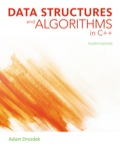
EBK DATA STRUCTURES AND ALGORITHMS IN C
4th Edition
ISBN: 9781285415017
Author: DROZDEK
Publisher: YUZU
expand_more
expand_more
format_list_bulleted
Question
Chapter 4, Problem 7E
Program Plan Intro
Stack:
Stack is a container in which elements inserted and removed in LIFO (Last In First Out) manner.
- The “top” is address of top element of stack.
- Basic stack operations are given below:
- push(): Insert an object into stack top.
- pop(): Delete object in stack top.
- topEl(): Get object in stack top.
- isEmpty(): Check stack is empty or not.
Queue:
- Queue is another data structure in which insertion and removal of elements are in FIFO(First In First Out) manner.
- Basic operations are given below:
- Enqueue(): Insert an element into back of queue
- Dequeue(): Remove an item from front of queue
- front(): Get first element of queue without removal of it.
- isEmpty(): Check queue is empty or not.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
#include <iostream>
usingnamespace std;
class Queue {
int size;
int* queue;
public:
Queue(){
size = 0;
queue = new int[100];
}
void add(int data){
queue[size]= data;
size++;
}
void remove(){
if(size ==0){
cout <<"Queue is empty"<<endl;
return;
}
else{
for(int i =0; i < size -1; i++){
queue[i]= queue[i +1];
}
size--;
}
}
void print(){
if(size ==0){
cout <<"Queue is empty"<<endl;
return;
}
for(int i =0; i < size; i++){
cout<<queue[i]<<" <- ";
}
cout << endl;
}
//your code goes here
};
int main(){
Queue q1;
q1.add(42); q1.add(2); q1.add(8); q1.add(1);
Queue q2;
q2.add(3); q2.add(66); q2.add(128); q2.add(5);
Queue q3 = q1+q2;
q3.print();…
class Queue { private static int front, rear, capacity; private static int queue[]; Queue(int size) { front = rear = 0; capacity = size; queue = new int[capacity]; } // insert an element into the queue static void queueEnqueue(int item) { // check if the queue is full if (capacity == rear) { System.out.printf("\nQueue is full\n"); return; } // insert element at the rear else { queue[rear] = item; rear++; } return; } //remove an element from the queue static void queueDequeue() { // check if queue is empty if (front == rear) { System.out.printf("\nQueue is empty\n"); return; } // shift elements to the right by one place uptil rear else {…
1 Implement a Queue Data Structure specifically to store integer data using a Singly Linked List.
2 The data members should be private.
3
You need to implement the following public functions:
4
1. Constructor:
5 It initialises the data members as required.
6
7
8
2. enqueue(data) :
This function should take one argument of type integer. It enqueues the element into the queue and returns nothing.
3. dequeue():
It dequeues/removes the element from the front of the queue and in turn, returns the element being dequeued or removed. In case the queue is empty, it r
4. front ():
10
11 It returns the element being kept at the front of the queue. In case the queue is empty, it returns -1.
12 5. getSize():
13
It returns the size of the queue at any given instance of time.
14 6. 1sEmpty():
15
It returns a boolean value indicating whether the queue is empty or not.
16 Operations Performed on the Stack:
17 Query-1 (Denoted by an integer 1): Enqueues an integer data to the queue.
18
19
Query-2…
Chapter 4 Solutions
EBK DATA STRUCTURES AND ALGORITHMS IN C
Knowledge Booster
Similar questions
- #include using namespace std; class Queue { int size; int* queue; public: Queue() { size = 0; queue = new int[100]; } void add(int data) { queue[size] = data; size++; } void remove() { if (size == 0) { cout << "Queue is empty"<arrow_forward#include <iostream>using namespace std; class Queue { int *queue, size, front, rear;public: Queue(int size) { queue = new int[size]; this -> size = size; front = rear = -1; } ~Queue() { delete[] queue; } void push(int val) { if (rear == -1) { queue[++rear] = val; front++; } else { if (rear == ((front - 1) % size)) throw "Queue Full!"; if (rear == size - 1) rear = -1; queue[++rear] = val; } } int pop() { if (front == -1) throw "Queue Empty!"; int ret = queue[front]; front = (front + 1) % size;…arrow_forwardconst int MAX = 10000; class enum errorCode (underflow, overflow, success); class Stack ( public: Stack () ({ count = 0; } errorCode top(int &) const; errorCode pop (); errorCode push (int) const; private: int count; on int entry[MAX]; Write the following functions that do not belong to the class: (a) Function int size(Stack &s) leaves the Stack s unchanged and returns a count of the number of entries in the Stack, (b) Function void deleteAll(Stack &s, stackEntry x) deletes all occurrences (if any) of x from s and leaves the remaining entries in s in the same relative order.arrow_forward
- Problem Description: 1. Suppose that we want to add a method to a class of queues that will splice two queues together. This method adds to the end of a queue all items that are in a second queue. The header of the method could be as follows: public void splice (QueueInterface anotherQueue) Write this method in such a way that it will work in any class that implements QueueInterface.arrow_forwardclass Queue { private static int front, rear, capacity; private static int queue[]; Queue(int c) { front = rear = 0; capacity = c; queue = new int[capacity]; } static void queueEnqueue(int data) { if (capacity == rear) { System.out.printf("\nQueue is full\n"); return; } else { queue[rear] = data; rear++; } return; } static void queueDequeue() { if (front == rear) { System.out.printf("\nQueue is empty\n"); return; } else { for (int i = 0; i < rear - 1; i++) { queue[i] = queue[i + 1]; } if (rear < capacity) queue[rear] = 0; rear--; } return; } static void queueDisplay() { int i; if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } for (i = front; i < rear; i++) { System.out.printf(" %d <-- ", queue[i]); } return; } static void queueFront() { if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } System.out.printf("\nFront Element is: %d", queue[front]);…arrow_forwardStack: Stacks are a type of container with LIFO (Last In First Out) type of working, where a new element is added at one end and (top) an element is removed from that end only. Your Stack should not be of the fixed sized. It should be able to grow itself. So using the class made in task 1, make a class named as Stack, having following additional functionalities: bool empty() : Returns whether the Stack is empty or not. Time Complexity should be: O(1) bool full() : Returns whether the Stack is full or not. Time Complexity should be: O(1)int size() : Returns the current size of the Stack. Time Complexity should be: O(1)Type top () : Returns the last element of the Stack. Time Complexity should be: O(1) void push(Type) : Adds the element of type Type at the top of the stack. Time Complexity should be: O(1) Type pop() : Deletes the top most element of the stack and returns it. Time Complexity should be: O(1) Write non-parameterized constructor for the above class. Write Copy…arrow_forward
- Write a C++ program to perform the Queue operation. Note:Use the following class template for Queue creation. template <class T>class Queue{ private: int front,rear; T *queue; int maxsize;}; Define the following function in the class Queue class Method name Description int isFull() The method is used to check whether the queue is full or not. void insert(T) The method is used to display the rear element in the queue (if the queue is stored with the element(s)). void deletion() The method is used to delete the front element from the queue. void atFront() The method is used to display the front element in the queue (if the queue is stored with the element(s)). void atRear() The method is used to add data to the rear end of the queue. void display() The method is used to display all the data in the queue. int isEmpty() The method is used to check whether the queue is empty or not. Input and Output Format:The first line of input…arrow_forwardFor the following problems, you need to submit a python code that performs the required functions. Q1: (Stack & Queue) 1. Write a program that utilizes the LinkedStack class and LinkedQueue class to perform the following function. Input :empty stack and a Queue Required: reverse the order of the queue. // you must use the methods of the stack and queue class // Q2) Write a member functions to the class ( CircularQueue ) that performs the followings: 1. Sum-even(CQ) : Print the sum of all even numbers stored in CircularQueue. 2. Perform the followings: a. Create two circular linked lists ( CQ1, CQ2). b. Merge the two circular linked lists in one →CQ1. //Remember: you need to use the class ( CircularQueue ) methods.//arrow_forwardMultiple choice in data structures void stack::do(){ for(int i=0li<=topindex/2;i++){ T temp=entry[i]; entry[i]=entry[topindex-i-1]; entry[topindex-1-i]=temp;} } What is this method do? a. swap the first item with last item b. replace each item with next item value c. doesn't do any thing d. reverse the stackarrow_forward
- Assume the Queue is initially empty. class Queue { public: int size () ; bool empty (); chará front () throw (Exception); void enqueue (char& e); void dequeue () throw (Exception); Function call Output Queue Contents (back -> front) enqueue ('A') enqueue ('B') front ( ) size ( ) dequeue () enqueue ('C') empty( ) dequeue ( ) size( ) dequeue () empty ( ) front ( ) enqueue ('D') front () dequeue () dequeue ( )arrow_forwardStack:#ifndef STACKTYPE_H_INCLUDED#define STACKTYPE_H_INCLUDEDconst int MAX_ITEMS = 5;class FullStack// Exception class thrown// by Push when stack is full.{};class EmptyStack// Exception class thrown// by Pop and Top when stack is emtpy.{};template <class ItemType>class StackType{public:StackType();bool IsFull();bool IsEmpty();void Push(ItemType);void Pop();ItemType Top();private:int top;ItemType items[MAX_ITEMS];};#endif // STACKTYPE_H_INCLUDED Queue:#ifndef QUETYPE_H_INCLUDED#define QUETYPE_H_INCLUDEDtemplate<class T>class QueType{public:QueType();QueType(int);~QueType();void MakeEmpty();bool IsEmpty();bool IsFull();void Enqueue(T);void Dequeue(T&);T Front();private:int front;int rear;T *info;int maxQue;};#endif // QUETYPE_H_INCLUDEDarrow_forwardc++ Write a client function that returns the back of a queue while leaving the queue unchanged. This function can call any of the methods of the ADT queue. It can also create new queues. The return type is ITemType, and it accepts a queue as a parameter.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
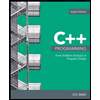
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning