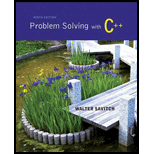
Concept explainers
Write a function that computes the average and standard deviation of four scores. The standard deviation is defined to be the square root of the average of the four values: (Si –a)2, where a is average of the four scores S1, S2, S3, and S4. The function will have six parameters and will call two other functions. Embed the function in a driver

Creation of program to compute average and standard deviation
Program Plan:
- Define the method “clc()” to calculate value of standard deviation.
- The variables are been declared initially.
- The difference of value with average is been computed.
- The square of resultant value is been computed.
- The final value is been returned.
- Define the method “stdMean()” to compute value of standard deviation as well as mean of values.
- The variables are been declared initially.
- The value of average is been calculated by summing values and dividing it by count of elements.
- Compute deviations for each value by calling “clc()” method.
- Compute overall value of deviation and return square root of resultant value.
- Define main method.
- Declare variables values of scores.
- Get each value from user.
- Store values in different variables.
- Call “stdMean()”to compute standard deviation as well as mean of values.
- Display standard deviation and mean of values.
Program Description:
The following C++ program describes about creation of program to compute mean as well as standard deviation of values entered by user.
Explanation of Solution
Program:
//Include libraries
#include <iostream>
#include <math.h>
//Define function prototypes
double stdMean(double,double,double,double,int, double&);
double clc(double, double);
//Use namespace
using namespace std;
//Define main method
int main()
{
//Declare variables
double s1,s2,s3,s4,avg,sd;
//Get value from user
cout<<"Enter 1st value ";
//Store value
cin>>s1;
//Get value from user
cout<<"Enter 2nd value ";
//Store value
cin>>s2;
//Get value from user
cout<<"Enter 3rd value ";
//Store value
cin>>s3;
//Get value from user
cout<<"Enter 4th value ";
//Store value
cin>>s4;
//Call method
sd=stdMean(s1,s2,s3,s4,4,avg);
//Display message
cout<<"The average is "<<avg<<"\n";
//Display message
cout<<"The standard deviation is "<<sd<<"\n";
//Pause console window
system("pause");
//Return
return 0;
}
//Define method clc()
double clc(double x,double avg)
{
//Declare variable
double tmp;
//Compute value
tmp=x-avg;
//Return value
return tmp*tmp;
}
//Define method stdMean()
double stdMean(double lX1, double lX2,double lX3, double lX4,
int n, double &avg)
{
//Declare variables
double d1,d2,d3,d4,dev;
//Compute value
avg=(lX1+lX2+lX3+lX4)/n;
//Call method
d1=clc(lX1,avg);
//Call method
d2=clc(lX2,avg);
//Call method
d3=clc(lX3,avg);
//Call method
d4=clc(lX4,avg);
//Compute value
dev=(d1+d2+d3+d4)/n;
//Return value
return sqrt(dev);
}
Enter 1st value 8
Enter 2nd value 3
Enter 3rd value 5
Enter 4th value 4
The average is 5
The standard deviation is 1.87083
Press any key to continue . . .
Want to see more full solutions like this?
Chapter 5 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Starting Out with Programming Logic and Design (4th Edition)
Starting Out with Python (4th Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
Starting Out With Visual Basic (7th Edition)
- Modify the program so that it does the following: Performs a function that gets the area of a rectangle. The function must receive two parameters (decimal numbers) that represent the base and height of the rectangle and must return the calculated value (decimal number). Perform a second function that obtains the total area of a rectangular prism with a rectangular base. The total area of such a prism is equal to the sum of the areas of each of its faces. It uses calls to the previous function for this calculation. Call this last function in the main with user data. Execution example Give me the base: 21.3 Give me the height: 10 Give me the depth: 2.0 The total area of the prism is: 551.2arrow_forwardDesign a function called intdiv() which takes a dividend and a divisor as parameters and returns the result and remainder (2 integers) as a tuple. E.G. given 9/2 equals 4 remainder 1:9 is the dividend, 2 is the divisor (parameters), 4 is the result and 1 is the remainder (values returned as a tuple).Write program statements which test this function by calling it twice with different parameters, and printing the returned values each time.arrow_forwardWrite a program that calculates the average of a group of test scores. You will ask the user to input each test score individually. When calculating the average, the lowest score in the group is dropped. Your program should use the following functions: void getScore() should ask the user for a test score, store it in a reference parameter variable, and validate it. This function should be called by main once for each of the five scores to be entered. void calcAverage() should calculate and display the average of the four highest scores. This function should be called just once by main and should be passed the five scores. int findLowest) should find and return the lowest of the five scores passed to it. It should be called by calcAverage(), which uses the function to determine which of the five scores to drop. Void displayAverage() should display the average of the four highest scores. This function should be called by calcAverage(). Input Validation: Do not accept test scores lower…arrow_forward
- Write a function called numberCompare. This function takes in two parameters (both numbers). If the first parameter is greater than the second, this function returns "First is Greater". If the second parameter is greater than the first, this function returns "Second is Greater" Else the function returns "Numbers are Equal". You will need to add an if statement in your functionarrow_forwardIt is estimated that a restaurant's tip box accumulates twice as much as the previous day. Write the function with the main () function that takes the number of days as a parameter and returns the total tip amount on the day entered in the restaurant with a 10-TL tip on the first day. Sample Work: Enter the number of days: 4 --------------------------------- Total estimated stake in the box: 150.00 -TL in carrow_forwardChange this question to work using a function. decide what the name of the function of each should be, how many parameters are required and what value needs to be returned. You're no longer required to solve the problem - try to re-manage your code to be a function. Write a program to prompt the user for hours and rate per hour to compute gross pay. You will have to use formatted printing here as well to only display two decimal points. Enter Hours: 35 Enter Rate: 2.75 Pay: $ 96.25arrow_forward
- Write a program that calculates the average of a group of test scores, where the lowest score in the group is dropped. It should use the following function: getScore This function ask the user for a test score, store it in a reference parameter variable, and validate it. For input validation, do not accept test scores lower than 0 or higher than 100. This function should be called by main() once for each of the five scores to be entered. calcAverage This function calculates and display the average of the four highest score. This function should be called just once by main(), by should be passed the five scores. • findLowest This function finds and returns the lowest of the five scores passed to it. It should be called by calcAverage function, which uses the function to determine which of the five scores to drop.arrow_forwardIf a year has the digit 7 OR the digit 4 in it, then it is called a “Beautiful year”. For example, 2017, 2014, and 2072 are all beautiful years. If the year has BOTH 4 and 7 in it, then it's an “Amazing year”. For example, the years 2047 and 2074 are amazing years. All other years are “Ugly years”. You need to write a function that takes a year as an argument and return it's type. Then, finally print the returned values in the function call. ================================================ Sample Function Call 1: function_name(2470) Sample Output 1: Amazing year ================================================ Sample Function Call 2: function_name(2170) Sample Output 2: Beautiful year ================================================ Sample Function Call 3: function_name(2021) Sample Output 3: Ugly yeararrow_forwardLewis' invention can determine if the weather is too hot (104°F and above) or too cold (50°F and below) once the unit of measure of the temperature is in Fahrenheit. Which is why, he added another function that converts the temperature from Celsius to Fahrenheit to make his invention work. Create a program that contains the function: HotOrCold(). HotOrCold() function will accept as parameter a temperature type and the temperature and will do the following: Convert the given temperature in Celsius to Fahrenheit. a. [F = C + 32] a. Display if depending on the temperature value. Weather is too hot" or "Weather is too cold" , OF THE MADE4Learners NSTITUTE FRAMEWORK VERSITY UNI MULTIPLE APPROACHES TO DISTANCE EDUCATION FOR TECHNOLOGIAN LEARNERS : O Google Slides CHNOLOGarrow_forward
- Change this question to work using a function. decide what the name of the function of each should be, how many parameters are required and what value needs to be returned. You're no longer required to solve the problem - try to re-manage your code to be a function. write a program to prompt to user for hours and rate per hour to compute gross pay. You’ll have to use formatted printing here as well to only display two decimal points. Enter Hours: 35 Enter Rate: 2.75 Pay: $96.25 rate = float (input ("How much do you get paid an hour?")) hours = float(input ("How many hours have you worked?")) print ("Pay: $%.2f"%(rate*hours))arrow_forwardWrite a function in python code for checking the speed of drivers. This function should have one parameter: speed. If speed is less than 70, it should print “Ok”. Otherwise, for every 5km above the speed limit (70), it should give the driver one demerit point and print the total number of demerit points. For example, if the speed is 80, it should print: “Points: 2”. If the driver gets more than 12 points, the function should print: “License suspended”.arrow_forwardThe following formula can be used to determine the distance an object falls due to gravity in a specific time period: d = 1⁄2 gt2The variables in the formula are as follows: d is the distance in meters, g is 9.8, and t is the time in seconds that the object has been falling. Write a function named fallingDistance that accepts an object’s falling time (in seconds) as an argument. The function should return the distance, in meters, that the object has fallen during that time interval. Write a program that demonstrates the function by calling it in a loop that passes the values 1 through 10 as arguments and displays the return value.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
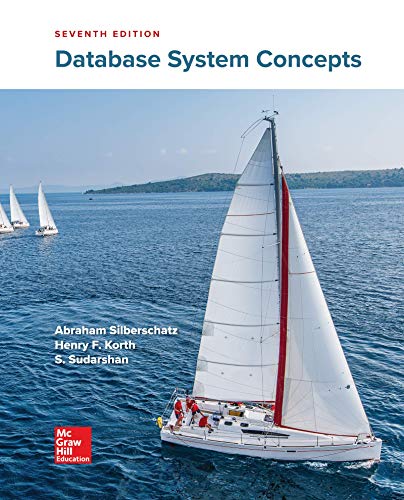
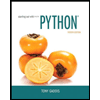
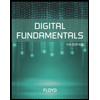
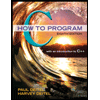
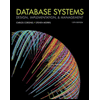
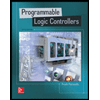