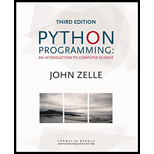
Draw a face
Program plan:
- Import the header file.
- Define the “drawFace” method
- Get the “x” and “y” positions
- Get the “p1” and “p2” positions
- Call the “Oval” method for drawing the face
- Set the color to the face
- Draw the face
- Get the “lc” and “rc” position
- Call the “Circle” method
- Set the color to the face
- Call the “draw” method
- Call the “Circle” method
- Set the color to the face
- Call the “draw” method
- Get the “m1”, “m2”, and “m3” values
- Call the “Rectangle” method
- Set the color to the face
- Call the “draw” method
- Call the “Line” method
- Get the center position
- Get the “x” and “y” position
- Call the “Point” method
- Call the “Line” method
- Call the “draw” method
- Get the “x” and “y” position for different variables
- Calculate “t” and “t1” value
- Call the “Rectangle” method
- Call the “draw” method
- Iterate “i” until it reaches 7
- Clone the teeth by calling “clone” method
- Move the face by calling “move” method
- Call the “draw” method
- Define the main method.
- Read the filename from the user
- Get the image width and height from the file
- Set the graph win size
- Set the coords for graph
- Draw the face
- Get the number of faces from the user
- Iterate “i” until it reaches “n” value
- Get the mouse action
- Call the “drawFace” method
- Call the function “main()”.

This Python program is used to get the GIF (Graphic Interchange format) file and draw the smileys when the user clicking the GIF image.
Explanation of Solution
Program:
#import the header file
from graphics import *
#definition of "drawFace" method
def drawFace(center, size, window):
#get the "x" value
x1 = center.getX()
#get the "y" value
y1 = center.getY()
#get the "p1" value
p1 = Point(x1-(.7 * size), y1 - size)
#get the "p2" value
p2 = Point(x1+(.7 * size), y1 + size)
#call the "Oval" method
head = Oval(p1, p2)
#fill the color
head.setFill("white")
#call the "draw" method
head.draw(window)
#get the "lc" value
lc = Point(x1 - .2 * size, y1 + .6 * size)
#get the "rc" value
rc = Point(x1 + .2 * size, y1 + .6 * size)
#call the "Circle" method
leftEye = Circle(lc, .13 * size)
#fill the color
leftEye.setFill("white")
#call the "draw" method
leftEye.draw(window)
#call the "Circle" method
rightEye = Circle(rc, .13 * size)
#fill the color
rightEye.setFill("white")
#call the "draw" method
rightEye.draw(window)
#get the "m1" value
m1 = Point(x1 - .3 * size, y1 - .5 * size)
#get the "m2" value
m2 = Point(x1 + .3 * size, y1 - .25 * size)
#get the "m3" value
m3 = Point(x1 + .3 * size, y1 - .5 * size)
#call the "Rectangle" method
mouth = Rectangle(m1, m2)
#fill the color
mouth.setFill("white")
#call the "draw" method
mouth.draw(window)
#call the "Line" method
leftLip = Line(m1, Point(x1- .3 * size, y1 - .25 * size))
#get the center position
mLeftCent = leftLip.getCenter()
#get the "x" position
mLCx = mLeftCent.getX()
#get the "y" position
mLCy = mLeftCent.getY()
#call the "Point" method
mRightCent = Point(x1 + .3 * size, mLCy)
#call the "Line" method
lip = Line(mLeftCent, mRightCent)
#call the "draw" method
lip.draw(window)
#get the "x" positions
m1x = m1.getX()
m3x = m3.getX()
#get the "y" positions
m1y = m1.getY()
m2y = m2.getY()
#calculate the "t" value
t = m1x - m3x
#calculate the "t1" value
t1 = Point(m1x - (1/8 * t), m2y)
#call the "Rectangle" method
teeth = Rectangle(m1, t1)
#call the "draw" method
teeth.draw(window)
#iterate "i" until it reaches 7
for i in range (7):
#clone the face
t2 = teeth.clone()
#call the "move" method
t2.move(-i * (1/8 * t), 0)
#call the "draw" method
t2.draw(window)
#definition of main method
def main():
#get the input filename from the user
fname = input("Enter filename: ")
infile = Image(Point(10, 10), fname)
#get the width of the image
wWidth = infile.getWidth()
#get the height of the image
wHeight = infile.getHeight()
#set graph win name
window = GraphWin('Smile!', wWidth,wHeight)
#set the coords
window.setCoords(0, 0, 20, 20)
#draw a smiley
infile.draw(window)
#get how many faces the user wants to draw
n = eval(input("How many faces should we block? "))
#iterate "i" until it reaches "n"
for i in range(n):
#get the mouse action
point = window.getMouse()
#draw a face by calling the method "drawFace"
drawFace(point, 3, window)
#call the "main" method
main()
Output:
Enter filename: test_img.gif
How many faces should we block? 2
>>>
Screenshot of “Smile!” window
Want to see more full solutions like this?
Chapter 6 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- In this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. The source code file already contains the necessary assignment and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Make sure the file MovieGuide.pyis selected and open. Write thewhile loop using a sentinel value to control the loop, and also write the statements that make up the body of the loop. Execute the program by clicking the Run button at the bottom of the screen. Input the following as star ratings: 0, 3, 4, 4, 1, 1, 2, -1 Ensure that the average output is correct.arrow_forwardIn this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. The source code file already contains the necessary assignment and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie.arrow_forwardPythonarrow_forward
- subject: Assembly Language Write a program that displays a string in all possible combinations of foreground and background colors (16 x 16 =256). The colors are numbered from 0 to 15, so you can use a nested loop to generate all possible combinations.arrow_forwardUsing a Counter-Controlled whileLoop Summary In this lab, you use a counter-controlled while loop in a Java program provided for you. When completed, the program should print the numbers 0 through 10, along with their values multiplied by 2 and by 10. The data file contains the necessary variable declarations and some output statements. Instructions Ensure the file named Multiply.java is open. Write a counter-controlled while loop that uses the loop control variable to take on the values 0 through 10. Remember to initialize the loop control variable before the program enters the loop. In the body of the loop, multiply the value of the loop control variable by 2 and by 10. Remember to change the value of the loop control variable in the body of the loop. Execute the program by clicking Run. Record the output of this program.arrow_forwardProgramming language C#arrow_forward
- Design and implement an application that prints the first few verses of the traveling song “One Hundred Bottles of Beer.” Use a loop such that each iteration prints one verse. Read the number of verses to print from the user. Validate the input. The following are the first two verses of the song:100 bottles of beer on the wall100 bottles of beerIf one of those bottles should happen to fall99 bottles of beer on the wall99 bottles of beer on the wall99 bottles of beerIf one of those bottles should happen to fall98 bottles of beer on the wallarrow_forwardTranscribed Image Text Write a loop of your choice that continues to read an integer x as long as x not divisible by 5 and 3. Complete the missing code in the space provided below. { int x=1; .....arrow_forwardpython Write a program that uses a text file to store the days and hours that a user worked in a week. The program should begin by prompting for the number of days worked in the week. It should continue with a loop for input of the days and hours and for writing these to the file, each on its own line. Sample Output (inputs shown in boldface)How many days did you work this week? 5Enter day of week MondayHow many hours did you work on Monday? 10Enter day of week TuesdayHow many hours did you work on Tuesday? 8Enter day of week WednesdayHow many hours did you work on Wednesday? 12Enter day of week FridayHow many hours did you work on Friday? 12Enter day of week SaturdayHow many hours did you work on Saturday? 8File was createdarrow_forward
- Chrome Capture 767x397 Create a python program called code.py. Write a function named pi_multiples() that takes an integer parameter num. This function repeatedly asks the user to enter an integer between 2 and 50. Assume the user will always enter an integer, but if the number is outside this range, the loop must end. So, for any integer x entered within the range, your function must do the following; if x is divisible by num (the parameter), it must multiply x by the value of pi (call it result) and maintain a sum of these results. In every iteration of the loop your function must print x and the result. And when the loop terminates your function should return the sum. To test your function, call pi_multiples() function using any integer number of your choice (for num) and print the returned value. Hint: to use the pi value do the following import math and use math.pi for the value of pi. frames: 0 0/ 10 secs Zip your file, name it code.zip, and submit it using the link available in…arrow_forwardWrite a complete program to generate a username based on a user's first and last name. Part One: Get a Valid First and Last Name ask the user for and read in their first and last name use a loop to validate the first name: it must be at least one character long and it must start with a letter use a loop to validate the last name: it must not contain any spaces Hint: use a nested loop. The outer loop will iterate until there is valid input. The inner loop will check each character in the String to see if any of them are whitespace. Part Two: Generate a Username generate a two-digit random number (i.e., a random number between 10 and 99, inclusive) create a username that is the following data concatenated: the first letter of their first name in lower case the first five characters of their last name in lower case; if the name is shorter than five characters, use the whole last name the two-digit number output the username to the user Additional Coding Requirements code…arrow_forwardAnswer fast else downvotearrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
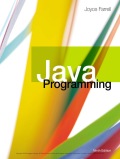
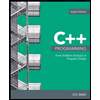