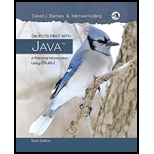
Concept explainers
Implement the findProduct method. This should look through the collection for a product whose id field matches the ID argument of this method. If a matching product is found, it should be returned as the method's result. If no matching product is found, return null.
This differs from the printProductDetai1s method, in that it will not necessarily have to examine every product in the collection before a match is found. For instance, if the first product in the collection matches the product ID, iteration can finish and that first Product object can be returned. On the other hand, it is possible that there might be no match in the collection. In that case, the whole collection will be examined without finding a product to return. In this case, the null value should be returned.
When looking for a match, you will need to call the get ID method on a Product.

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Additional Engineering Textbook Solutions
Starting Out with Python (4th Edition)
Electric Circuits. (11th Edition)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
SURVEY OF OPERATING SYSTEMS
Starting Out With Visual Basic (8th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
- Can you implement the Student class using the concepts of encapsulation? A solution is placed in the "solution" section to help you, but we would suggest you try to solve it on your own first. You are given a Student class in the editor. Your task is to add two fields: ● String name ● String rollNumber and provide getter/setters for these fields: ● getName ● setName ● getRollNumber ● setRollNumber Implement this class according to the rules of encapsulation. Input # Checking all fields and getters/setters Output # Expecting perfectly defined fields and getter/setters. There is no need to add constructors in this class.arrow_forwardPlease help me create a cave class for a Hunt the Wumpus game. You can read the rules in it's entirety of the Hunt the Wumpus game online to get a better idea of the specifications. It's an actual game. INFORMATION: The object of this game is to find and kill the Wumpus within a minimum number of moves, before you get exhausted or run out of arrows. There is only one way to win: you must discover which room the Wumpus is hiding in and kill it by shooting an arrow into that room from an adjacent room. The Cave The Wumpus lives in a cave of 30 rooms. The rooms are hexagonal. Each room has up to 3 tunnels, allowing access to 1, 2 or 3 (out of 6) adjacent rooms. The attached diagram shows the layout of rooms in the cave. The map wraps around such that rooms on the edges (white cells) have neighbors from the opposite edge (blue cells). E.g., the neighbors of room 1 are rooms 25, 26, 2, 7, 6, and 30, and you could choose to connect room 1 to any of these rooms. Observe how room 1…arrow_forwardFor the first part of this lab, copy your working ArrayStringList code into the GenericArrayList class.(already in the code) Then, modify the class so that it can store any type someone asks for, instead of only Strings. You shouldn't have to change any of the actual logic in your class to accomplish this, only type declarations (i.e. the types of parameters, return types, etc.) Note: In doing so, you may end up needing to write something like this (where T is a generic type): T[] newData = new T[capacity]; ...and you will find this causes a compiler error. This is because Java dislikes creating new objects of a generic type. In order to get around this error, you can write the line like this instead: T[] new Data = (T[]) new Object[capacity] This creates an array of regular Objects which are then cast to the generic type. It works and it doesn't anger the Java compiler. How amazing! Once you're done, screenshot or save your code for checkin later. For the second part of the lab,…arrow_forward
- The code given below represents a saveTransaction() method which is used to save data to a database from the Java program. Given the classes in the image as well as an image of the screen which will call the function, modify the given code so that it loops through the items again, this time as it loops through you are to insert the data into the salesdetails table, note that the SalesNumber from the AUTO-INCREMENT field from above is to be inserted here with each record being placed into the salesdetails table. Finally, as you loop through the items the product table must be update because as products are sold the onhand field in the products table must be updated. When multiple tables are to be updated with related data, you should wrap it into a DMBS transaction. The schema for the database is also depicted. public class PosDAO { private Connection connection; public PosDAO(Connection connection) { this.connection = connection; } public void…arrow_forwardbetterAddProduct Write a method in your Warehouse class to further optimize addProduct. As of now, it’s possible that an item is removed from a full sector to make room for a new product, even if there are other sectors which are not full. If the current sector is not full, add the product as normal. Otherwise perform a linear probing-like operation to try to find a non-full sector (if the current one is full). Keep incrementing the sector until you either find one with space, or you return to your original sector. If you get to Sector 9, wrap around to Sector 0. If you found a new sector with space, add the product into this sector. In a real-world scenario we would have to make sure to change the ID in our system and make sure it doesn’t conflict with an existing one. In this assignment that is not necessary since the output only contains the name. If you returned to the original sector, perform eviction and add the product as normal.arrow_forwardYour task: Remove any word that does not start with a capital letter. For instance, for the input text of "Hello, 2022 world!", we should get "Hello, 2022 !".arrow_forward
