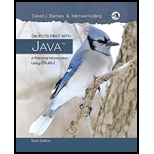
Concept explainers
Add a getUnsold method to the Auction class with the following header:
This method should iterate over the lots field, storing unsold lots in a new ArrayList local variable. What you are looking for is Lot objects whose highestBid field Is null. At the end of the method, return the list of unsold lots.

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects (9th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
SURVEY OF OPERATING SYSTEMS
Starting Out with Python (4th Edition)
Starting Out With Visual Basic (8th Edition)
Concepts Of Programming Languages
- This lab was designed to reinforce programming concepts from this lab, you will practice: Declaring and initializing ArrayList. Add elements to the Arraylist. Display the content of the ArrayList. Write a class name PhoneBookEntry that has fields for a person’s name and phone number. The class should have a constructor and the appropriate accessor and mutator methods. Then write a program that creates at least 5 PhoneBookEntry objects and stores them in an ArrayList. Use a loop to display the contents of each object in the ArrayList.arrow_forwardGiven a base Plant class and a derived Flower class, complete main() to create an ArrayList called myGarden. The ArrayList should be able to store objects that belong to the Plant class or the Flower class. Create a method called printArrayList(), that uses the printInfo() methods defined in the respective classes and prints each element in myGarden. The program should read plants or flowers from input (ending with -1), add each Plant or Flower to the myGarden ArrayList, and output each element in myGarden using the printInfo() method. Ex. If the input is: plant Spirea 10 flower Hydrangea 30 false lilac flower Rose 6 false white plant Mint 4 -1 the output is: Plant 1 Information: Plant name: Spirea Cost: 10 Plant 2 Information: Plant name: Hydrangea Cost: 30 Annual: false Color of flowers: lilac Plant 3 Information: Plant name: Rose Cost: 6 Annual: false Color of flowers: white Plant 4 Information: Plant name: Mint Cost: 4 import java.util.Scanner;import java.util.ArrayList;import…arrow_forwardpublic class Product { private String name; private double cost; public Product(String n, double c) { name=n; cost=c; } public String getName() { return name; On the left is code for a class called Product. Fill in the blanks in the code on the right in order to create three appropriate Product instances and add them to the cart ArrayList. Use the output of the code, shown below the code, to guide you. } public double getCost() { return cost; } public String toString() { return (name + "$" + cost); } public static void addProducts (ArrayList c) { add(new Product("Shampoo", 13.89)); add(new Product("Bread",4.99)); add(new Product("Cereal", 7.49)); } public static void main(String[] args) { ArrayList cart = new ArrayList(); ddProducts (cart); for (Product p : cart) { System.out.println(p); } } Output: Shampoo $13.89 Bread $4.99 Cereal $7.49arrow_forward
- Part #2: Write a class called Test equallists having main method to test the application. In main method create following: 1- Object of type ArrayList list1. 2- Add the following data to list1 (4 13 3- Object of type ArrayList list2. 4- Add the following data to list2 (4 2 1 12 5- Object of type ArrayList list3. 6- Test the equallists method to print the result. Example: list1: 4 13 1 list2: 4 2 1 list3: 1 0 8 12 1 9 0 9 0 2 0 OOL 1 0 (generated by the method) 0 150 10 18 19 01). 9205).arrow_forward1. ArrayListsCreate a class called OurArrayList and implement the following methods. Do NOT create any auxiliary memory.Include a main method and test all your methods with appropriate examples.Assume the following classes have been defined:a. Write a method called scaleByK() that takes an ArrayList of integers as a parameter and replaces every integer of value K with K copies of itself. For example, if the list stores the values (4, 1 , 2, 0 ,3) before the method is called, it should store the values (4, 4, 4, 4, 1, 2, 2, 3, 3, 3) after the method finishes executing. Zeroes and negative numbers should be removed from the list by this method.b. Write a method markLength4() that takes an ArrayList of Strings as a parameter and that places a String of four asterisks ("****") in front of every String of length 4. For example, suppose that an ArrayList called "list" contains the following values:(this, is, lots, of, fun, for, every, Java, programmer)And you make the following…arrow_forwardWrite the definitions of the member functions of the classes arrayListType and unorderedArrayListType that are not given in this chapter. The specific methods that need to be implemented are listed below. Implement the following methods in arrayListType.h: isEmpty isFull listSize maxListSize clearList Copy constructor Implement the following method in unorderedArrayListType.h insertAt Also, write a program (in main.cpp) to test your function.arrow_forward
- Arrays: create an array of a given type and populate its values. Use of for loop to traverse through an array to do the following : to print the elements one by one, to search the array for a given value. ArrayList: create an ArrayList containing elements of a given type . Use some of the common ArrayList methods to manipulate contents of the ArrayList. Write methods that will take an ArrayList as its parameter/argument ; and/or return an ArrayList reference variable as its return type. Searching for an object in an Array: Loop through the ArrayList to extract each object and to check if this object’s attribute has a given value. Explain how and why interfaces are used in Java Collection Framework. Explain the major differences between a Stack and a Queue. Be able to use stack and queue methods. What is meant by O(N) notation? Express the complexity of a given code using the O(N) notation.arrow_forwardTask 4. Utility classes Implement a Menu-Driven program of ArrayList class using the above scenario and perform the following operations. Create an object of ArrayList class based on the scenario with explanations of the method Create a method that will allow inserting an element to ArrayList Class based on the scenario. The input field must be validated as Create a method to delete specific elements in the ArrayList with explanations of the methodarrow_forwardJava (ArrayList) - Plant Informationarrow_forward
- Write code that creates an ArrayList that can hold String objects. Add the names of three cars to the ArrayList, and then display the contents of the ArrayList.arrow_forwardThis program is going to maintain a list of customers’ names and bank account balances. 1. Create a class called CustomerList with a main method that instantiates an ArrayList of String objects called customerName. Invoke the add method to add the following names into the ArrayList: Cathy Ben Jorge Wanda Freddie 1. Print each name in the ArrayList using a for a loop. 2. Create another ArrayList object called customerBalance, to save users’ balance. What is the type of this ArrayList? double or Double? You just need to declare this ArrayList and leave it empty for now. 3. For each customer, print the name and ask the user to enter the initial balance for this customer. Save the initial balance into ArrayList customerBalance. Use a loop to get this done for all 5 customers. The program should print out this for the first customer: Example: Please enter the account balance for Cathy: (waiting for user’s input …) Code: for(int i=0; i<5;i++) { Print(“Please enter the account balance…arrow_forwardTask 1:The first task is to make the maze. A class is created called Maze, in which a 2D array for the maze is declared. Your job is to implement the constructor according to the given javaDoc. When you completed this task, write a set of Junit test case to test your code.As you probably noticed, the maze is defined as a private variable. The second job for you is to write an accessor(getter) method that returns the maze. Other information about what is expected for this method is given in the starter code. public class PE1 { MazedogMaze; /** * This method sets up the maze using the given input argument * @param maze is a maze that is used to construct the dogMaze */ publicvoidsetup(String[][]maze){ /* insert your code here to create the dogMaze * using the input argument. */ }arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
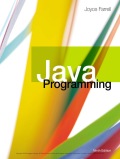