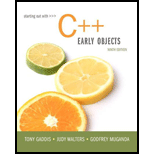
Assume the following variable definitions:
int a = 5, b = 12;
double x = 3.4, z = 9.1;
What are the values of the following expressions?
A) b / a
B) x * a
C) static_cast<double>(b / a)
D) static_cast<double>(b) / a
E) b / static_cast<double>(a)
F) static_cast<double>(b) / static_cast<double>(a)
G) b / static_cast<int>(x)
H) static_cast<int>(x) * static_cast<int>(z)
I) static_cast<int>(x * z)
J) static_cast<double>(static_cast<int>(x) * static_cast<int>(z))

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Starting Out with C++: Early Objects (9th Edition)
Additional Engineering Textbook Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Web Development and Design Foundations with HTML5 (8th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
SURVEY OF OPERATING SYSTEMS
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
- ut da Q4: Consider the LIBRARY relational database schema shown in Figure below a. Edit Author_name to new variable name where previous surname was 'Al - Wazny'. Update book_Authors set Author_name = 'alsaadi' where Author_name = 'Al - Wazny' b. Change data type of Phone to string instead of numbers. عرفنه شنو الحل BOOK Book id Title Publisher_name BOOK AUTHORS Book id Author_name PUBLISHER Name Address Phone e u al b are rage c. Add two Publishers Company existed in UK. insert into publisher(name, address, phone) value('ali','uk',78547889), ('karrar', 'uk', 78547889) d. Remove all books author when author name contains second character 'D' and ending by character 'i'. es inf rmar nce 1 tic عرفته شنو الحل e. Add one book as variables data? عرفته شنو الحلarrow_forwardAdd a timer in the following code. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private Timer timer; private long elapsedTime; public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) { this.labyrinth = labyrinth; this.player = player; this.dragon = dragon; String playerName = JOptionPane.showInputDialog("Enter your name:"); player.setName(playerName); elapsedTime = 0; timer = new Timer(1000, e -> { elapsedTime++; repaint(); }); timer.start(); } @Override protected void paintComponent(Graphics g) { super.paintComponent(g); int cellSize = Math.min(getWidth() / labyrinth.getSize(), getHeight() / labyrinth.getSize());}arrow_forwardChange the following code so that when player wins the game, the game continues by creating new GameGUI with the same player. However the player's starting position is same, everything else should be reseted. public static void main(String[] args) { Labyrinth labyrinth = new Labyrinth(10); Player player = new Player(9, 0); Random rand = new Random(); Dragon dragon = new Dragon(rand.nextInt(10), 9); JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon); frame.setLayout(new BorderLayout()); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(gui, BorderLayout.CENTER); frame.pack(); frame.setResizable(false); frame.setVisible(true); } public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private Timer timer; private long…arrow_forward
- Create a menu item which restarts the game. Also add a timer, which counts the elapsed time since the start of the game level. When the restart is pressed, the restarted game should ask the player' name (in the GameGUI constructor) and set the score of player to 0 (player.setScore(0)), and the timer should restart again. And create a logic so that if the player loses his life (checkGame if the condition is false), then save this number together with his name into two variables. And display two buttons where one quits the game altogether (System.exit(0)) and the other restarts the game. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private final ImageIcon playerIcon = new ImageIcon("data/images/player.png"); private final ImageIcon dragonIcon = new ImageIcon("data/images/dragon.png"); private final ImageIcon wallIcon = new ImageIcon("data/images/wall.png"); private final ImageIcon…arrow_forwardPlease original work Analyze the complexity issues of processing big data What are five complexities and talk about the reasons they make the implementation complex. Please cite in text references and add weblinksarrow_forwardCreate a Database in JAVA Netbeans that saves name of the player and how many labyrinths did the player solve. Record the number of how many labyrinths did the player solve, and if he loses his life, then save this number together with his name into the database. Create a menu item, which displays a highscore table of the players for the 10 best scores public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private void checkGameState() { if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) { JOptionPane.showMessageDialog(this, "You escaped! Congratulations!"); System.exit(0); } if (Math.abs(player.getX() - dragon.getX()) <= 1 && Math.abs(player.getY() - dragon.getY()) <= 1) { JOptionPane.showMessageDialog(this, "The dragon caught you! Game Over."); System.exit(0); } } } public…arrow_forward
- Create a Database in JAVA OOP that saves name of the player and how many labyrinths did the player solve. Record the number of how many labyrinths did the player solve, and if he loses his life, then save this number together with his name into the database. Create a menu item, which displays a highscore table of the players for the 10 best scores. Also, create a menu item which restarts the game. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private void checkGameState() { if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) { JOptionPane.showMessageDialog(this, "You escaped! Congratulations!"); System.exit(0); } if (Math.abs(player.getX() - dragon.getX()) <= 1 && Math.abs(player.getY() - dragon.getY()) <= 1) { JOptionPane.showMessageDialog(this, "The dragon caught you! Game Over.");…arrow_forwardChange the following code so that the player can see only the neighboring fields at a distance of 3 units. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private final ImageIcon playerIcon = new ImageIcon("data/images/player.png"); private final ImageIcon dragonIcon = new ImageIcon("data/images/dragon.png"); private final ImageIcon wallIcon = new ImageIcon("data/images/wall.png"); private final ImageIcon emptyIcon = new ImageIcon("data/images/empty.png"); public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) { this.labyrinth = labyrinth; this.player = player; this.dragon = dragon; setFocusable(true); addKeyListener(new KeyAdapter() { @Override public void keyPressed(KeyEvent e) { char move = switch (e.getKeyCode()) { case KeyEvent.VK_W -> 'W'; case…arrow_forwardQ/ Fill in the table below with the correct answers for the network devices and components required, then draw the topology diagram for the network of this three-story building: I want a drawing Cisco Packet tracer Ground Floor: This floor will house the main server room, reception area, and a few conference rooms. The server room should contain the core network devices that will connect the entire building. The reception area and conference rooms need network access require access for mobile devices for visitors and guests with devices. First Floor: This floor is dedicated to offices with workstations, each needing reliable network connectivity for staff computers, IP phones, IP camera, and printers. Second Floor (Education Department): This floor consists of educational spaces requiring controlled internet access. Only the educational website (https://uokerbala.edu.iq) should be accessible, with all other sites restricted.. Device Media type Location floor Type of IP Static/dynamic…arrow_forward
- Problem Statement You are working as a Devops Administrator. Y ou’ve been t asked to deploy a multi - tier application on Kubernetes Cluster. The application is a NodeJS application available on Docker Hub with the following name: d evopsedu/emp loyee This Node JS application works with a mongo database. MongoDB image is available on D ockerHub with the following name: m ongo You are required to deploy this application on Kubernetes: • NodeJS is available on port 8888 in the container and will be reaching out to por t 27017 for mongo database connection • MongoDB will be accepting connections on port 27017 You must deploy this application using the CL I . Once your application is up and running, ensure you can add an employee from the NodeJS application and verify by going to Get Employee page and retrieving your input. Hint: Name the Mongo DB Service and deployment, specifically as “mongo”.arrow_forwardI need help in server client project. It is around 1200 lines of code in both . I want to meet with the expert online because it is complicated. I want the server send a menu to the client and the client enters his choice and keep on this until the client chooses to exit . the problem is not in the connection itself as far as I know.I tried while loops but did not work. please help its emergentarrow_forwardI need help in my server client in C languagearrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
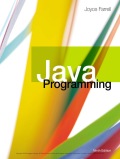
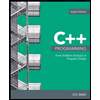