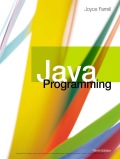
Explanation of Solution
Program:
File name: “Card.java”
//Defining Card class
class Card
{
//Declaring variables
int cardValue;
String cardSuit;
String cardRank;
//Defining setValue method
public void setValue(int value)
{
cardValue=value;
//Computing value
switch(cardValue)
{
case 1: cardRank="Ace"; break;
case 2: cardRank="Two"; break;
case 3: cardRank="Three"; break;
case 4: cardRank="Four"; break;
case 5: cardRank="Five"; break;
case 6: cardRank="Six"; break;
case 7: cardRank="Seven"; break;
case 8: cardRank="Eight"; break;
case 9: cardRank="Nine"; break;
case 10: cardRank="Ten"; break;
case 11: cardRank="Jack"; break;
case 12: cardRank="Queen"; break;
case 13: cardRank="King"; break;
default: cardRank="";
}
}
//Defining setSuit() method
public void setSuit(String str)
{
//Assigning string value to suit
cardSuit=str;
}
}
File name: “War2.java”
//Import necessary header files
import java.util...

Want to see the full answer?
Check out a sample textbook solution
- The Spider Game Introduction: In this assignment you will be implementing a game that simulates a spider hunting for food using python. The game is played on a varying size grid board. The player controls a spider. The spider, being a fast creature, moves in the pattern that emulates a knight from the game of chess. There is also an ant that slowly moves across the board, taking steps of one square in one of the eight directions. The spider's goal is to eat the ant by entering the square it currently occupies, at which point another ant begins moving across the board from a random starting location. Game Definition: The above Figure illustrates the game. The yellow box shows the location of the spider. The green box is the current location of the ant. The blue boxes are the possible moves the spider could make. The red arrow shows the direction that the ant is moving - which, in this case, is the horizontal X-direction. When the ant is eaten, a new ant is randomly placed on one of the…arrow_forwardCreate a BowlingTeam class The class has 2 fields: a field for the team name and an array that holds the team members’ names. Create get and set methods for the teamName field. Add a setMember method that sets a team member’s name. The method requires a position and a name, and it uses the position as a subscript to the members array. Add a getMember method that returns a team member’s name. The method requires a value used as a subscript that determines which member’s name to return. Create a BowlingTeamDemo class. In the main method include the following: Declare 2 variables: name and a constant NUM_TEAMS that holds 4 Bowling Team objects. Declare and instantiate an array teams of BowlingTeam objects. Using nested for loops, prompt the user to enter the 4 team names and enter the team members’ names. Using another nested for loop, output each team’s name and their team members’. Output should look like: Members of team The Lucky Strikes Carlos Diego Rose Lynn Members of team I…arrow_forwardDice Rolling Class In this problem, you will need to create a program that simulates rolling dice. To start this project, you will first need to define the properties and behaviors of a single die that can be reused multiple times in your future code. This will be done by creating a Dice class. Create a Dice class that contains the following members: Two private integer variables to store the minimum and maximum roll possible. Two constructors that initialize the data members that store the min/max possible values of rolls. a constructor with default min/max values. a constructor that takes 2 input arguments corresponding to the min and max roll values Create a roll() function that returns a random number that is uniformly distributed between the minimum and maximum possible roll values. Create a small test program that asks the user to give a minValue and maxValue for a die, construct a single object of the Dice class with the constructor that initializes the min and max…arrow_forward
- Write a statement to create an array, named productList, in a supportive class, with a size of TEN and a data type of String: Question 7 (Mandatory) Write an invoke statement for the currentCircle object from the Circle class, to the behavior setCircleRadius, that invokes validateRadius in the parameters, which passes input in the parameters: ); Question 8 (Mandatory) Write a test condition for a selection structure that tests the values of the Strings named, userName and borrowedUserName, where case matters and the results will be -1, 0 or 1: Question 9 (Mandatory). Write a test condition for a selection structure that tests the values of the Strings named, userName and borrowedUserName, where case does not matter and the results will be true or false:arrow_forwardWhy is it important for a class to have a destructor implemented? With your comment, fill in the spaces.arrow_forwardFinish the TestPlane class that contains a main method that instantiates at least two Planes. Add instructions to instantiate your favorite plane and invoke each of the methods with a variety of parameter values to test each option within each method. To be able to test the functionality of each phase, you will add instructions to the main method in each phase.arrow_forward
- Add a list of procedures in your patient class, fill them and print them with the patient data. This is called composition which is a "has a" relationship. A patient has procedures. Write a class named Patient that has attributes for the following data: First name, middle name, and last name Address, city, state, and ZIP code Phone number Name and phone number of emergency contact The Patient class’s _ _init_ _ method should accept an argument for each attribute. The Patient class should also have accessor and mutator methods for each attribute. Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data: Name of the procedure Date of the procedure Name of the practitioner who performed the procedure Charges for the procedure The Procedure class’s _ _init_ _ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator…arrow_forwardmau Open Leathing inta... - The class has data members that can hold the name of your cube, length of one of the sides and the color of your cube. - The class has a constructor that accepts the name, length of one of the sides, and color as arguments and sets the data members to those values. - The class has the following methods to set the corresponding data member. setName(string newName) setSide(double newSide) setColor(string newColor) - The class has a method called getVolume() that returns the volume of the cube, calculated by cubing the length of the side. - The class has a method called volumelncrease(double newVolume) that receive the percent the volume should increase, and then the side to the corresponding value. i - For example, 2.5 indicates 2.5% increasing of the volume. So you take the current volume, increase it by 2.5%, and then find the cube root to calculate the new side length. You can use the built in function called cbrt, part of the math library, to find the cube…arrow_forwardCreate one more method in the Starship class: encounter(self, enemy_ship) This method takes in self, along with another Starship object. The method should simulate a battle between the two ships. How the method is implemented is almost entirely up to you. You could take in user input for each crew member, or have them each act randomly from the available options, or specify exactly what a given crew member of each type should do. The only requirements are as follows: Each crew member on each ship should get at least one “turn” before the battle is complete. If the crew member is Injured on their turn, they should do nothing. You may assume that each ship will have at least one crew member uninjured when the encounter begins. You shouldn’t make any assumptions about how many crew members there will be on a given ship, where they will start, or about which subclasses will be present - it’s possible that you’ll have test cases including a ship with no captain, or no doctor.…arrow_forward
- Write a shoe class with the following attributes: color (e.g., "blue", "green", "orange") displayName (e.g., "nikes, adidas", "puma") price (e.g., 100, 200,60) Include only one constructor. It should have parameters for each of the attributes and set their values. Additionally, include getters and setters for each of the attributes. Add a driver, name it Purchases, and create 2 objects. Finally, print out some information about both objects (i.e., print the information from some or all of the getters). For example, if you created a shoe object whose color was blue, whose display name was shoes , for a price of 230, you could use the getters to print something like this:These work trousers are blue and cost $230arrow_forwardCreate a Ferret class with properties name and weight passed to the constructor.arrow_forwardCreate a player class and a Key class. A Player hasa name, HP, and a key. A character may only have one key at a time, a Key contains a String array that lists the names of all the placed the key can go. The key should have a use() function that takes in a string of what it is trying to be used on, the function will return true if it can be used on that door, and false if it cannot. The player can pick Up and Drop a key.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
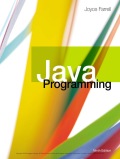