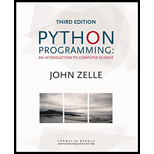
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Question
Chapter 6, Problem 7PE
Program Plan Intro
Calculate the nth Fibonacci number
- Import the required packages
- The function named “fibonacci()” is defined and inside the “fibonacci()”,
- Declare the required variables named as “x”, “y” and “z”.
- Initialize a for loop to calculate and assign the value of the variables.
- Return the value stored in the “y” variable.
- In the “main()” function,
- Generate a prompt to take the user input.
- Call the method named “fibonacci()” with user input as the argument.
- Print the output statement and output value.
- Call the function “main()”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Of the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization?
Part A - Define each primary component of the information system.
Part B - Include your perspective on why your selection is most important.
Part C - Provide an example from your personal experience to support your answer.
Management Information Systems
Q2/find the transfer function C/R for the system shown in the figure
Re
ད
Chapter 6 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 6 - Prob. 1TFCh. 6 - Prob. 2TFCh. 6 - Prob. 3TFCh. 6 - Prob. 4TFCh. 6 - Prob. 5TFCh. 6 - Prob. 6TFCh. 6 - Prob. 7TFCh. 6 - Prob. 8TFCh. 6 - Prob. 9TFCh. 6 - Prob. 10TF
Ch. 6 - Prob. 1MCCh. 6 - Prob. 2MCCh. 6 - Prob. 3MCCh. 6 - Prob. 4MCCh. 6 - Prob. 5MCCh. 6 - Prob. 6MCCh. 6 - Prob. 7MCCh. 6 - Prob. 8MCCh. 6 - Prob. 9MCCh. 6 - Prob. 10MCCh. 6 - Prob. 1DCh. 6 - Prob. 2DCh. 6 - Prob. 3DCh. 6 - Prob. 4DCh. 6 - Prob. 5DCh. 6 - Prob. 1PECh. 6 - Prob. 2PECh. 6 - Prob. 3PECh. 6 - Prob. 4PECh. 6 - Prob. 5PECh. 6 - Prob. 6PECh. 6 - Prob. 7PECh. 6 - Prob. 8PECh. 6 - Prob. 9PECh. 6 - Prob. 10PECh. 6 - Prob. 11PECh. 6 - Prob. 12PECh. 6 - Prob. 13PECh. 6 - Prob. 14PECh. 6 - Prob. 16PECh. 6 - Prob. 17PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forwardPlease original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forward
- What are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forwardDiscuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forwardDetermine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward
- • Solve the problem (pls refer to the inserted image)arrow_forwardWrite .php file that saves car booking and displays feedback. There are 2 buttons, which are <Book it> <Select a date>. <Select a date> button gets an input from the user, start date and an end date. Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, it books cars for specific dates, with bookings saved. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. In the booking.json file, if the Car ID and start date and end date matches, it fails Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert).arrow_forwardWrite .php file with the html that saves car booking and displays feedback. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. There are 2 buttons, which are <Book it> <Select a date> Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, book cars for specific dates, with bookings saved. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert). And then add an additional feature that only free dates are selectable (e.g., calendar view).arrow_forward
- • Solve the problem (pls refer to the inserted image) and create line graph.arrow_forwardwho started the world wide webarrow_forwardQuestion No 1: (Topic: Systems for collaboration and social business The information systems function in business) How does Porter's competitive forces model help companies develop competitive strategies using information systems? • List and describe four competitive strategies enabled by information systems that firms can pursue. • Describe how information systems can support each of these competitive strategies and give examples.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
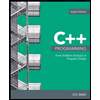
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
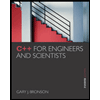
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
What Are Data Types?; Author: Jabrils;https://www.youtube.com/watch?v=A37-3lflh8I;License: Standard YouTube License, CC-BY
Data Types; Author: CS50;https://www.youtube.com/watch?v=Fc9htmvVZ9U;License: Standard Youtube License