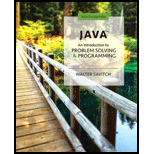
Suppose that we are selling boxes of candy for a fund-raiser. We have five kinds of candy to sell: Mints, Chocolates with Nuts, Chewy Chocolates, Dark Chocolate Creams, and Sugar-Free Suckers. We will record a customer’s order as an array of five integers, representing the number of boxes of each Kind of candy. Write a static method combineorder that takes two orders as its arguments and returns an array that represents the combined orders. For example, if order1 contains 0, 0, 3, 4, and 7, and order2 contains 0, 4, 0, 1, and 2, the method should return an array containing 0, 4, 3, 5, and 9.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Database Concepts (7th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Programming in C
Concepts of Programming Languages (11th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
- An element in the array is a peak (max or min) if the elements before and after that element are greater than the element itself (min) or the elements before and after that element are less than the element (max). Write a method in your program called displayPeaks that will take a single-dimensional array as parameter and display all peak elements of that array (line by line) together with their index and whether they are max or min. For example, calling the method displayPeaks from main method will display: 83 at position 0 is a max 48 at position 1 is a min 89 at position 2 is a max 40 at position 4 is a min 94 at position 5 is a max 12 at position 8 is a min 87 at position 11 is a max 10 at position 12 is a min 78 at position 14 is a max 2 at position 15 is a min 52 at position 18 is a max 46 at position 19 is a minarrow_forwardUsing Java Programing, write a RainFall class that stores the total rainfall for each of 12 months into an array of doubles. The program should have methods that return the following: the total rainfall for the year the average monthly rainfall the month with the most rain, and the month with the least rain. Demonstrate the class in a complete program. Input Validation: Do not accept negative numbers for monthly rainfall figures.arrow_forwardCreate a Golf Game. Allow for two players. Prompt for the player names. Each player plays 18 holes. Use an array or List for each player to hold their number of swings for each hole. Logical methods should be implemented for different tasks (broken out below). It is up to you to determine the parameters and return types of each method as appropriate. Method 1 - For each hole, the player has a number of swings. Determine the player's swings by generating a random number between 1 and 4. Store each players number of swings in the array/list. Method 2 - After completing the 18 holes, add up the number of swings for each hole for each player. The player with the fewest number of swings is the winner. Method 3 - Save the game data into a CSV file in the main project (root) directory. Name the file "Golf_Game_Player1_Player2.csv" using the player's names. The information in the file should look like this: playername, scoreforhole1, scoreforhole2, scoreforhole3, etc... Method 4 - In the…arrow_forward
- You are given an array of n integers. Write a java method that splitsthe numbers of the array into two equal groups so that the GCD of all numbers in the second group is equal to one and the GCD of all numbers in the first group is not one. After splitting the numbers display the resulting groups as shown in the sample run below. Note: 1. The array has even size. 2. All elements of the array are less than 100. You are not allowed to define new arrays. 3. 4. You are not allowed to definenew methods or use methods from java libraries. Sample run of the method: Inputarray: (6,7,9,4,3,2} Output Group two: (7,9,3} Group one: (2,4,6} I Ignore Order of numbers. Order is not important //Ignore Order of numbers. Order is not importantarrow_forwardUSING JAVA Write a method intToWeekDay(integer) that returns a week day if integer is belongs to {0,1,...,6} by using enumeration type. For example, calling intToWeekDay(0) returns "Sunday".arrow_forwardin java Write a RainFall class that stores the total rainfall for each of 12 months into an array ofdoubles. The program should have methods that return the following:• the total rainfall for the year• the average monthly rainfall• the month with the most rain• the month with the least rainDemonstrate the class in a complete program.Input Validation: Do not accept negative numbers for monthly rainfall figures.arrow_forward
- You are given an array of n integers. Write a java method that splits the numbers of the array into two equal groups so that the GCD of all numbers in the first group is equal to one and the GCD of all numbers in the second group is not one. After splitting the numbers display the resulting groups as shown in the sample run below. Note: 1. The array has even size. 2. All elements of the array are less than 100. 3. You can define at most only one one-dimensional array. Sample run of the method: Input array: {6,7,9,4,3,2} Output Group one : {7,9,3} //Ignore Order of numbers. Order is not important Group two: {2,4,6} //Ignore Order of numbers. Order is not importantarrow_forwardRename the show method to people Override the show method in student and in the other class you created (this will be the polymorphic method) In main program, create an array of 3 objects of class people Instantiate each array position with a different object (people, student, other) With a loop, display all the objects in the array.arrow_forwardin computer programming: java Create a rectangle class that can compute the area and the sum of its sides. Create a specialized method that will allow the class to compare other rectangle objects. The comparison should identify the larger rectangle according to the object’s area. You should be able to implement the class where the user provides rectangle dimensions. The program should be able to display which of the rectangle inputs is larger using the Rectangle class method that you defined. Sample output: Enter Length R1: 1 Enter Width R1: 1 Enter Length R2: 2 Enter Width R2: 2 Results: R1 Area: 1 R1 Sum: 2 R2 Area: 4 R2 Sum: 4 R2 is the bigger Rectangle! Note: All characters in boldface are user inputs.arrow_forward
- Write a method that displays an n-by-n matrix usingthe following header:public static void printMatrix(int n)Each element is 0 or 1, which is generated randomly. Write a test programthat prompts the user to enter n and displays an n-by-n matrix. Here is asample run: Enter n: 3 ↵Enter0 1 00 0 01 1 1arrow_forwardPlease add comment on each line Thank you very much! Write a static method, getBigWords, that gets a single String parameter and returns an array whose elements are the words in the parameter that contain more than 5 letters. (A word is defined as a contiguous sequence of letters.) EXAMPLE: So, if the String argument passed to the method was "There are 87,000,000 people in Canada", getBigWords would return an array of two elements, "people" and "Canada". ANOTHER EXAMPLE: If the String argument passed to the method was "Send the request to support@turingscraft.com", getBigWords would return an array of three elements, "request", "support" and "turingscraft".arrow_forwardNeeds to be written in java: Write a program with a main() method that asks the user to input an integer array of 10 elements.Next, create three methods described below. From inside your main() method, call each of thethree methods described below and print out the results of the methods 2, 3, which return values.1. printReverse() - a method that receives an array of integers, then reverses the elements ofthe array and prints out all the elements from inside the method. Print all in one lineseparated by commas (see sample output below).2. getLargest() – a method that receives an array of integers, then returns the largest integervalue in the array. (print result from main())3. computeTwice()- a method that receives the previously reversed array of integers, thenreturns an array of integers which doubles the value of each number in the array (see thesample output below). (print result from main())Sample output:Enter a number:22Enter a number:34Enter a number:21Enter a number:35Enter a…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
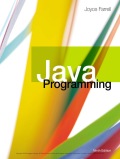