Create one more method in the Starship class: encounter(self, enemy_ship) This method takes in self, along with another Starship object. The method should simulate a battle between the two ships. How the method is implemented is almost entirely up to you. You could take in user input for each crew member, or have them each act randomly from the available options, or specify exactly what a given crew member of each type should do. The only requirements are as follows: Each crew member on each ship should get at least one “turn” before the battle is complete. If the crew member is Injured on their turn, they should do nothing. You may assume that each ship will have at least one crew member uninjured when the encounter begins. You shouldn’t make any assumptions about how many crew members there will be on a given ship, where they will start, or about which subclasses will be present - it’s possible that you’ll have test cases including a ship with no captain, or no doctor. It’s also possible you’ll have two captains on the same ship. The method does not need to return anything, but it can if you want to indicate a result of some sort. Be sure to specify what, if anything, it returns as part of your documentation for this method.
Create one more method in the Starship class: encounter(self, enemy_ship) This method takes in self, along with another Starship object. The method should simulate a battle between the two ships. How the method is implemented is almost entirely up to you. You could take in user input for each crew member, or have them each act randomly from the available options, or specify exactly what a given crew member of each type should do. The only requirements are as follows: Each crew member on each ship should get at least one “turn” before the battle is complete. If the crew member is Injured on their turn, they should do nothing. You may assume that each ship will have at least one crew member uninjured when the encounter begins. You shouldn’t make any assumptions about how many crew members there will be on a given ship, where they will start, or about which subclasses will be present - it’s possible that you’ll have test cases including a ship with no captain, or no doctor. It’s also possible you’ll have two captains on the same ship. The method does not need to return anything, but it can if you want to indicate a result of some sort. Be sure to specify what, if anything, it returns as part of your documentation for this method.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Create one more method in the Starship class:
- encounter(self, enemy_ship)
- This method takes in self, along with another Starship object.
- The method should simulate a battle between the two ships.
How the method is implemented is almost entirely up to you. You could take in user input for each crew member, or have them each act randomly from the available options, or specify exactly what a given crew member of each type should do. The only requirements are as follows:
- Each crew member on each ship should get at least one “turn” before the battle is complete.
- If the crew member is Injured on their turn, they should do nothing.
- You may assume that each ship will have at least one crew member uninjured when the encounter begins.
- You shouldn’t make any assumptions about how many crew members there will be on a given ship, where they will start, or about which subclasses will be present - it’s possible that you’ll have test cases including a ship with no captain, or no doctor. It’s also possible you’ll have two captains on the same ship.
- The method does not need to return anything, but it can if you want to indicate a result of some sort. Be sure to specify what, if anything, it returns as part of your documentation for this method.
If you want to be lazy, hardcode the method to have every crew member in both ships take one turn, and use it to move to the Sleep Pods, ignoring the enemy ship completely.
Current Code:
![Å
File Edit Selection View Go Run Terminal Help
hw12.py ×
C: > Users > lane3 > OneDrive > Desktop > hw12.py > ...
1 class Crew:
HN M tin
2
3
ⒸOA O
10°F
Clear
4
5
6
789
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
def _init__(self, name):
self.name = name
self.status = 'Active'
self.location = 'Sleep Pods'
def _repr__(self):
return f'{self.name} : {self.status}, at {self.location}'
def move(self, location):
place
if location in place:
=
else:
self.location = location
["Bridge", "Medbay", "Engine", "Lasers", "Sleep Pods"]
print("Not a valid location.")
def repair(self, ship):
print (f"<{self.name}› doesn't know how to do that.")
def first_aid(self, ship):
print(f"<{self.name}› doesn't know how to do that.")
def fire_lasers(self, ship, target_ship, target_location):
print(f"<{self.name}› doesn't know how to do that.")
class Starship:
def __init__(self,name, crew_list):
self.name = name
self.crew_list
self.damaged
=
PROBLEMS OUTPUT DEBUG CONSOLE TERMINAL JUPYTER
= crew_list
{'Bridge':False, 'Medbay': False, 'Engine': False, 'Lasers':False, 'Sleep Pods': False}
No problems have been detected in the workspace.
hw12.py - Visual Studio Code
O Search
Filter (e.g. text, **/*.ts, !**/node_modules/**)
800-0
Ln 27, Col 1 Spaces: 4 UTF-8 CRLFPython
|
wat kather than th
Y a
X
ce duct tan tan ta de
Pure Comercial, they happy thing happy to
© Loksatt man, crm_linkļu
wa, "Padar india, Papier intia, "Leavinia, "Moup Pudr inating.
3.10.6 64-bit
:
^х
8:17 PM
12/7/2022](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F44cf4428-d50c-4dc1-a095-73926c558c67%2F5cac49be-6c03-4661-9f52-f369cdf41fd1%2Fuki0if_processed.png&w=3840&q=75)
Transcribed Image Text:Å
File Edit Selection View Go Run Terminal Help
hw12.py ×
C: > Users > lane3 > OneDrive > Desktop > hw12.py > ...
1 class Crew:
HN M tin
2
3
ⒸOA O
10°F
Clear
4
5
6
789
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
def _init__(self, name):
self.name = name
self.status = 'Active'
self.location = 'Sleep Pods'
def _repr__(self):
return f'{self.name} : {self.status}, at {self.location}'
def move(self, location):
place
if location in place:
=
else:
self.location = location
["Bridge", "Medbay", "Engine", "Lasers", "Sleep Pods"]
print("Not a valid location.")
def repair(self, ship):
print (f"<{self.name}› doesn't know how to do that.")
def first_aid(self, ship):
print(f"<{self.name}› doesn't know how to do that.")
def fire_lasers(self, ship, target_ship, target_location):
print(f"<{self.name}› doesn't know how to do that.")
class Starship:
def __init__(self,name, crew_list):
self.name = name
self.crew_list
self.damaged
=
PROBLEMS OUTPUT DEBUG CONSOLE TERMINAL JUPYTER
= crew_list
{'Bridge':False, 'Medbay': False, 'Engine': False, 'Lasers':False, 'Sleep Pods': False}
No problems have been detected in the workspace.
hw12.py - Visual Studio Code
O Search
Filter (e.g. text, **/*.ts, !**/node_modules/**)
800-0
Ln 27, Col 1 Spaces: 4 UTF-8 CRLFPython
|
wat kather than th
Y a
X
ce duct tan tan ta de
Pure Comercial, they happy thing happy to
© Loksatt man, crm_linkļu
wa, "Padar india, Papier intia, "Leavinia, "Moup Pudr inating.
3.10.6 64-bit
:
^х
8:17 PM
12/7/2022
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
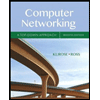
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
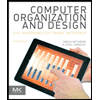
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
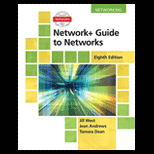
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
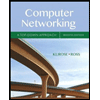
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
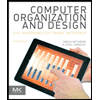
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
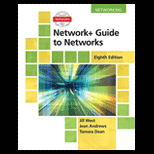
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
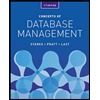
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
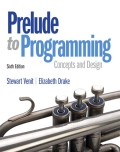
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
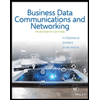
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY