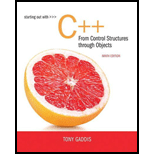
Regular “for” loop:
The “for” loop is also referred as count-controlled loop and it contains three processes as follows:
- Initialization
- Compare
- Update
It is possible to initialize more than one variable in loop expression. In some cases it is necessary to track number of times the loop gets executed; so C++ provides “for” loop.
Syntax:
The syntax for the “for” loop is as follows:
//for loop condition
for (initialization; compare; update)
{
//statement
}
In the above format,
- The “initialization” represents the initialization variable for the loop.
- The “compare” represents the comparison condition which is used to exit from the loop when the condition becomes failure.
- The “update” represents the counter variable to count each iteration in the loop.
- The “statement” represents the statements which are needs to be executed during the loop iteration.
Range based for loop:
The range based “for” loop is the one which iterates each element once from the array. On iteration, the loop copies element from the array to variable each time.
- For example, consider an array consists of six elements; so, the loop will iterate for 6 times to retrieve each element from the array.
Syntax:
The syntax for the range based “for” loop is as follows:
for(datatype rangeVariable: array)
Statement;
In the above format,
- The “datatype” is the data type followed by the range variable; the range variable uses the same data type as the array follows or else it follows a type to which the element of the array can be automatically converted.
- The “rangeVariable” is the name which receives the value from array for each loop iteration; this variable changes the value on each loop iteration like first, second and so on.
- The “array” represents the name of the array that the loop needs to operate; from this array, the loop iterates once for every element.
- The “statement” represents the statement that needs to be executed during the loop iteration.
- Start the program
- Declare the variable “name” as “int” and assign the size of the variable “name” with 20 elements.
- Then, write the regular “for” loop and range based for loop to print each element of the array.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
- (Electrical eng.) Write a program that specifies three one-dimensional arrays named current, resistance, and volts. Each array should be capable of holding 10 elements. Using a for loop, input values for the current and resistance arrays. The entries in the volts array should be the product of the corresponding values in the current and resistance arrays (sovolts[i]=current[i]resistance[i]). After all the data has been entered, display the following output, with the appropriate value under each column heading: CurrentResistance Voltsarrow_forwardCan enumerators be used in a loop to step through the elements of an array? Why or why not?arrow_forwardA for loop is often used when processing the elements of an array. Given an array called scores with 10 elements, what entries would complete the for loop header shown below? for (int i = 10; i++) O first blank: 0 second blank: <= O first blank: 0 second blank: < O first blank: 1 second blank: <= first blank: 1 second blank: < O Oarrow_forward
- Ch7 - Arrays java In a loop, ask the user to enter 10 integer values and store the values in an array. Pass the array to a method. In the method, use a loop to subtract 5 from each element and return the changed array to the main method.In the main method, use a loop to add the array values and display the result.arrow_forwardFill-in-the-Blank To print out all elements of a two-dimensional array you would normally use a(n) _________ loop.arrow_forwardC: Array & Looparrow_forward
- The arrays array1 and array2 each hold 25 integer elements. Write code that copies the values in array1 to array2.arrow_forwardTrue or FalseYou use the == operator to compare two array reference variables and determine whether the arrays are equal.arrow_forwardWrite code that includes a Sub Procedure that includes an array initialized with integers, statements that print the total number of integers in the array, the sum of all integers in the array, and a For Each loop that prints the elements of the array. Include another For Each loop that add the elements of the array to a listbox.arrow_forward
- Date Printer Write a program that reads a char array from the user containinga date in the form mm/dd/yyyy. It should print the date in the form March 12, 2014.arrow_forwardQuestion Write a statement that declares a string arrays initialized with the following strings: Write a loop that display the content of each element in the array declared in question 1 "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" and "Sunday"arrow_forwardQuestion 1 Write a program that declares an array of 100 doubles. The program asks the user to enter a number N between 100, and then: initializes the first N elements of the array to random numbers between 1 and 100. calculates the average of these numbers and displays it. A final loop displays the elements of the array, each on a line, with an indication as to whether that number is lower or higher than the average. For example, if the numbers generated are 5, 28, 3, 8, 15, 7, 22, 6, 1, 4, then the program will print: Average = 9.9 5 28 3 8 15 7 22 6 1 4 11 11 11 -- 11 11 1- —— 11 lower than average higher than average lower than average lower than average higher than average lower than average higher than average lower than average lower than average lower than averagearrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
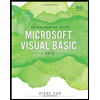
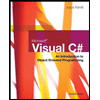
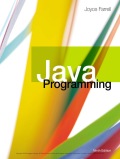
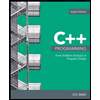
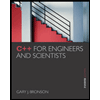