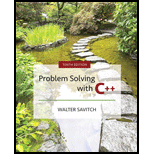
Concept explainers
The following function is supposed to take as arguments a length expressed in feet and inches and return the total number of inches in that many feet and inches. For example, total_inches(1,2) is supposed to return 14, because 1 foot and 2 inches is the same as 14 inches. Will the following function perform correctly? If not, why not?
double total_inches(int feet, int inches)
{
inches = 12 * feet + inches;
return inches;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Introduction To Programming Using Visual Basic (11th Edition)
Modern Database Management
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- Suppose that the two Rank methods below are added to the Skip List class on Blackboard. public int Rank(T item) Returns the rank of the given item. public T Rank(int i) Returns the item with the given rank i. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an expected time complexity of O(log n) for each of the Rank methods. Show as well that the methods Insert and Remove can efficiently maintain this data as items are inserted and removed. (7 marks) 2. Re-implement the methods Insert and Remove of the Skip List class to maintain the augmented data in expected O(log n) time. Using the Contains method, ensure that added items are distinct. (6 marks) 3. Implement the two Rank methods. (8 marks) 4. Test your new methods thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forwardWhy do we need official standards for copper cable and fiber-optic cable? What happens without the standard?arrow_forwardWhat is the difference between physical connection (Physical topology) and logical connection (Logical topology)? Why are both necessary?arrow_forward
- There are two network models and name them while providing a couple of advantages and disadvantages for each network model.arrow_forwardhttps://docs.google.com/document/d/1lk0DgaWfVezagyjAEskyPoe9Ciw3J2XUH_HQfnWSmwU/edit?usp=sharing use the link to answer the question below b) As part of your listed data elements, define the following metadata for each: Data/FieldType, Field Size, and any possible constraint/s or needed c) Identify and describe the relationship/s among the tables. Please provide an example toillustrate Referential Integrity and explain why it is essential for data credibility. I have inserted the data elements below for referencearrow_forwardWhy do we use NAT and PAT technologies? What happens without them? What is the major difference between NAT and PAT (Port Address Translation)? please answer it in the simplest way as possiblearrow_forward
- What is the difference between physical connection (Physical topology) and logical connection (Logical topology)? Why are both necessary? Why do we need the Seven-Layer OSI model? What will happen If we don’t have it? Why do we need official standards for copper cable and fiber-optic cable? What happens without the standard? please answer in the simplest way as possiblearrow_forwardSuppose that the MinGap method below is added to the Treap class on Blackboard. public int MinGap( ) Returns the absolute difference between the two closest numbers in the treap. For example, if the numbers are {2, 5, 7, 11, 12, 15, 20} then MinGap would returns 1, the absolute difference between 11 and 12. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an time complexity of O(1) for the MinGap method. Show as well that the methods Add and Remove can efficiently maintain this data as items are added and removed. (6 marks) 2. Re-implement the methods Add and Remove of the Treap class to maintain the augmented data in expected O(log n) time. (6 marks) 3. Implement the MinGap method. (4 marks) 4. Test your new method thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forwardSuppose that the two Rank methods below are added to the Skip List class on Blackboard. public int Rank(T item) Returns the rank of the given item. public T Rank(int i) Returns the item with the given rank i. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an expected time complexity of O(log n) for each of the Rank methods. Show as well that the methods Insert and Remove can efficiently maintain this data as items are inserted and removed. (7 marks) 2. Re-implement the methods Insert and Remove of the Skip List class to maintain the augmented data in expected O(log n) time. Using the Contains method, ensure that added items are distinct. (6 marks) 3. Implement the two Rank methods. (8 marks) 4. Test your new methods thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forward
- https://docs.google.com/document/d/1lk0DgaWfVezagyjAEskyPoe9Ciw3J2XUH_HQfnWSmwU/edit?usp=sharing use the link to answer the question below b) As part of your listed data elements, define the following metadata for each: Data/FieldType, Field Size, and any possible constraint/s or needed c) Identify and describe the relationship/s among the tables. Please provide an example toillustrate Referential Integrity and explain why it is essential for data credibility. I have inserted the data elements below for referencearrow_forwardHighlight the main differences between Computer Assisted Coding and Alone Coding with their similaritiesarrow_forwardSuppose that the MinGap method below is added to the Treap class on Blackboard. public int MinGap ( ) Returns the absolute difference between the two closest numbers in the treap. For example, if the numbers are {2, 5, 7, 11, 12, 15, 20} then MinGap would returns 1, the absolute difference between 11 and 12. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an time complexity of O(1) for the MinGap method. Show as well that the methods Add and Remove can efficiently maintain this data as items are added and removed. (6 marks) 2. Re-implement the methods Add and Remove of the Treap class to maintain the augmented data in expected O(log n) time. (6 marks) 3. Implement the MinGap method. (4 marks) 4. Test your new method thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- COMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
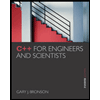
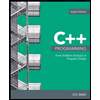
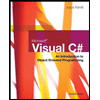