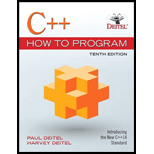
(Knight's Tour) One of the more interesting puzzlers for chess buffs is the Knight's Tour
problem. The question is this: Can the chess piece called the knight move around an empty chess-
board and touch each of the 64 squares once and only once? We study this intriguing problem in
depth in this exercise.
The knight makes L-shaped moves (over two in one direction then over one in a perpendicular
direction). Thus, from a square in the middle of an empty chessboard. the knight can make
eight different moves (numbered 0 through 7) as shown in Fig. 7.25.
- Draw an 8-by-8 chessboard on a sheet of paper and attempt a Knight's Tour by hand' Put a 1 in the first square you move to. a 2 in the second square, A 3 in the third,
- Now let's develop a
program that will move the knight around a chessboard. The board Is represented by an 8-by-8 two-dimensional array board. Each of the squares is initialized
Before starting the tour, estimate how far you think you'll get, remembering that a full
tour consists of 64 moves. How Ear did you get? Was this close to your estimate?
zero. We describe each of the eight possible moves in terms of both their horizontal
and vertical components. For example, a move of type 0. as shown in Fig, 7.25,
consists of moving two squares horizontally to the right and one square vertically up-
ward. Move 2 consists of moving one square horizontally to the left and squares
0 1 2 3 4 5 6 7 0 1 2 1 2 3 0 3 K 4 4 7 5 5 6 6 7
Fig.7.25 The eight possible moves of the knight
Vertically upward. Horizontal moves to the left and vertical moves upward are indicated with negative numbers. The eight moves may be described by two one-dimensional arrays, horizontal and vertical, as follows:
Horizontal [0] =2 vertical [0] = 1 Horizontal [1] = 1 vertical [1] = 2 Horizontal [2] =-1 vertical [2] = -2 Horizontal [3] = -2 vertical [3] = -1 Horizontal [4] = -2 vertical [4] = 1 Horizontal [5] = -1 vertical [5] = 2 Horizontal [6] = 1 vertical [6] = 2 Horizontal [7]= 2 vertical [7] = 1
Let the variables and currentCoIumn indicate the row and column of
the knight's current position. To make a move of type moveNumber, where is moveNumber is
between 0 and 7. your program uses the statements
CurrentRow += vertical [moveNumber];
currentColumn += horizontal [moveNumber];
Keep a counter that varies I to 64. Record latest in each square the knight moves to. Remember to test cach potential move to see if the knight has already visited that square, and of course, test every potential move to make sure that the knight does not land off the chessboard. Now "Tite a program to move the knight around the chessboard. Run the program. How many moves did the knight make?
c) After attempting to write and run a Knight's Tour program. you've probablv developed some value Insights. We'll use these develop a heuristic (or strategy) for moving the knight. Heuristics do not guarantee success, but a carefully developed heuristic greatly improves the chance of success. You may have observed that the outer squares are more troublesome than the squares nearer the center of the board. In fact, the most troublesome, or inaccessible, squares arc the four corners.
Intuition may suggest that you should attempt to move the knight to the most troublesome squares first and leave open those that easiest to get to, so when the board gets congested near the end of the tour. there will be a greater chance of success.
We may may develop an "accessibility heuristic" by classifying each square according to how accessible it's then always moving the knight to the square (within the knight's L shaped moves, of course) that's least accessible. We label a two-dimensional array accessibility with numbers indicating from how many squares each particular square is accessible. On a blank chessboard, each center square is rated as 8, each corner square is rated as 2 and the other squares have accessibility numbers of 3,4 or 6 as follows
-
2 3 4 4 4 4 3 2
3 4 6 6 6 6 4 3
4 6 8 8 8 8 6 4
4 6 8 8 8 8 6 4
4 6 8 8 8 8 6 4
4 6 8 8 8 8 6 4
3 4 6 6 6 6 4 3
2 3 4 4 4 4 3 2
Now write a version of the Knight's Tour program using the accessibility heuristic.
