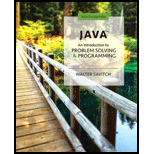
Add a method insertionSort to the class ArraySorter, as given in Listing 7.10, mat performs an insertion sort of an array. To simplify this protect, our insertion sort
Inserting into a sorted array
Insertion sort algorithm to sort an array
for (index = 0; index <a.length; index++)
Insert the value of a [index] into its correct position in the array temp, so that all the elements copied into the array temp so far are sorted.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Database Concepts (8th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- Min Heap of Candy — Add and Remove Using the provided code (Question04.java and Candyjava), write the add and remove methods for a min heap of Candy using an array implementation. Make sure to include all provided files in your project and write your solution in the file "Question04.java". This method should preserve the properties of a min hap, and the candies are compared based on their weight. This value can be accessed through its accessor. Solution Tests: • Does the solution compile? • Does the solution have your name in the comments? • Does the solution have a high-level solution description (150-300 words) in the comments? • Does the method preserve the properties of a Min Heap? • Does the output match the following? Testing the Add Method Candy Weight: 10.0, Candy Weight: 40.0, Candy Weight: 20.0, Candy Weight: 50.0, Candy Weight: 60.0, Candy Weight: 80.0, Candy Weight: 30.0, Candy Weight: 100.0, Candy Weight: 70.0, Candy Weight: 90.0, Testing the Remove Method Candy Weight:…arrow_forwardWrite in java code Create an array myArr of 10 integer elements and initialize/fill it with numbers (not sorted) between 0 and 20; for example myArr = [ 12, 3, 19, 5, 7, 11,….etc.]. (a) print the array. (b) Use method sort() of class Arrays to sort myArr and print it after sorting. (c) Use the arraycopy() method of class System to copy myArr to another array called my2ndArr, then print both arrays. (d) use method equals() of class Arrays between the two arrays and print the results. (e) use method fill() to fill my2ndArr with 99 in all elements and then use method equals()and print the result whether the two arrays are equal or not. (f) use the method binarySearch() to search myArr for the value 15 and print the resultarrow_forwardWrite a Java program tat prompts user for a list of integers with 0 as the last value.Save the values in an array. Assume there can be maximum 100 values. Theprogram should have the following methods:- A method that takes the array as parameter and updates each value in thearray to the square of the value.- Another method that takes the original and modified arrays as parametersand displays the original and the squared values.arrow_forward
- Plz answer in java onlyarrow_forwardDefine a class Car as follows:class Car { public String make; public String model; public int mpg;// Miles per gallon}a) Implement a comparator called CompareCarsByMakeThenModel that can be passed as an argument to the quicksort method from the lecture notes. CompareCarsByMakeThenModel should return a value that will cause quicksort to sort an array of cars in ascending order (from smallest to largest) by make and, when two cars have the same make, in ascending order by model.b) Implement a comparator called CompareCarsByDescendingMPG that can be passed as an argument to the quicksort method from the lecture notes. CompareCarsByDescendingMPG should return a value that will cause quicksort to sort an array of cars in descending order (from largest to smallest) by mpg.c) Implement a comparator called CompareCarsByMakeThenDescendingMPG that can be passed as an argument to the quicksort method from the lecture notes.…arrow_forwardjava code that completes tasks forr an array integers. Swaped the first and last elements in array. Replace all even elements with 0. Shift all elements by one to the right and move the last element into the first position. For example, 1 4 9 16 25 would be turned i into 25 1 4 9 16. Replace each element except first and last the larger of its two neighbors.arrow_forward
- Solve it quick in javaarrow_forwardIntellij - Java Write a program which does the following: Create a class with the main() method. Take a string array input from the user in main() method. Note: Do not hardcode the string array in code. After the String array input loop, the array should be: words[] = {"today", "is", "a", "lovely", "spring","day", "not", "too", "hot", "not", "too", "cold"}; Print the contents of this array using Arrays.toString() method. Write a method called handleString() that performs the following: Takes a String[] array as method parameter (similar to main method). Loop through the array passed as parameter, and for each word in the array: If the length of the word is even, get the index of the last letter & print that index. Use length() and indexOf() String object methods. If the string length is odd, get the character at the 1st position in the string & print that letter. Use length() and charAt() String object methods.arrow_forwardI want solution with stepsarrow_forward
- Note: Using Arrays In Java Language.arrow_forwardGiven an array of length N and an integer x, you need to find all the indexes where x is present in the input array. Save all the indexes in an array (in increasing order). Do this recursively. Indexing in the array starts from 0. Input Format : Line 1: An Integer Ni.e. size of array Line 2: N integers which are elements of the array, separated by spaces Line 3: Integer x Output Format : indexes where x is present in the array (separated by space) Constraints: 1 <= N <= 10^3 Sample Input: 5 981088 8 Sample Output : 134 Solution:///// public class Solution { public static int[] allIndexes (int input[], int x, int startIndex) { if(startIndex==input.length) { int output[] = new int[0]; return output; } int smallOutput[] = allIndexes (input,x, startIndex+1); if(input[startIndex]==x){ int output[] = new int[smallOutput.length +1]; } } output[0] = startIndex; for(int i=0;iarrow_forwardExtend the Array class adding the following two newmethods:A) the method called min that returns the smallest numberin the array.E.g., applying min to the array [−3, 2, 0, −10, 9, 7], we get −10.B) the method called reverse that reverses the array.E.g., applying reverse to the array [−3, 2, 0, −10, 9, 7], we change itto [7, 9, −10, 0, 2, −3]. USE THE JAVA CODE BELOW TO ADD ON PLEASE public class Array {private int[] elems;private int nElems;public Array(int size) {if (size < 0) {throw new IllegalArgumentException();}elems = new int[size];}public void print() {System.out.print("[");for (int i = 0; i < nElems; ++i)if (i < nElems - 1) {System.out.print(elems[i] + ", ");} else {System.out.print(elems[i]);}System.out.println("]");}public void insert(int element) {// if the array is full, increases its sizeif (elems.length == 0) {int[] extendedElems = new int[1];elems = extendedElems;}if (elems.length == nElems) {int[] extendedElems = new int[nElems * 2];for (int i = 0; i <…arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
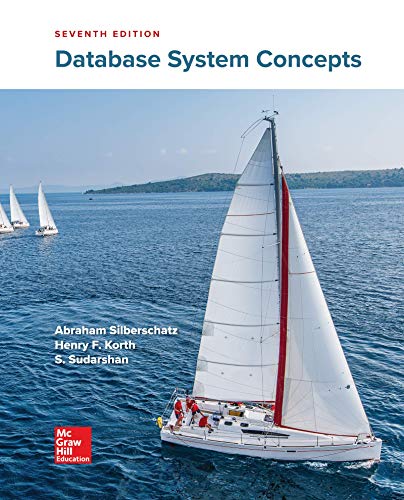
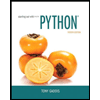
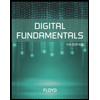
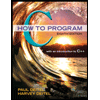
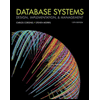
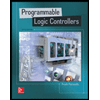