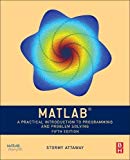
To write:
A script that will prompt the user to enter the coordinates of three points that determine a triangle, then calculate and print the area of the triangle and then call one

Answer to Problem 21E
Solution:
The function file is,
% MATLAB code to calculate the side length of the triangle.
%function file.
function [out] = sidetriangle(x1, y1, x2, y2)
%define the side of the triangle by using the function sidetriangle.
out = sqrt((x1-x2)^2+(y1-y2)^2);
end
% end of function
%The script file should be placed in the same folder.
The function file to calculate the area is,
% MATLAB code to calculate the area of the triangle and print the result.
%function file.
function areatriangle(x1, y1, x2, y2, x3, y3)
%define the area of triangle by using the function areatriangle.
a = sidetriangle(x1, y1, x2, y2);
%define the variable a.
b = sidetriangle(x2, y2, x3, y3);
%define the variable b.
c = sidetriangle(x3, y3, x1, y1);
%define the variable c.
s = (a+b+c)/2;
%define the variable s.
area = sqrt (s*(s-a)*(s-b)*(s-c));
fprintf('Area of the triangle is %3.2f\n', area);
%print the area of the triangle.
end
% end of function
%The script file should be placed in the same folder.
The script file is,
% MATLAB code to calculate the area of the triangle by calling a function.
%script file.
point1 = input('the coordinates of the fist point is entered:');
%enter the coordinates of the first point.
point2 = input('the coordinates of the second point is entered:');
%enter the coordinates of the second point.
point3 = input('the coordinates of the third point is entered:');
%enter the coordinates of the third point.
x1 = point1(1);y1 = point1(2);
%define the variable x1.
x2 = point2(1);y2 = point2(2);
%define the variable x2.
x3 = point3(1);y3 = point3(2);
%define the variable x3.
areatriangle(x1, y1, x2, y2, x3, y3)
% end of file
%The script file should be placed in the same folder.
Explanation of Solution
The given two points are
The formula for the distance between the two points is given as,
Substitute 0 for
Consider the three points are
The side of the triangle is,
Substitute 0 for
The side of the triangle is,
Substitute 5 for
The side of the triangle is,
Substitute 5 for
The formula for half sum of the sides of the triangle is,
Substitute 5 for a, 5 for b and
The formula for the area of the triangle is,
Substitute 5 for a, 5 for b,
MATLAB Code:
clc
clear all
close all
% MATLAB code to calculate the side length of the triangle.
%function file.
function [out] = sidetriangle(x1, y1, x2, y2)
%define the side of the triangle by using the function sidetriangle.
out = sqrt((x1-x2)^2+(y1-y2)^2);
end
% end of function
%The script file should be placed in the same folder.
% MATLAB code to calculate the area of the triangle and print the result.
%function file.
function areatriangle(x1, y1, x2, y2, x3, y3)
%define the area of triangle by using the function areatriangle.
a = sidetriangle(x1, y1, x2, y2);
%define the variable a.
b = sidetriangle(x2, y2, x3, y3);
%define the variable b.
c = sidetriangle(x3, y3, x1, y1);
%define the variable c.
s = (a+b+c)/2;
%define the variable s.
area = sqrt (s*(s-a)*(s-b)*(s-c));
fprintf('Area of the triangle is %3.2f\n', area);
%print the area of the triangle.
end
% end of function
%The script file should be placed in the same folder.
% MATLAB code to calculate the area of the triangle by calling a function.
%script file.
point1 = input('the coordinates of the fist point is entered:');
%enter the coordinates of the first point.
point2 = input('the coordinates of the second point is entered:');
%enter the coordinates of the second point.
point3 = input('the coordinates of the third point is entered:');
%enter the coordinates of the third point.
x1 = point1(1);y1 = point1(2);
%define the variable x1.
x2 = point2(1);y2 = point2(2);
%define the variable x2.
x3 = point3(1);y3 = point3(2);
%define the variable x3.
areatriangle(x1, y1, x2, y2, x3, y3)
% end of file
%The script file should be placed in the same folder.
Save the MATLAB scripts with names, sidetriangle.m, areatriangle.m and areacall.m in the current folder. Execute the script by typing the script name at the command window to generate result.
Result:
The results are,
Therefore, the results and script files are stated above.
Want to see more full solutions like this?
Chapter 6 Solutions
MATLAB: A Practical Introduction to Programming and Problem Solving
- Algebra and Trigonometry (MindTap Course List)AlgebraISBN:9781305071742Author:James Stewart, Lothar Redlin, Saleem WatsonPublisher:Cengage LearningCollege AlgebraAlgebraISBN:9781305115545Author:James Stewart, Lothar Redlin, Saleem WatsonPublisher:Cengage LearningAlgebra & Trigonometry with Analytic GeometryAlgebraISBN:9781133382119Author:SwokowskiPublisher:Cengage
- Trigonometry (MindTap Course List)TrigonometryISBN:9781305652224Author:Charles P. McKeague, Mark D. TurnerPublisher:Cengage LearningMathematics For Machine TechnologyAdvanced MathISBN:9781337798310Author:Peterson, John.Publisher:Cengage Learning,
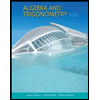
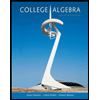
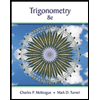
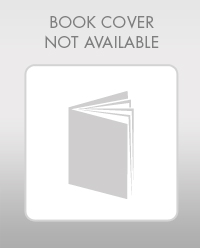