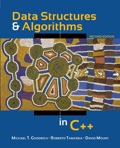
Explanation of Solution
Program code:
//include the required header files
#include <iostream>
#include<time.h>
//use the std namespace
using namespace std;
//Declare n as type of constant
const int n = 10;
//Implementation of prefixAverages1 array
double *prefixAverages1(int X[])
{
//Declare a as integer type
int a;
//Decalre A as double pointer type array
double *A = new double[n];
//Iterate the loop
for (int i = 0; i <= n - 1; i++)
{
//assign 0 to a
a = 0;
//Iterate the loop
for (int j = 0; j <= i; j++)
{
//calculate a = a+X[j] value
a = a + X[j];
}
//assign a / (i + 1)
A[i] = a / (i + 1);
}
//return value of the array A
return A;
}
//Implementation of prefixAverages1 array
double *prefixAverages2(int X[])
{
//Declare s and assign 0
int s = 0;
//Decalre A as double pointer type array
double *A = new double[n];
//Iterate the loop
for (int i = 0; i <= n - 1; i++)
{
//calculate s = s+X[i] value
s = s + X[i];
//assign s / (i + 1)
A[i] = s / (i + 1);
}
//return A
return A;
}
int main()
{
//Decalre X as type of an array
int X[n];
//Declare differenceValue1, differenceValue2 as type of double
double differenceValue1, differenceValue2;
//Declare timeInseconds1, timeInseconds2 as type of double
double timeInseconds1, timeInseconds2;
//Declare i as type of integer
int i = 0;
//Iterate the loop
while (i <= n)
{
//assign i to X[i]
X[i] = i;
//increment i
i++;
}
//Declare resultimeEntrie1,resultimeEntrie2 as type of double
double *resultimeEntrie1 =0, *resultimeEntrie2 = 0;
// declare clock_t objects as timeEntrie1,timeEntrie2
clock_t timeEntrie1, timeEntrie2;
timeEntrie1 = clock();
//store the return value of prefixAverages1(x)
resultimeEntrie1 = prefixAverages1(X);
//Declare k as integer type and assign 0
int k = 0;
// initialize timeEntrie2
timeEntrie2 = clock();
// get the differenceValueerence of two object values
differenceValue1 = (double)timeEntrie2 - (double)timeEntrie1;
// calculate timeInseconds
timeInseconds1 = differenceValue1 / CLOCKS_PER_SEC;
// display statement for timeInseconds
cout << " prefixAverages1(X) " << endl;
//Display statement
cout<<"For n = " << n << " elements then it take " << timeInseconds1 << " seconds" << endl;
// declare clock_t objects as timeEntrie3,timeEntrie4
clock_t timeEntrie3, timeEntrie4;
timeEntrie3 = clock();
//store the return value of prefixAverages2(x)
resultimeEntrie2 = prefixAverages2(X);
timeEntrie4 = clock();
// calculate the differenceValue of two object values
differenceValue2 = (double)timeEntrie4 - (double)timeEntrie3;
// calculate timeInseconds
timeInseconds2 = differenceValue2 / CLOCKS_PER_SEC;
// display statement for timeInseconds
cout << " prefixAverages2(X)" << endl;
//Display statement
cout<<"\n For n = " << n << " elements then it take " << timeInseconds2 << " seconds" << endl;
return 0;
}
Explanation:
The above program snippet is used to implement “prefixAverages1()” and “prefixAverages2()”. In the code,
- Include the required header files.
- Use the “std” namespace.
- Declare the constant “n”.
- Define the method “prefixAverages1()”.
- Declare the required variables.
- Declare an array “A”.
- Iterate a “for” loop.
- Calculate the value of “a”.
- Iterate a “for” loop.
- Calculate the value of “a”.
- Assign a value “a/(i+1)”.
- Return the value of “A”...

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Data structures and algorithms in C++
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
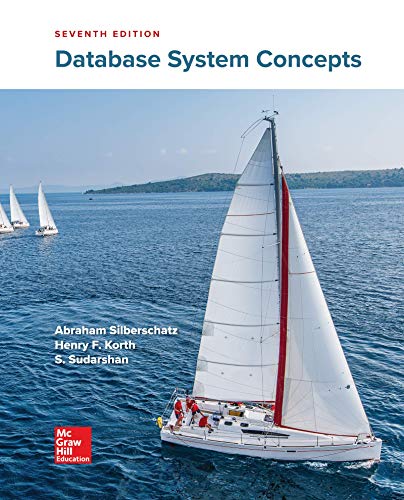
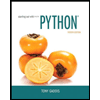
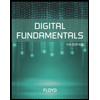
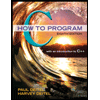
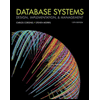
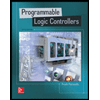