At any time, the knight should move to the square with the lowest accessibility num ber. In case of a tie, the knight may move to any of the tied squares. Therefore, the tour may begin in any of the four corners. [Note: As the knight moves around the chess- board, your program should reduce the accessibility numbers as more and more Squares become occupied. In this way, at any given time during the tour, each available square's accessibility number will remain equal to precisely the number of squares from which that square may be reached.] Run this version of your program. Did you get a full tour? Now modify the program to run 64 tours, one starting from each square of the chessboard. How many full tours did you get?
d.Write a version of the Knight's Tour program Which, When encountering a tie between two or more Squares, decides what square to choose by looking ahead to those squares reachable from the "tied" squares. Your program should move to the square for which the next move would arrive at a square with the lowest accessibility number.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
C++ How to Program (10th Edition)
- can you give me the code to the folloowing?arrow_forwardCan you help me trying to do this code because I am struggling big time with this. question that i need help with: the Eight Puzzle consists of a 3 x 3 board of sliding tiles with a single empty space. For each configuration, the only possible moves are to swap the empty tile with one of its neighboring tiles. The goal state for the puzzle consists of tiles 1-3 in the top row, tiles 4-6 in the middle row, and tiles 7 and 8 in the bottom row, with the empty space in the lower-right corner.you will develop two solvers for a generalized version of the Eight Puzzle, in which the board can have any number of rows and columns. A natural representation for this puzzle is a two-dimensional list of integer values between 0 and r · c -1 (inclusive), where r and c are the number of rows and columns in the board, respectively. In this problem, we will adhere to the convention that the 0-tile represents the empty space.tasks:In the TilePuzzle class, write an initialization method __init__(self,…arrow_forwardOne variation on the game of nim is described in Luger. The game begins with a single pile of stones. The move by a player consists of dividing a pile into two piles that contain an unequal number of stones. For example, if one pile contains six stones, it could be subdivided into piles of five and one, or four and two, but not three and three. The first player who cannot make a move loses the game. (5.1) Draw the complete game tree for this version of Nim if the start state consists of six stones. (5.2) Perform a minimax evaluation for this game. Let 1 denote a win and 0 a loss.arrow_forward
- Idiot’s Delight is a fairly simple game of solitaire, yet it is difficult to win. The goal is to draw all of the cards from the deck, and end up with no cards left in your hand. You will run through the deck of cards one time. Start by dealing 4 cards to your hand. You will always look at the last 4 cards in your hand. If the ranks of the “outer” pair (1st and 4th) are the same, discard all four cards. Otherwise, if the suits of the “inner” pair (2nd and 3rd) are the same, discard those 2 cards only. If you have less than 4 cards, draw enough to have 4 cards in your hand. If the deck is empty, the game is over. Your score will be the number of cards that remain in your hand. Like in golf, the lower the score the better. Create a new Python module in a file named “idiots_delight.py”. Add a function called deal_hand that creates a standard deck of cards, deals out a single hand of 4 cards and returns both the hand and the deck. Remember that for the last assignment, you created several…arrow_forwardPython Programing: Sahil is an outstanding entertainer. He is expert in what he does. At a day, he accepted Samir's challenge to plant 20 million trees by 2020. Currently, there are N trees (numbers 1 to N) planted in different places in the row; for each valid I, the location of the i-Ayi tree. A bunch of trees is good if for each tree in this set (let's say its place in x), there is a tree in x - 1 and / or a tree in x + 1. Sahil task is to plant more trees (perhaps zero) in such a way that the effect