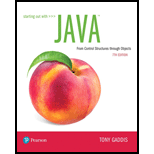
What message will the following
import javax.swing.J0ptionPane;
public class Checkpoint
{
public static void main(String[ ] args)
{
String input;
int number;
input = JOptionPane.showInputDialog(“Enter a number.”);
number = Integer.parseInt(input);
if (number < 10)
method1();
else
method2();
System.exit(0);
}
public static void method1()
{
J0ptionPane.showMessageDialog(nul1, “Able was I.”);
}
public static void method2()
{
J0ptionPane.showMessageDialog(null, “I saw Elba.”);
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Absolute Java (6th Edition)
Starting out with Visual C# (4th Edition)
Programming in C
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Software Engineering (10th Edition)
- Please help me comment these lines of code. Please not these are just some lines of code that have been taken from the java program I am creating which is a coin toss program. I do not need you to create one. Just comment what the lines of code below private static String sideUp; } public void toss() { Random in = new Random(); int x = in.nextInt(); if (x % 2 == 0) sideUp = "Heads"; else sideUp = "Tails"; } public static void main(String[] args) { int tail = 0, head = 0; System.out.println("\nTossing coin 20 times:-"); for (int i = 1; i <= 20; i++) { coin.toss(); if (coin.getFace().equals("tails")) tail++; else head++;arrow_forwardMany user-created passwords are simple and easy to guess. Program that takes a simple password and makes it stronger by replacing characters using the key below, and by appending "q*s" to the end of the input string. i becomes ! a becomes @ m becomes M B becomes 8 o becomes . Ex: If the input is: mypassword the output is: Myp@ssw.rdq*s IN JAVA. USING LOOPSarrow_forwardWrite a java program call CountVowelsDigits which prompts the user for a string, counts the number of vowels(a,e,i,o,u,A,E,I,O,U) and digits(0-9) contain in the string, and prints the counts and percentages rounded to 2 decimal places. Enter a String : testing12345 Number of vowels is: 2 (16.67%) Number of digits is : 5 (41.67%)arrow_forward
- Write a program that prompts a student to enter a Java score. If thescore is greater or equal to 60, display “you pass the exam”; otherwise, display “youdon’t pass the exam”. Your program ends with input -1. Here is a sample run: Enter your score: 80 ↵EnterYou pass the exam.Enter your score: 59 ↵EnterYou don't pass the exam.arrow_forwardWhat will be the output of the following Java code? class average { public static void main(String args[]) { double num[] = {5.5, 10.1, 11, 12.8, 56.9, 2.5}; double result; result = 0; for (int i = 0; i < 6; ++i) result = result + num[i]; System.out.print(result/6); } } a) 16.34 b) 16.566666644 c) 16.46666666666667 d) 16.46666666666666arrow_forwardWrite a complete Java program that displays a message “Welcome to Kean” 5 times using while loop.arrow_forward
- Why is my dialog box not popping up and why is it not working, please help me fix it. and possibly give me an example My Java code is below import javax.swing.JOptionPane;public class HELPME { public static void main(String[] args) { String inputString; //For reading input int numA; int numB; int numC; int numD; double gpa; // Have user input the number of letter grades they earned. inputString = JOptionPane.showInputDialog(null, "Enter the number of A grades received: "); numA =Integer.parseInt(inputString); inputString = JOptionPane.showInputDialog(null, "Enter the number of B grades received: "); numB =Integer.parseInt(inputString); inputString = JOptionPane.showInputDialog(null, "Enter the number of C grades received: "); numC =Integer.parseInt(inputString); inputString = JOptionPane.showInputDialog(null, "Enter the number of D grades received: ");…arrow_forwardPlease help I am receiving error from the java code below. In the images is the assignment I have been given Java code below import java.util.Random; public class Coin{ private static String sideUp; void Coin(){ toss(); } public static void toss(){ Random in = new Random(); int x = in.nextInt(); if(x % 2 == 0) sideUp = "heads"; else sideUp = "tails"; } public static String getFace(){ return sideUp; } public static void main(String[] args) { int tail = 0, head = 0; for(int i = 1; iarrow_forwardWhat will be the output of the following Java program? class Output { public static void main(String args[]) { Double i = new Double(257.5); Double x = i.MAX_VALUE; System.out.print(x); } }arrow_forward
- How do I code a java program that shows the number of grades, calculates the max and min of code, uses -1 as an input end and repeats it self to produce: The output will be: Enter a grade : Number Of Grades = the amount of grades the user inputed Maximum Exam Grade = besed on user input Minimum Exam Grade = besed on user input Enter a grade : Number Of Grades = the amount of grades the user inputed Maximum Exam Grade = besed on user input Minimum Exam Grade = besed on user input Enter a grade : Number Of Grades = the amount of grades the user inputed Maximum Exam Grade = besed on user input Minimum Exam Grade = besed on user input Enter a grade :arrow_forwardJava Question- Convert this source code into a GUI application using JOptionPane. Make sure the output looks similar to the following picture. Thank you. import java.util.Calendar;import java.util.TimeZone;public class lab11_5{public static void main(String[] args){//menuSystem.out.println("-----------------");System.out.println("(A)laska Time");System.out.println("(C)entral Time");System.out.println("(E)astern Time");System.out.println("(H)awaii Time");System.out.println("(M)ountain Time");System.out.println("(P)acific Time");System.out.println("-----------------"); System.out.print("Enter the time zone option [A-P]: "); //inputString tz = System.console().readLine();tz = tz.toUpperCase(); //change to uppercase //get current date and timeCalendar cal = Calendar.getInstance();TimeZone.setDefault(TimeZone.getTimeZone("GMT"));System.out.println("GMT/UTC:\t" + cal.getTime()); switch (tz){case "A":tz =…arrow_forwardWrite an application that asks a user to type an even number or the sentinel value 999 to stop. When the user types an even number, display the message “Good job!” and then ask for another input. When the user types an odd number, display an error message, "x is not an even number", and then ask for another input. When the user types the sentinel value 999, end the program. import java.util.*; public class EvenEntryLoop { public static void main (String args[]) { // Write your code here } }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
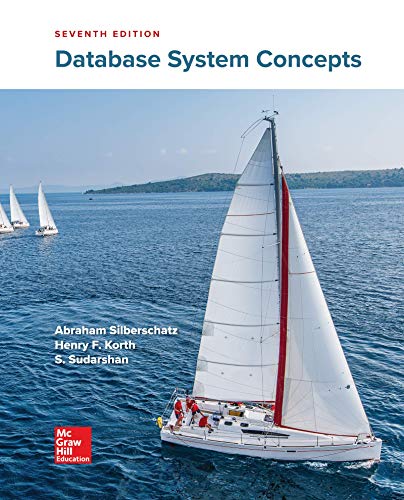
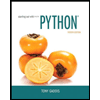
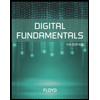
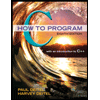
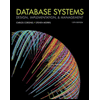
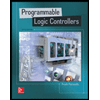