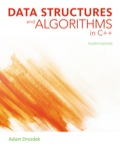
EBK DATA STRUCTURES AND ALGORITHMS IN C
4th Edition
ISBN: 9781285415017
Author: DROZDEK
Publisher: YUZU
expand_more
expand_more
format_list_bulleted
Question
Chapter 4, Problem 5PA
Program Plan Intro
a. Program to find sum of large numbers by adding each number to resultant:
Program Plan:
- In function “addingLargeNumbers()”:
- It takes two stacks corresponding to two large numbers as parameters.
- Till any one of stack becomes empty,
- Pop each digit from both stacks and add them with carry.
- Push resultant digit into resultant stack and store carry digit in “carryy”.
- If first stack is not empty, till stack becomes empty,
- Pop each digit from stack and add them with carry.
- Push resultant digit into resultant stack and store carry digit in “carryy”.
- If second stack is not empty, till stack becomes empty,
- Pop each digit from stack and add them with carry.
- Push resultant digit into resultant stack and store carry digit in “carryy”.
- If carry is not “0”, push it into resultant stack.
- Return resultant stack.
- In function “main()”:
- Read number of large numbers to find sum and first two numbers from user.
- Compute sum of first two numbers by calling function “addingLargeNumbers()”.
- Read each number from user and add it with resultant number by calling function “addingLargeNumbers()”.
- After adding all numbers. Pop each digit from resultant stack and push into other stack to get digits of result in correct order.
- Print result.
Program Plan Intro
b. Program to find sum of larger numbers by using
Program Plan:
- In function “addingLargeNumbers()”:
- It takes a vector of stacks and number of elements in vector as parameters.
- Compute maximum number of digits “maxsz” a number having in vector.
- For each digit in first position to “maxsz” position
- Add each digit of all stacks together and pop each digit from stack.
- Replace old stack with new stack.
- Add result with carry. Push resultant digit into resultant stack and store carry digit in “carryy”.
- After adding all digits of all numbers, if carry is not “0”, push it into resultant stack.
- Return resultant stack.
- In function “main()”:
- Read number of large numbers to find sum.
- Read all numbers from user and store them in a vector.
- Call function “addingLargeNumbers()” by passing vector and vector size as parameters. It will return resultant stack.
- Print resultant stack.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
It's a very nice application of stacks. Consider that a freight train has n railroad cars. Each to be left at different station. They're numbered 1 through n & freight train visits these stations in order n through 1. Obviously, the railroad cars are labeled by their destination.
To facilitate removal of the cars from the train, we must rearrange them in ascending order of their number (i.e. 1 through n). When cars are in this order, they can be detached at each station. We rearrange cars at a shunting yard that has input track, output track & k holding tracks between input & output tracks (i.e. holding track).
The figure shows a shunting yard with three holding tracks H1, H2 & H3, also n = 9. The n cars of freight train begin in the input track & are to end up in the output track in order 1 through n from right to left. The cars initially are in the order 5,8,1,7,4,2,9,6,3 from back to front. Later cars are rearranged in desired order.
To rearrange cars, we…
Use stacks from the STL to implement a video game library functionality. In this implementation, consider a VideoGame class with title, release year, genre and publisher as variables. Provide setters and getters to these variables.
In main, create a stack of video games. Add 5 different game objects into the stack. Then in a loop, for each item that you retrieve from the stack display the game information as follows:
TITLEYEARGENREPUBLISHER
It is known that a matrix can be understood (in python) by a list of lists. In this context, make a program that receives 9 numbers, organize them in a matrix 3 x 3 and print this matrix and its transpose.
In Phyton3
Chapter 4 Solutions
EBK DATA STRUCTURES AND ALGORITHMS IN C
Knowledge Booster
Similar questions
- Given a singly linked list, reverse the list. This means you have to reverse every node. For example if there are 4 nodes, the node at position 0 will move to position 3, the node at 1 will move to position 2 and so on. You do not have to write the Node class just write what you have been asked to. You are NOT allowed to create a new list. In python languagearrow_forwardImplement a simple stack in java using the scenario below. A company wishes to keep track of the order in which it employed staff. Users can enter either "f" to fire, "h" to hire or "e" to end the program. When a member of staff is employed, their employee number is added to the top of a stack when someone has to be made redundant, the last person to join the company is removed from the top of the stack. Hint: Use ArrayListarrow_forwardGiven a stack of positive integers, write a Java method called movePrimes that rearranges elements of the stack such that all prime numbers are below none primes regardless to the order of primes. Note: You are allowed to create only one other stack. You can not use other data structures such as array, arraylist, queue, ....etc. Consider the following example:arrow_forward
- Apply the methods in the ListIterator interface to write a Java program in NetBeans that creates a LinkedList of four elements of type string, namely: Java, C#, PHP and Python. The program should then print out the elements initially in the original order, and then afterwards in reverse order using the ListIterator methods. In this case, the following is the expected output:arrow_forward1. Suppose you have the following array: {11, 22, 33, 44, 55, 66, 77}. Write a java program to find the sum of elements of the array recursively. 2. Suppose a program builds and manipulates a linked list: What two special nodes would the program typically keep track of? Describe two common uses for the null reference in the node of the linked lists. 3. Consider the following Java Code: What is the output when this code is executed? import java.util.LinkedList; import java.util.ListIterator; class Main { public static void main(String[] args) { LinkedList<String> myLList = new LinkedList<String>(); myLList.addFirst("Ali"); myLList.addFirst("Omar"); myLList.addLast("Sara"); ListIterator<String> myiterator = myLList.listIterator(); myiterator.next(); myiterator.next(); myiterator.add("Reem"); myiterator.previous(); myiterator.add("Ahmad"); myiterator.add("Khaled"); myiterator.previous(); myiterator.remove(); System.out.println(myLList); myiterator.next();…arrow_forwardWrite a program SentenceReverser that reverses the words in a sentence by reading words into a Stack until you find a period. Your program should then pop off the words from the stack. Begin your reverse sentence with a capital letter and end it with a period. Your program should be able to handle multiple sentences. Use while (scan.hasNext()) to capture your input. Mary had a little lamb. His fleece was as white as snow. Becomes: Lamb little a had mary. Snow as white as was fleece his. Note: Your test input should include multiple sentences as shown above. There should NOT be a space before your periods.arrow_forward
- Apply the methods in the ListIterator interface to write a Java program in NetBeans that creates a LinkedList of four elements of type string, namely: Java, C#, PHP and Python.The program should then print out the elements initially in the original order, and then afterwards in reverse order using the ListIterator methods. In this case, the following is the expected output: Run: original order of strings: Java C# PHP Python Reverse order of strings: Python PHP C#Java BUILD SUCCESSFULarrow_forwardWrite a java method to search for an element in an array using a linear search. Many list processing tasks, including searching, can be done recursively. The base case typically involves doing something with a limited number of elements in the list (say the first element), then the recursive step involves doing the task on the rest of the list. Think about how linear search can be viewed recursively; if you are looking for an item in a list starting at index i:o ¬If i exceeds the last index in the list, the item is not found (return -1).o ¬If the item is at list[i], return i.o ¬If the is not at list[i], do a linear search starting at index i+1arrow_forwardWrite a program in java that randomly fills in 0s and 1s into an n-by-n matrix, prints the matrix, and finds the rows and columns with the most 1s. (Hint: Use two ArrayLists to store the row and column indices with the most 1s.)arrow_forward
- In Java: Modify the attached program code below According to the question a, b and c a. Replace the appendNode() method by an insertNode() method which inserts the new node in such a way to keep the list always sorted in increasing order. b. Add a recursive method displayReverse() which displays the list in reverse order. c. Do the needed changes to the main()in order to reflect the above two changes. public class DoublyLinkedList { private Node head;private Node tail;private int size; DoublyLinkedList() {tail = head = null;size = 0;} public void addNode(String item) {//adding a node at the endNode newNode = new Node(item);if(head == null) {head = tail = newNode;}else {newNode.prev = tail;tail.next = newNode;tail = newNode;}size++;} public boolean remove(String item) {Node current = head;boolean found = false;while((current != null)&&(!found)) {if(current.element.equals(item))found = true;elsecurrent = current.next;}if(found){if(current == head) head =…arrow_forwardWe have to create a program in java in which the below mentioned things should be there- You have been given the head to a singly linked list of integers. Write a function check to whetherthe list given is a 'Palindrome' or not.Input Format:Elements of linked listOutput Format:True or FalseSample Input 1:9 3 2 2 3 9Sample Output 1:trueSample Input 2:0 2 3 2 5Sample Output 2:falsearrow_forwardApply the methods in the ListIterator interface to write a Java program in NetBeans that creates a LinkedList of four elements of type string, namely: Java, C#, PHP and Python.The program should then print out the elements initially in the original order, and then afterwards in reverse order using the ListIterator methods. In this case, the following(attached) is the expected output:arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
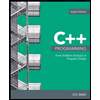
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning