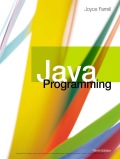
EBK JAVA PROGRAMMING
9th Edition
ISBN: 9781337671385
Author: FARRELL
Publisher: CENGAGE LEARNING - CONSIGNMENT
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 5, Problem 8PE
Program Plan Intro
Automobile application
Program plan:
- In a file “Automobile.java”, create a class “Automobile”,
- Declare the necessary variables.
- Define the constructor,
- Call the method “set_Id()”,
- Call the method “set_Make()”,
- Call the method “set_Model()”,
- Call the method “set_Color()”,
- Call the method “set_Year()”,
- Call the method “set_Mpg()”,
- Define the method “set_Id()”,
- Initialize the final variable.
- Check whether either the id is less than “0” or greater than maximum id,
- If it is true, set the id to “0”.
- Otherwise, set the initial id.
- If it is true, set the id to “0”.
- Define the method “set_Make()” to set the initial make value.
- Define the method “set_Model()” to set the initial model value.
- Define the method “set_Color()” to set the initial color value.
- Define the method “set_Year(),
- Initialize the minimum year and maximum year.
- Check whether either the year is less than the minimum year or greater than the maximum year,
- If it is true, set the year to “0”.
- Otherwise, set the initial year.
- If it is true, set the year to “0”.
- Define the method “set_Mpg()”,
- Initialize the minimum and maximum miles per gallon.
- Check whether either the Miles Per Gallon(MPG) is less than the minimum mpg or greater than the maximum mpg,
- If it is true, set the MPG to “0”.
- Otherwise, set the mpg value.
- If it is true, set the MPG to “0”.
- Define the method “get_Id()” to return the id.
- Define the method “get_Make()” to return the Make.
- Define the method “get_Model()” to return the Model.
- Define the method “get_Color()” to return the Color.
- Define the method “get_Year()” to return the year.
- Define the method “get_Mpg()” to return the MPG.
- In a file “TestAutomobiles.java”, create a class “TestAutomobiles”,
- Define the method “main ()”,
- Call the constructor “Automobile()” that instantiates several job automobile dealers.
- Call the method “display1()” for first automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Call the method “set_Id()” for first automobile dealer.
- Call the method “display1()” for first automobile dealer.
- Call the method “set_Id()” for first automobile dealer.
- Call the method “display1()” for first automobile dealer.
- Call the method “set_Make()” for first automobile dealer.
- Call the method “set_Model()” for first automobile dealer.
- Call the method “display1()” for first automobile dealer.
- Call the method “set_Id()” for second automobile dealer.
- Call the method “set_Color()” for second automobile dealer.
- Call the method “set_Year()” for second automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Call the method “set_Mpg()” for second automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Call the method “set_Mpg()” for second automobile dealer.
- Call the method “display1()” for second automobile dealer.
- Define the method “display1()”,
- Print the output.
- Define the method “main ()”,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
create an automobile class for a dealership. include fields fo an ID number, model, make, color, year and miles per gallon. include get and set methods for each field. do not allow the ID to be negative or more than 9999; if it is, set the ID to 0. do not allow the year to be earlier than 2005 or later than 2019, if it is, set year to 0. do not allow the miles per gallon to be less than 10 or more than 60; if it is, set the miles per gallon to 0. include a constructor that accepts arguments for each field value and uses the set methods to assign the values.
Create an Automobile class for a dealership. Include fields for an ID number, make, model, color, year, and miles per gallon. Include get and set methods for each field. Do not allow the ID to be negative or more than 9999; if it is, set the ID to 0. Do not allow the year to be earlier than 2005 or later than 2019; if it is, set the year to 0. Do not allow the miles per gallon to be less than 10 or more than 60; if it is, set the miles per gallon to 0. Include a constructor that accepts arguments for each field value and uses the set methods to assign the values.
use this code template to help you continue:
private boolean included
Indicates whether the item should be taken or not
public Item(String name, double weight, int value)
Initializes the Item’s fields to the values that are passed in; the included Field is initialized to false
public Item(Item other)
Initializes this item’s fields to the be the same as the other item’s
public void setIncluded(boolean included)
Setter for the item’s included field (you don’t need setters for the other fields)
Given code:
public class Item {
private final String name;
private final double weight;
private final int value;
public Item(String name, double weight, int value) {
this.name = name;
this.weight = weight;
this.value = value;
}
static int max(int a, int b)
{
if(a > b)
return a;
return b;
}
// function to print the items which are taken
static void printSelection(int W, Item[] items, int…
Chapter 5 Solutions
EBK JAVA PROGRAMMING
Ch. 5 - Prob. 1RQCh. 5 - Prob. 2RQCh. 5 - Prob. 3RQCh. 5 - Prob. 4RQCh. 5 - Prob. 5RQCh. 5 - Prob. 6RQCh. 5 - Prob. 7RQCh. 5 - Prob. 8RQCh. 5 - Prob. 9RQCh. 5 - Prob. 10RQ
Ch. 5 - Prob. 11RQCh. 5 - Prob. 12RQCh. 5 - Prob. 13RQCh. 5 - Prob. 14RQCh. 5 - Prob. 16RQCh. 5 - Prob. 17RQCh. 5 - Prob. 18RQCh. 5 - Prob. 19RQCh. 5 - Prob. 20RQCh. 5 - Prob. 1PECh. 5 - Prob. 2PECh. 5 - Prob. 3PECh. 5 - Prob. 4PECh. 5 - Prob. 5PECh. 5 - Prob. 6PECh. 5 - Prob. 7PECh. 5 - Prob. 8PECh. 5 - Prob. 9PECh. 5 - Prob. 10PECh. 5 - Prob. 1GZCh. 5 - Prob. 2GZCh. 5 - Prob. 3GZCh. 5 - Prob. 4GZCh. 5 - Prob. 5GZ
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Using oop in java Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score.arrow_forwardSuper Class: Customer_order Attributes : Customer_name, Customer_Code,Street, City, Zip_code Methods : Read the Customer_order details Display the Customer _order details Sub class: Purchase_details Attributes : Productno, Productname, Price, Quantity Methods : Find the total number of products Find the total price of all products Display the Customer_order details and purchase_details.arrow_forwardDesign a class named CustomerRecord that holds a customer number, name, and address. Include separate methods to 'set' the value for each data field using the parameter being passed. Include separate methods to 'get' the value for each data field, i.e. "return" the field's value. Pseudocode:arrow_forward
- Part A Create an Automobile class for a dealership. Include fields for an ID number (id), make (make), model (model), color (color), year (year), and miles per gallon (mpg). Include get and set methods for each field. Do not allow the ID to be negative or more than 9999; if it is, set the ID to 0. Do not allow the year to be earlier than 2005 or later than 2024; if it is, set the year to 0. Do not allow the miles per gallon to be less than 10 or more than 60; if it is, set the miles per gallon to 0. Include a default constructor that accepts no arguments and an overloaded constructor that accepts arguments for each field value and uses the set methods to assign the values. Part B Write an application called TestAutomobiles that declares two Automobile objects. Write a function called enterData() that prompts the user for the data values for an Automobile object and returns that object. When you test the program, be sure to enter some invalid data to demonstrate that all the methods…arrow_forwardMethods are functions which are declared inside a class. True Falsearrow_forwardApply for higher studies: The system allows the students to search for study programs and apply for higher studies in foreign universities (applying requires attaching transcripts). Administrator can use this application to download reports add more seats into the course. etc etc Create your own case study (problem statement) for the above application by expanding it with more details from your side and for this case study 1. Class diagram with at least 4 classes and explain all the relationships 3. Draw the use case diagramarrow_forward
- solve with java Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score. Create a class “Main” having main method to perform following tasks. Create two objects of Subject class having…arrow_forwardI CANT GET IT RIGHT Create a class named Purchase. Each Purchase contains an invoice number, amount of sale, and amount of sales tax. Include set methods for the invoice number and sale amount. Within the set() method for the sale amount, calculate the sales tax as 5% of the sale amount. Also include a display method that displays a purchase’s details. CODE import java.util.Scanner; class CreatePurchase { publicstaticvoidmain(Stringargs[]) { Purchase purchase=newPurchase(); Scanner s=newScanner(System.in); System.out.println("Enter the details: "); while(true) { System.out.print("Enter invoice number[between 1000 and 8000]: "); int i=s.nextInt(); if(i>=1000&& i<=8000){purchase.setInvoiceNumber(i);break;} else System.out.println("Wrong entry,enter again"); } while(true) { System.out.print("Enter amount of purchase[should be non-negative]: "); int i=s.nextInt(); if(i>=0){purchase.setAmountOfSales(i);break;} else System.out.println("Wrong entry,enter again"); }…arrow_forwardStatic & Not Final Field: Accessed by every object, Changing Non-Static & Final Field: Accessed by object itself, Non-Changing Static & Final: Accessed by every object, Non-Changing Non-Static & Not Final Field: Accessed by object itself, ChangingRead the following situation and decide how the variables should be defined. You have a class named HeartsPlayerA round of Hearts starts with every player having 13 cardsPlayers then choose 3 cards to “trade” with a player (1st you pass left, 2nd you pass right, 3rd you pass across, 4th you keep)Players then strategically play cards in order to have the lowest scoreAt the end of the round, points are cumulatively totaled for each player.If one player’s total is greater than 100, the game ends and the player with the lowest score wins. 1. How should the following data fields be defined (with respect to final and static)?(a) playerPosition (These have values of North, South, East, or West)(b) directionOfPassing(c) totalScore…arrow_forward
- Lab conditions: This lab exercise to be completed by the end of the class. No late submission will be accepted Work as group of two students. Submit Word document file on D2L Make sure your following naming format as listed below: Last name, First Name: Last name, First Name: Questions: 1. Research, discuss Explain the purpose of different personal computer (PC) hardware components. Make sure to address all the aspect of the topic. Partial list of opcodes: 2. Desktop Computer DIY. Suppose you decide to build a desktop by yourself and your budget is around $1000 (without OS). Discuss with your team members and list all the parts and tools you have to purchase with price. List the technical Details and explain what your desktop will be used for, such as listen to music, word document, 3D design, software development, watch movie and so on. 3. Download and run CPU-Z. Paste your screenshots (technical details) below. 4. Challenge Question Consider the hypothetical machine: Instruction…arrow_forwardsolve eith java :- Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score. Create a class “Main” having main method to perform following tasks. Create two objects of Subject class having…arrow_forwardA(n)_ is a special kind of class that never uses any non-virtual methods. Please provide your comment in the spaces provided.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
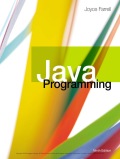
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY