) Use a one-dimensional...Ch. 7 - (One-Dimensional array Questions) Write statements...Ch. 7 - (Find the Errors) Find the error(s) in each of the...Ch. 7 - (Duplicate Elimination with array) Use a...Ch. 7 - Prob. 7.14ECh. 7 - Prob. 7.15E
Ch. 7 - (Dice Rolling) Write a program that simulates...Ch. 7 - ( What Does This Code Do?) What does the following...Ch. 7 - (Craps Game Modification) Modify the program of...Ch. 7 - (Converting vector Example of Section 7.10 to...Ch. 7 - Prob. 7.20ECh. 7 - (Sales Summary) Use a two-dimensional array to...Ch. 7 - (Knight's Tour) One of the more interesting...Ch. 7 - (Knight's Tour: Brute Forty Approaches ) In...Ch. 7 - (Eight Queens) Another puzzler for chess buffs is...Ch. 7 - (Eight Queens: Brute Force Approaches) In this...Ch. 7 - Prob. 7.26ECh. 7 - (The Sieve of Eratosthenes) A prime integer is any...Ch. 7 - Prob. 7.28RECh. 7 - (Eight Queens) Modify the Eight Queens program you...Ch. 7 - (Print an array) Write a recursive function...Ch. 7 - Prob. 7.31RECh. 7 - Prob. 7.32RECh. 7 - (Maze Traversal) The grid of hashes (#) and dots...Ch. 7 - Prob. 7.34RECh. 7 - Making a Difference 7.35 (Polling) The Internet...
Knowledge Booster
Similar questions
- (Find the index of the largest element) Write a function that returns the index of the largest element in an array of integers. If there are more such elements than one, return the largest index. Use the following header: int indexOfLargestElement(double array[], int size)arrow_forwardQuestion 4: (Find the minimum value in an array) Write a program that include a recursive function "recursiveMinimnm" that takes an integer array and the array size as arguments and returns the smallest element of the array. The function should stop processing and return when it receives an array of one element. Answer (2.5)arrow_forward(Recursive Binary Search) Write a recursive method RecursiveBinarySearch to perform abinary search of the array. The method should receive the search key, starting index, endingindex and array A as arguments. If the search key is found, return its index in the array. If thesearch key is not found, return -1.int RecursiveBinarySearch(int search, int start, int end, int[] A)arrow_forward
- (Replace strings) Write the following function that replaces the occurrence of a substring old_substring with a new substring new_substring in the string s. The function returns true if string s is changed, and otherwise, it returns false. bool replace_strings (string& s, const string& old_string, const string& new_string) Write a test program that prompts the user to enter three strings, i.e., s, old string, and new_string, and display the replaced string.arrow_forward4. CodeW X For func X C Solved b Answer x+ https://codeworkou... ... [+) CodeWorkout X271: Recursion Programming Exercises: Minimum of array For function recursiveMin, write the missing part of the recursive call. This function should return the minimum element in an array of integers. You should assume that recursiveMin is initially called with startIndex = 0. Examples: recursiveMin({2, 4, 8}, 0) -> 2 Your Answer: 1 public int recursiveMin(int numbers[], int startIndex) { numbers.length - 1) { if (startIndex 2. return numbers[startIndex]; } else { return Math. min(numbers[startIndex], >); 5. { 1:11 AM 50°F Clear 12/4/2021arrow_forwardstate the statement either true or false.arrow_forward
- (Check test scores) The answers to a true-false test are as follows: T T F F T. Given a two-dimensional answer array, in which each row corresponds to the answers provided on one test, write a function that accepts the two-dimensional array and number of tests as parameters and returns a one-dimensional array containing the grades for each test. (Each question is worth 5 points so that the maximum possible grade is 25.) Test your function with the following data: int score = 0;arrow_forward(True/False): A segment descriptor does not contain segment size informationarrow_forward- array from user and print all the elements after doubuq have to separate function for doubling operation. 2arrow_forward
- Exercise 1: (Design of algorithm to find greatest common divisor) In mathematics, the greatest common divisor (gcd) of two or more integers is the largest positive integer that divides each of the integers. For example, the gcd of 8 and 12 is 4. Why? Divisors of 8 are 1, 2, 4, 8. Divisors of 12 are 1, 2, 4, 6, 12 Thus, the common divisors of 8 and 12 are 1, 2, 4. Out of these common divisors, the greatest one is 4. Therefore, the greatest common divisor (gcd) of 8 and 12 is 4. Write a programming code for a function FindGCD(m,n) that find the greatest common divisor. You can use any language of Java/C++/Python/Octave. Find GCD Algorithm: Step 1 Make an array to store common divisors of two integers m, n. Step 2 Check all the integers from 1 to minimun(m,n) whether they divide both m, n. If yes, add it to the array. Step 3 Return the maximum number in the array.arrow_forwardProgramming Language C Note:No Need for Detailed Explanation. The Answer is Enough For Me.Solve according to this information. (No: 2012010206083)arrow_forwardCorrect my mistake in my C++ code please! Here is the question and my code is below as well. (Duplicate Elimination with array) Use a one-dimensional array to solve the following problem. Read in 20 numbers, each of which is between 10 and 100, inclusive. As each number is read, validate it and store it in the array only if it isn't a duplicate of a number already read. After reading all the values, display only the unique values that the user entered. Provide for the 'worst case' in which all 20 numbers are different. Use the smallest possible array to solve this problem. Code; #include <iostream> using namespace std; int main(){ int arr[100]={0};int temp,i;for( i=0;i<20;i++){ cout<<"Enter an Integer : "; cin>>temp; arr[temp]++;}for( i=0;i<100;i++) if(arr[i]==1) cout<<i<<" "; return 0;}arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
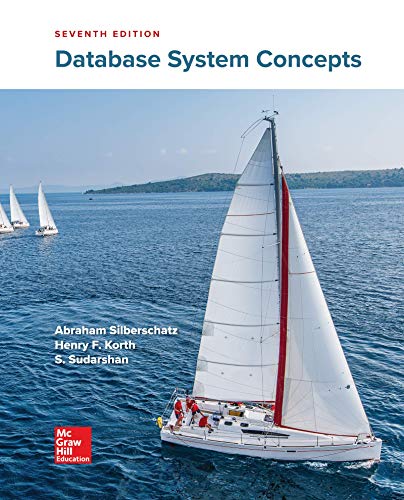
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
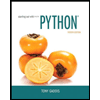
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
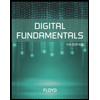
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
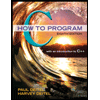
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
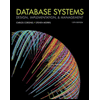
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
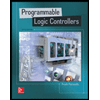
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education