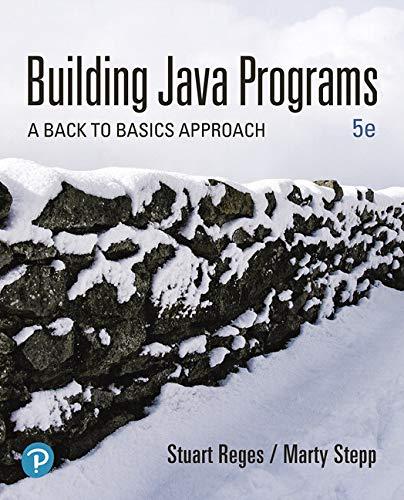
Concept explainers
Explanation of Solution
a.
Number of times that the loop will execute the body:
//definition of "Test" class
public class Test
{
//definition of main method
public static void main(String[] args)
{
//declare the "x" as integer datatype
int x = 1;
//check the "x" value is less than 100
do
{
//display the "x" value
System.out.print(x + " " ) ;
//increment the "x" value by 10
x = x + 10 ;
}whil...
Explanation of Solution
b.
Number of times that the loop will execute the body:
//definition of "Test" class
public class Test
{
//definition of main method
public static void main(String[] args)
{
//declare the "max" as integer datatype
int max = 10;
//check the "max" value is less than 10
do
{
//display the "max" value
System.out.println("count down: " + max );
//decrement the "max" value
max--;
�...
Explanation of Solution
c.
Number of times that the loop will execute the body:
//definition of "Test" class
public class Test
{
//definition of main method
public static void main(String[] args)
{
//declare the "x" as integer datatype
int x = 250;
//check the "x" mod 3 is not equal to 0
do
{
//display the "x" value
System.out...
Explanation of Solution
d.
Number of times that the loop will execute the body:
//definition of "Test" class
public class Test
{
//definition of main method
public static void main(String[] args)
{
//declare the "x" as integer datatype
int x = 100;
//check the "x" mod 2 is equal to 0
do
{
//display the "x" value
System.out...
Explanation of Solution
e.
Number of times that the loop will execute the body:
//definition of "Test" class
public class Test
{
//definition of main method
public static void main(String[] args)
{
//declare the "x" as integer datatype
int x = 2;
//check the "x" less than 200
do
{
//display the "x" value
System.out.println(x + " ") ;
//calculate the "x" value
x *= x;
;&#...
Explanation of Solution
f.
Number of times that the loop will execute the body:
//definition of "Test" class
public class Test
{
//definition of main method
public static void main(String[] args)
{
//declare the "word" as string datatype
String word = "a";
//check the length of "word" less than 10
do
{
//append the letters
word = "b" + word + "b";
}while (word.length() < 10);
//display the "word"
System.out.println(word);
}
}
Explanation:
- In the above the program “Test” is the class name and inside the main method,
- Declare the “word” variable and assign the value as “a”.
- The “do-while” loop is used to check whether length of the “word” less than 10 or not.
- In first iteration, append the “b” in front and back of “word” value and store the “word” value as “bab”...
Explanation of Solution
g.
Number of times that the loop will execute the body:
//definition of "Test" class
public class Test
{
//definition of main method
public static void main(String[] args)
{
//declare the "x" as integer datatype
int x = 100;
//check the "x" is less than 0
do
{
//display the "x" value
System.out.println(x / 10) ;
//calculate the "x" value
x = x / 2;
}while (x > 0);
}
}
Explanation:
- In the above the program “Test” is the class name and inside the main method,
- Declare the “x” variable and assign the value as 100.
- The “do-while” loop is used to check whether “x” is less than “0” or not.
- In first iteration, display the “100/10” value as 10, then calculate “100 / 2 = 50” and store it in “x” variable...
Explanation of Solution
h.
Number of times that the loop will execute the body:
//definition of "Test" class
public class Test
{
//definition of main method
public static void main(String[] args)
{
//declare the "str" as integer datatype
String str = "/\\";
//check the length of "str" is greater than 10
do
{
//calculate the "str" value
str += str;
}while (str.length() < 10);
//display the "str" value
System.out.println(str) ;
}
}
Explanation:
- In the above the program “Test” is the class name and inside the main method,
- Declare the “str” variable and assign the value as “/\\”...

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Building Java Programs: A Back To Basics Approach (5th Edition)
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
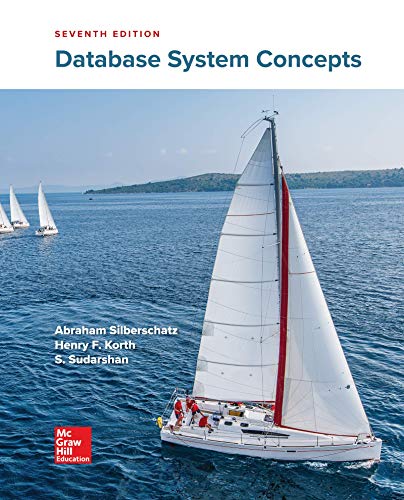
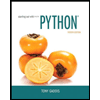
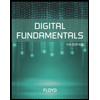
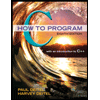
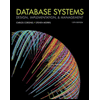
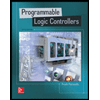