of all the trees planted (the first N trees and the ones planted by Sahil) is good. It is only allowed to plant trees in complete (perhaps unfavorable) areas. Help Sahil to find the minimum number of trees they need to plant to achieve that using Python Programming. Input: 1 3 538 Output: 3.arrow_forward[using C++]Masterchef pankaj recently baked a big nepotialn pizza that can be represented as a grid of N rows and M columns, each cell can be either empty or contain a jalapeno, pankaj wants to cut out a sub-rectangle from the pizza which contains even number of jalapenos. Before cutting such a sub-rectangle, he is interested in knowing how many sub-rectangles are there which contains even number of jalapenos.First line of input consist of two integers P and Q. Each of the next P lines contains a string of length Q, j-th character of i-th string is 1 if the corresponding cell contains a jalapeno otherwise it's 0.Output a single integer, the number of sub rectangles which contains even number of jalapeno.Sample Input:2 22101Output:5arrow_forward
- Correct answer will be upvoted else Multiple Downvoted. Don't submit random answer. Computer science. anglers have recently gotten back from a fishing excursion. The I-th angler has gotten a fish of weight man-made intelligence. Anglers will flaunt the fish they got to one another. To do as such, they initially pick a request where they show their fish (every angler shows his fish precisely once, in this way, officially, the request for showing fish is a stage of integers from 1 to n). Then, at that point, they show the fish they discovered by the picked request. At the point when an angler shows his fish, he may either become glad, become dismal, or stay content. Assume an angler shows a fish of weight x, and the most extreme load of a formerly shown fish is y (y=0 if that angler is quick to show his fish). Then, at that point: in the event that x≥2y, the angler becomes cheerful; in the event that 2x≤y, the angler becomes miserable; in the event that none of these two…arrow_forwardCorrect answer will be upvoted else Multiple Downvoted. Computer science. you can choose two indices x and y (x≠y) and set ax=⌈axay⌉ (ceiling function). Your goal is to make array a consist of n−1 ones and 1 two in no more than n+5 steps. Note that you don't have to minimize the number of steps. Input The first line contains a single integer t (1≤t≤1000) — the number of test cases. The first and only line of each test case contains the single integer n (3≤n≤2⋅105) — the length of array a. It's guaranteed that the sum of n over test cases doesn't exceed 2⋅105. Output For each test case, print the sequence of operations that will make a as n−1 ones and 1 two in the following format: firstly, print one integer m (m≤n+5) — the number of operations; next print m pairs of integers x and y (1≤x,y≤n; x≠y) (x may be greater or less than y) — the indices of the corresponding operation. It can be proven that for the given constraints it's always possible to find a correct sequence…arrow_forwardKnight's Tour: The Knight's Tour is a mathematical problem involving a knight on a chessboard. The knight is placed on the empty board and, moving according to the rules of chess, must visit each square exactly once. There are several billion solutions to the problem, of which about 122,000,000 have the knight finishing on the same square on which it begins. When this occurs the tour is said to be closed. Your assignment is to write a program that gives a solution to the Knight's Tour problem recursively. You must hand in a solution in C++ AND Java. The name of the C++ file should be "main.cc" and the name of the Java file should be "Main.java". Write C++ only with a file name of main.cc Please run in IDE and check to ensure that there are no errors occuring Output should look similar to: 1 34 3 18 49 32 13 16 4 19 56 33 14 17 50 31 57 2 35 48 55 52 15 12 20 5 60 53 36 47 30 51 41 58 37 46 61 54 11 26 6 21 42 59 38 27 64 29 43 40 23 8 45 62 25 10 22 7 44 39 24 9 28 63arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
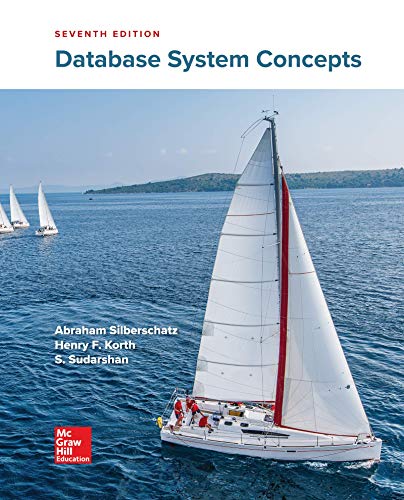
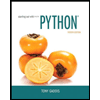
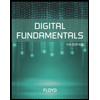
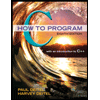
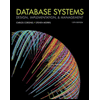
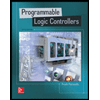