- Please help me create a cave class for a Hunt the Wumpus game (in java). You can read the rules in it's entirety of the Hunt the Wumpus game online to get a better idea of the specifications. It's an actual game. INFORMATION: The object of this game is to find and kill the Wumpus within a minimum number of moves, before you get exhausted or run out of arrows. There is only one way to win: you must discover which room the Wumpus is hiding in and kill it by shooting an arrow into that room from an adjacent room. The Cave The Wumpus lives in a cave of 30 rooms. The rooms are hexagonal. Each room has up to 3 tunnels, allowing access to 1, 2 or 3 (out of 6) adjacent rooms. The attached diagram shows the layout of rooms in the cave. The map wraps around such that rooms on the edges (white cells) have neighbors from the opposite edge (blue cells). E.g., the neighbors of room 1 are rooms 25, 26, 2, 7, 6, and 30, and you could choose to connect room 1 to any of these rooms. Observe how…arrow_forwardWrite a method called changeQueue to be considered inside the ArrayQueue class and has one parameter item of type E. Your method will change the queue in such a way that if the the first element of the queue and the last element of the queue are equal, the first and last elements in the queue will be replaced with item, otherwise, do not do any change. Similarly, the same process is done for the second element in the queue and the element before the last in the queue and so on. Assume you have a non-empty queue with even number of elements. You are not allowed to call any method from the the ArrayQueue. class. Do not use iterators. Method head: public void changeQueue(E item) Examplet: Before run: front rear "this" queue: 10 29 2 25 10 Item1: 100 After run: front rear "this" queue: 100 29 100 100 5 100 Use the editor to format your answerarrow_forward(Count entire words, not parts of words. For example rate and rated would be different counts. Say rate is there 10 times and rated is there 5 times, that should be the count not rate 15, rated 5. Don't count parts of words.) A Map is an interface that maps keys to values. The keys are unique and thus, no duplicate keys are allowed. A map can provide three views, which allow the contents of the map to be viewed as a set of keys, collection of values, or set of key-value mappings. In addition, the order of the map is defined as the order in which, the elements of a map are returned during iteration. The Map interface is implemented by different Java classes, including HashMap, HashTable, and TreeMap. Each class provides different functionality and can be either synchronized or not. Also, some implementations prohibit null keys and values, and some have restrictions on the types of their keys. A map has the form Map <k,v> where: K: specifies the type of keys maintained in…arrow_forward
- Please answer the question in the screenshot. The language used is Java.arrow_forwardThe map file holds a bunch of rectangles. Some of the rectangles are solid, and you can think of them like crates in a warehouse. They can be enclosed by a non-solid rectangle, and those rectangles are just there to make theintersection testing and such go faster. If a line doesn't cross a non-solid rectangle, then it cannot hit any rectangle inside it. Nice! The format is:Solid(1)/Nonsolid(0) x_min y_min x_max y_max (list of children iff not solid) The list of children are line numbers (the lines start at 0) of solid rectangles inside the non-solid rectangle. Solid rectangles do not have children. All non-solid rectangles must have children. Here's a sample file:0 0 0 100 100 1 21 1.1 1.0 1.2 1.31 10 10 11 110 50 60 70 80 40 55 65 65 75 51 56 66 64 74 The first line (line 0) is a non-solid rectangle with two children (the next two rows, lines 1 and 2). The first rectangle ranges from (0,0) to (100,100). The children of it are both small solid rectangles, the first is only .1 x .3,…arrow_forwardWrite the value and compareTo methods for a pair of cards where suits play an important role. Aces are high, and assume that suits are ranked clubs (low), diamonds, hearts, and spades (high). Assume that face values are only considered if the suits are the same; otherwise ranking of cards depends on their suits alone.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
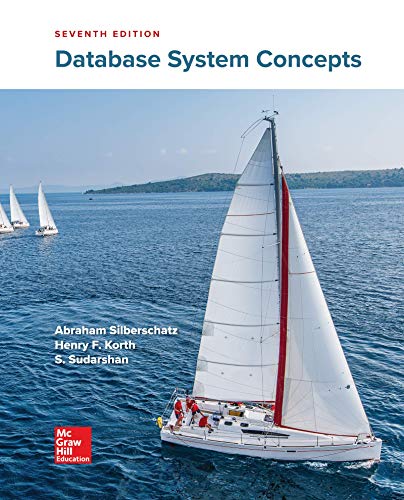
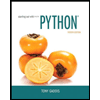
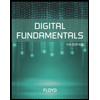
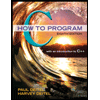
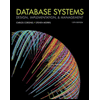
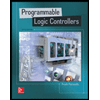