What is meant by function?
A function is a process or task that does some operation in the program. Some programming languages may contain built-in functions for certain commonly used tasks. Most programming languages also support user-defined functions, which means a user can write their own function to perform some operation. There are some libraries or header files that contain built-in functions for performing certain tasks.
Types of functions
- Predefined function or Built-in function: It contains functions that performs well-known tasks and they are distributed as libraries.
- User-defined function: Created by a developer for their own use.
Built-in function
Predefined functions are created during the development of the programming language. Header files contain these functions. Given below are the examples of built-in functions in C programming language:
- printf() is a default function, which is used to display the output on the console. It is present in the stdio.h library file.
- sqrt() is a function, which calculates the square root value of a particular numeric value. It takes an integer argument and finds its square root. This function is available in the math.h library file.
User-defined function
User-defined functions are developed by a programmer for reducing the steps in the program. Once defined it can be called any number of times. It improves readability and maintainability of code. The general of user-defined function is shown below.
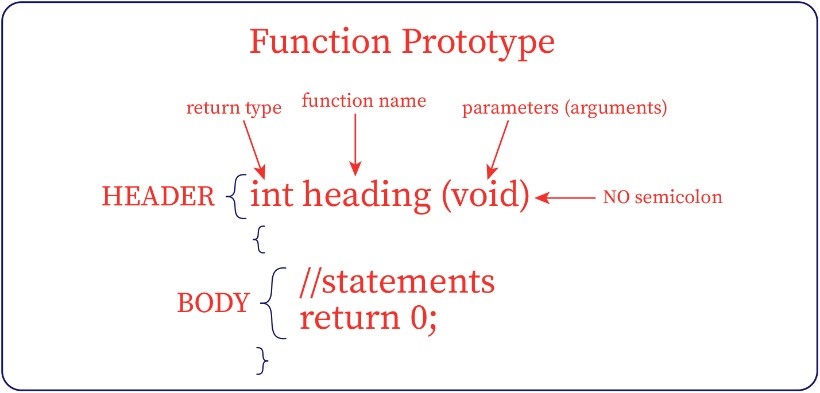
Syntax
datatype name_of_function()
{
// body of function
return type of function
}
User-defined functions consists of the following:
- Data type of function.
- Name of function.
- Parameters passed to the function (optional).
- Body of function.
- Return type of function.
Function parameter types
Function parameters can be passed in the following ways. They are,
- Pass by value.
- Pass by reference.
Pass by value
In pass-by value, the actual value will be passed directly to the function. The values of actual parameters are copied to formal parameters. The modifications done to the formal parameters are not reflected in the calling function.
Code
#include <stdio.h>
void swapfun(int , int); // function declaration
int main()
{
int aa = 13; //variable declaraion
int bb = 23;
printf("At main function n Before swapping n aa = %d, n bb = %dn",aa,bb); // print aa and bb value
swapfun(aa,bb); //function call
printf("At main function n After swapping n aa = %d, n bb = %dn",aa,bb);
}
void swapfun (int aa, int bb) //function definition
{
int tem;
tem = aa;
aa=bb;
bb=tem;
printf("At swap functionn After swapping n aa = %d, n bb = %dn",aa,bb);
}
Output
At main function
Before swapping
aa =13,
bb =23
At swapfun function
Before swapping
aa =23,
bb =13
At main function
Before swapping
aa =13,
bb =23
Explanation
The above code swaps the values of aa and bb. In the main function, the variables are initialized and print the values of aa and bb before swapping. The function call swapfun() swaps the values and the print statement displays the values of aa and bb after swapping. In call by value, the print statement after function call will print the same values, as the changes made to the formal parameters within the function is not reflected in the calling function.
Pass value by reference
In pass value by reference, the address of the actual parameter is passed which is assigned to the formal parameter. This is achieved by using pointer variables. Therefore, changes made inside the function will be reflected in the calling function. The implementation is given below:
Code
#include<stdio.h>
int func(int *x) //function definition
{
(*x) +=100; //Adding 100 to the pointer
printf(“ The value of x is: %d”,*x); //printing the pointer
return 0; //returns false value to avoiding errors
}
main() //main function
{
int x = 10; //declaring and initializing the variable
func(&x); //passing the value by reference
}
Output
The value of x is: 110
Explanation
The changes made to the variable x in the called function is reflected in the calling function since both refer to the same memory location.
Advantages
- Reduces the repetition of code in the program.
- Improves readability by reducing number of lines of code.
- Complex logic can be easily implemented by dividing it into simpler modules.
- Predefined functions for common tasks reduces development effort.
Disadvantage
- It results in processing overhead in terms of function call and return.
Context and Applications
This topic is important for postgraduate and undergraduate courses, particularly for, Bachelors in Computer Science Engineering and Associate of Science in Computer Science.
Practice Problems
Question 1: A program in C/C++ must contain atleast one ___________
A) function
B) argument
C) parameter
D) none of the above
Answer: Option A is correct.
Explanation: Program execution begins from main() function. Therefore, a program must contain atleast one function.
Question 2: Which of the following syntax is correct for function declaration?
A) var abc(x);
B) int abc(int x);
C) char 12();
D) None of the above
Answer: Option B is correct
Explanation: Function declaration must contain return type, function name and comma-separated list of arguments enclosed within parenthesis.
Question 3: Select the advantage of the function.
A) Complex to implement
B) Not processed easily
C) Easy to implement
D) None of the above
Answer: Option C is correct.
Explanation: The function implementation is always an important part of programming. It reduces the lines of code in the program and makes the code understandable.
Question 4: Does math.h is defined as a function?
A) Yes
B) Maybe yes
C) No
D) Maybe no
Answer: Option is C is correct.
Explanation: Math.h is a header file used in programming which contains different mathematical functions and it has different functions for various operations.
Question 5: Which of the given function is used to find the square root of integer value?
A) squareroot()
B) square_root()
C) sqrt()
D) None of the above
Answer: Option C is correct.
Explanation: sqrt() is a predefined function available in math.h header file, which is used to find the square root of an integer value.
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Types of Function Homework Questions from Fellow Students
Browse our recently answered Types of Function homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.