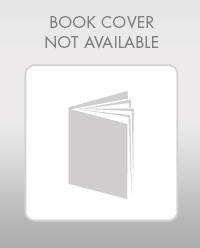
Concept explainers
A)
Explanation of Solution
Analysis of given code:
The given code is used to print “Hello world” once. The process is carried out using a “do…while” loop. Initially the “count” variable is assigned with a value “3”. The loop executes till the value of “count” is lesser than “1”.
Given code segment:
//Include necessary header file
#include <iostream>
using namespace std;
//Function main
int main()
{
// Declare and initialize a variable
int count = 3; //Line 1
//Loop
do //Line 2
{ //Line 3
// Print statement
cout<<"Hello World...
B)
Explanation of Solution
Analysis of given code:
The given code is used to print value of the variable “val”. The process is carried out using a “do…while” loop. Initially the “val” variable is assigned with a value “5”. The loop executes till the value of “val” is greater than or equal to “5”.
Given code segment:
// Include necessary header file
#include <iostream>
using namespace std;
// Driver function main()
int main()
{
// Declare and initialize variable
int val = 5; //Line 1
//Loop
do //Line 2
&...
C)
Explanation of Solution
Analysis of given code:
The given code is used to print the value of variables “number” and “count” on the screen. The “do…while” loop is used to increment the value of variable “number” by “2” add the value of variable “count” by “1”.
Given code segment:
// Include necessary header file
#include <iostream>
using namespace std;
// Function main
int main()
{
// Declare and initialize variables
int count = 0, number = 0, limit = 4; //Line 1
//Loop
do //Line 2
{ //Line 3
// Increment number by 2
number+=2; //Line 4
// Increament count by 1
count++; //Line 5
} //Line 6
//Condition
while(count < limit); //Line 7
//Display number and count
cout << number << " " << count <<endl; //Line 8
//Return value
return 0;
}
Explanation:
- In “Line 1”, declare the variables “count”, “number”, and “limit” and initialize it to “0”, “0”, and “4” respectively...

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Starting Out With C++: Early Objects (10th Edition)
- #include<stdio.h>#include<stdlib.h> int cent50=0;int cent20=0;int cent10=0;int cent05=0; void calculatechange(int* change){if(*change>0){if(*change>=50){*change-=50;cent50++;}else if(*change>=20){*change-=20;cent20++;}else if(*change>=10){*change-=10;cent10++;}else if(*change>=05){*change-=05;cent05++;}calculatechange(change);}}void printchange(){if(cent50)printf("\n50cents:%d coins",cent50);if(cent20)printf("\n20cents:%d coins",cent20);if(cent10)printf("\n10cents:%d coins",cent10);if(cent05)printf("\n05cents:%d coins",cent05);cent50=0;cent20=0;cent10=0;cent05=0;}void takechange(int* change){scanf("%d",change);getchar();}int main(){int change=0;int firstinput=0;while(1){if(!firstinput){printf("\nEnter the amount:");firstinput++;}else{printf("\n\nEnter the amount to continue or Enter -1 to…arrow_forward#include<stdio.h>#include<stdlib.h> int cent50=0;int cent20=0;int cent10=0;int cent05=0; void calculatechange(int* change){if(*change>0){if(*change>=50){*change-=50;cent50++;}else if(*change>=20){*change-=20;cent20++;}else if(*change>=10){*change-=10;cent10++;}else if(*change>=05){*change-=05;cent05++;}calculatechange(change);}}void printchange(){if(cent50)printf("\n50cents:%d coins",cent50);if(cent20)printf("\n20cents:%d coins",cent20);if(cent10)printf("\n10cents:%d coins",cent10);if(cent05)printf("\n05cents:%d coins",cent05);cent50=0;cent20=0;cent10=0;cent05=0;}void takechange(int* change){scanf("%d",change);getchar();}int main(){int change=0;int firstinput=0;while(1){if(!firstinput){printf("\nEnter the amount:");firstinput++;}else{printf("\n\nEnter the amount to continue or Enter -1 to…arrow_forward17. Math Tutor Write a program that can be used as a math tutor for a young student. The program should display two random numbers to be added, such as 247 +129 The program should then pause while the student works on the problem. When the student is ready to check the answer, he or she can press a key and the program will display the correct solution: 247 +129 376arrow_forward
- #include <stdio.h>#include <stdlib.h> int cent50 = 0;int cent20 = 0;int cent10 = 0;int cent05 = 0; //Function definitionvoid calculateChange(int change) {if(change > 0) {if(change >= 50) {change -= 50;cent50++;} else if(change >= 20) {change -= 20;cent20++;} else if(change >= 10) {change -= 10;cent10++;} else if(change >= 05) {change -= 05;cent05++;}calculateChange(change);}} //Define the functionvoid printChange() { if(cent50)printf("\n50 Cents : %d coins", cent50); if(cent20)printf("\n20 Cents : %d coins", cent20); if(cent10)printf("\n10 Cents : %d coins", cent10); if(cent05)printf("\n05 Cents : %d coins", cent05);cent50 = 0;cent20 = 0;cent10 = 0;cent05 = 0; } //Function's definitionint TakeChange() { int change;printf("\nEnter the amount : ");scanf("%d", &change);return change; }//main functionint main() {//call the functionint change = TakeChange(); //use while-loop to repeatedly ask for input to the userwhile(change != -1){if((change %…arrow_forwardCoin Toss Write a function named coinToss that simulates the tossing of a coin. When you call the function, it should generate a random number in the range of 1 through 2. If the random number is 1, the function should display "heads". If the random number is 2, the function should display "tails". Demonstrate the function in a program that sks the user how many times the coin should be tossed and the coin then simulates the tossing of the coin that number of times.arrow_forwardint x1 = 66; int y1 = 39; int d; _asm { } mov EAX, X1; mov EBX, y1; push EAX; push EBX; pop ECX mov d, ECX; What is d in decimal format?arrow_forward
- #include <stdio.h>#include <stdlib.h> int cent50 = 0;int cent20 = 0;int cent10 = 0;int cent05 = 0; //Function definitionvoid calculateChange(int change) {if(change > 0) {if(change >= 50) {change -= 50;cent50++;} else if(change >= 20) {change -= 20;cent20++;} else if(change >= 10) {change -= 10;cent10++;} else if(change >= 05) {change -= 05;cent05++;}calculateChange(change);}} //Define the functionvoid printChange() { if(cent50)printf("\n50 Cents : %d coins", cent50); if(cent20)printf("\n20 Cents : %d coins", cent20); if(cent10)printf("\n10 Cents : %d coins", cent10); if(cent05)printf("\n05 Cents : %d coins", cent05); cent50 = 0;cent20 = 0;cent10 = 0;cent05 = 0; } //Function's definitionint TakeChange() { int change;printf("\nEnter the amount : ");scanf("%d", &change);return change; }//main functionint main() {//call the functionint change = TakeChange(); //use while-loop to repeatedly ask for input to the userwhile(change != -1){if((change %…arrow_forwardQuestion 7 //Determines if a number is PRIME or NOT. #include using namespace std; int prime(int n, int i-1); int main() { int num; cout > if (prime(num)== cout << " << endl; //Answer must either PRIME / NOT PRIME. else cout << " "<< endl; //Answer must either PRIME / NOT PRIME. system("pause"); int prime(int n, int { if (i n) return( ): else if (n % i == return( + prime(n, ): else return(0 + prime(n, i + 1));arrow_forward#include <stdio.h>#include <stdlib.h> int cent50 = 0;int cent20 = 0;int cent10 = 0;int cent05 = 0; //Function definitionvoid calculateChange(int change) {if(change > 0) {if(change >= 50) {change -= 50;cent50++;} else if(change >= 20) {change -= 20;cent20++;} else if(change >= 10) {change -= 10;cent10++;} else if(change >= 05) {change -= 05;cent05++;}calculateChange(change);}} //Define the functionvoid printChange() { if(cent50)printf("\n50 Cents : %d coins", cent50); if(cent20)printf("\n20 Cents : %d coins", cent20); if(cent10)printf("\n10 Cents : %d coins", cent10); if(cent05)printf("\n05 Cents : %d coins", cent05);cent50 = 0;cent20 = 0;cent10 = 0;cent05 = 0; } //Function's definitionint TakeChange() { int change;printf("\nEnter the amount : ");scanf("%d", &change);return change; }//main functionint main() {//call the functionint change = TakeChange(); //use while-loop to repeatedly ask for input to the userwhile(change != -1){if((change %…arrow_forward
- int x; X=-2; do { x++; cout0); cout<<"done"; O done O -2 done O -1 donearrow_forward5- int fun() int a, b, c, result; cout>a>>b>>c; cout<<"The result is "; reutrn result; } The error is Your answerarrow_forwardDetermine all the output from the following program as it would appear on the screen. void func1(int); void func2(int = 4, int = 5, int = 2); int func3(int &, int, int); %3D int main() { int x = 0, z = 0, y = 2; func1(y); cout << y << endl; func2(x, y, z); func2(); func3(x, y, z); = Z func1(x); cout << x << " " << y << " " << z << endl; return 0; } void func1(int b) { static int a;arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
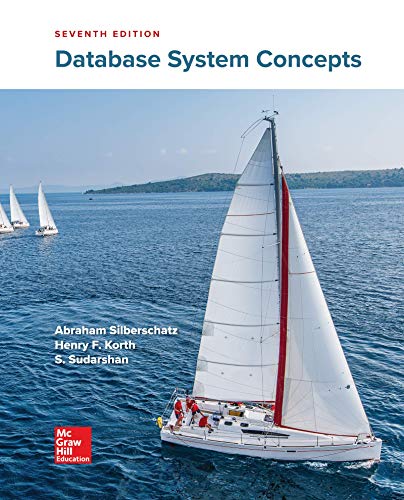
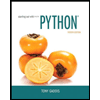
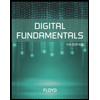
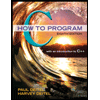
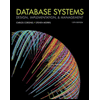
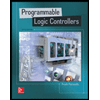